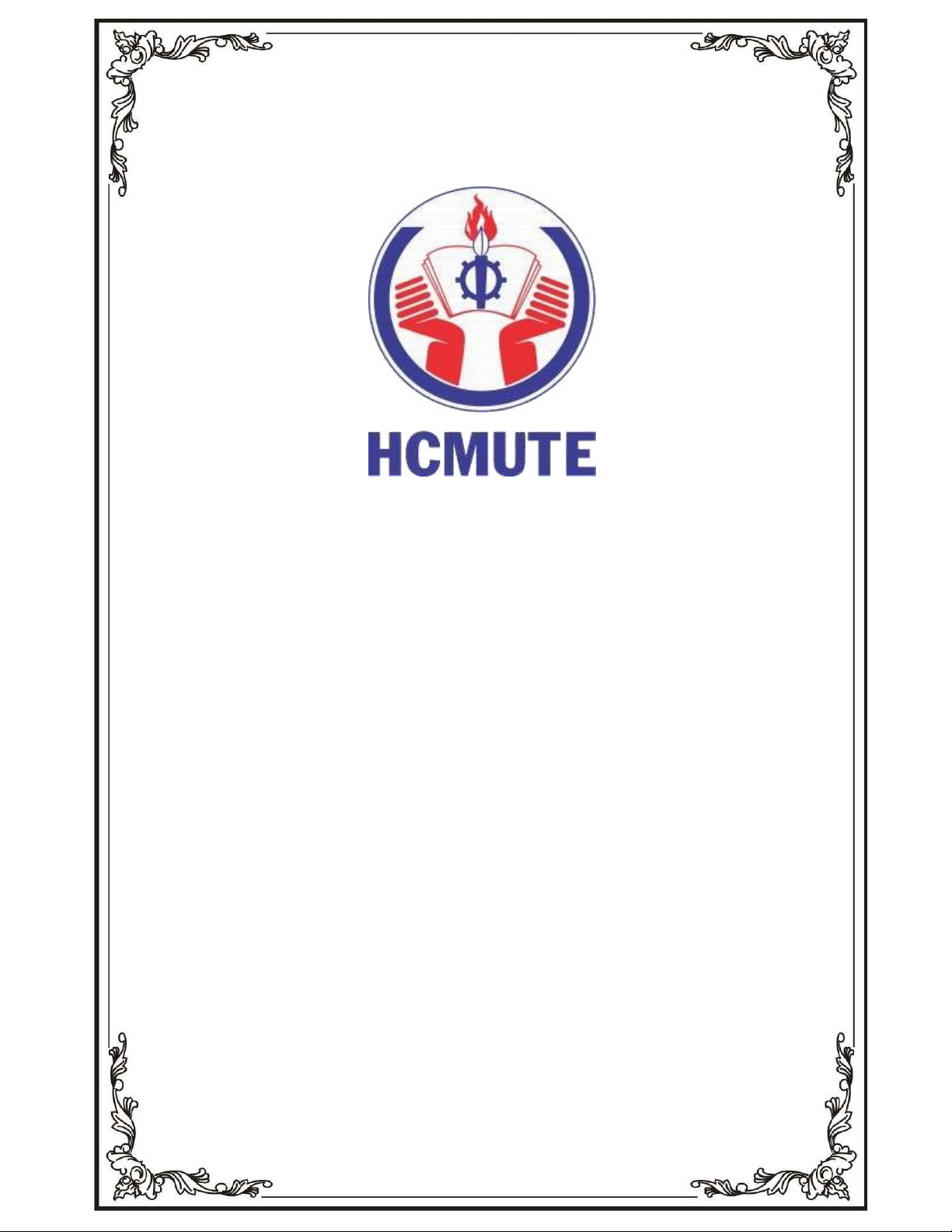
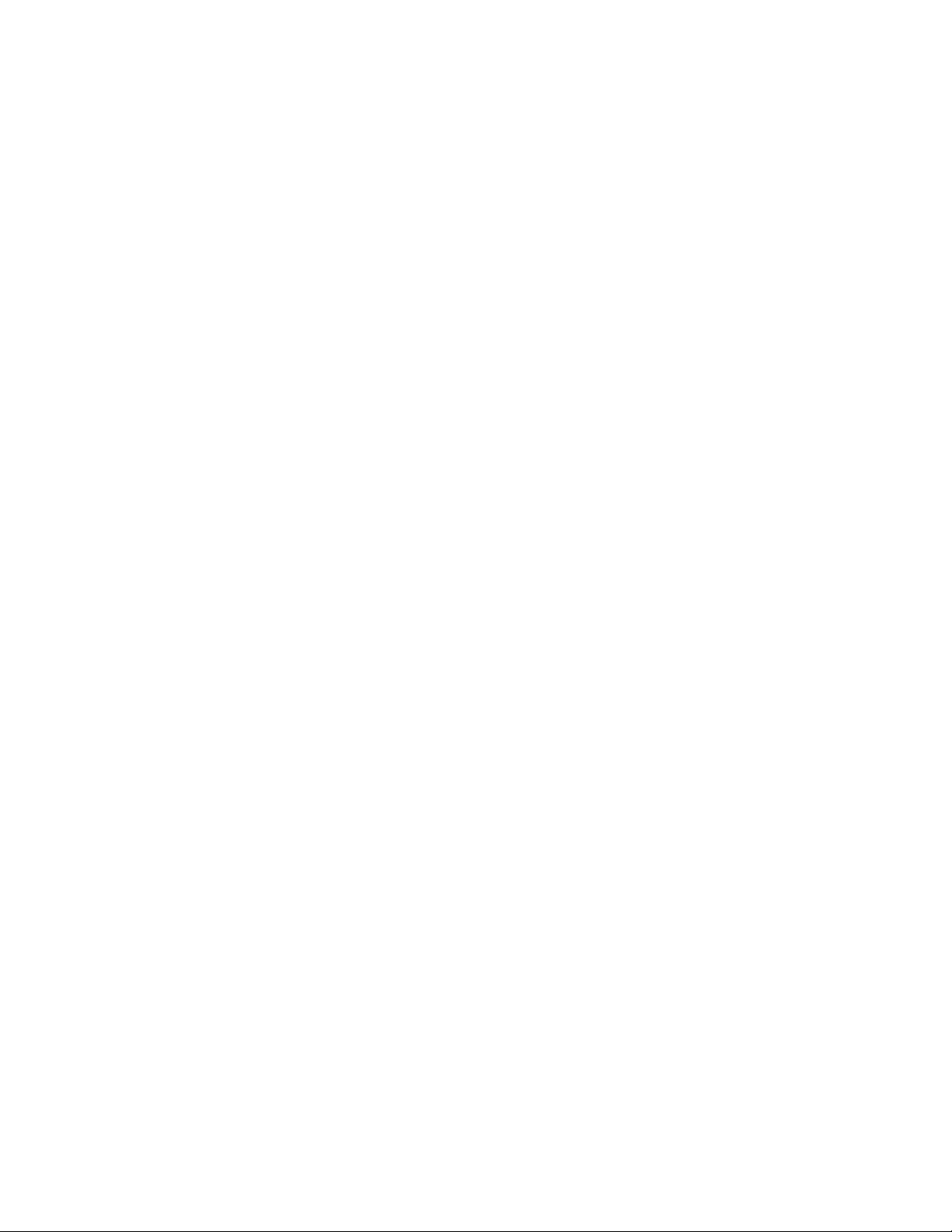
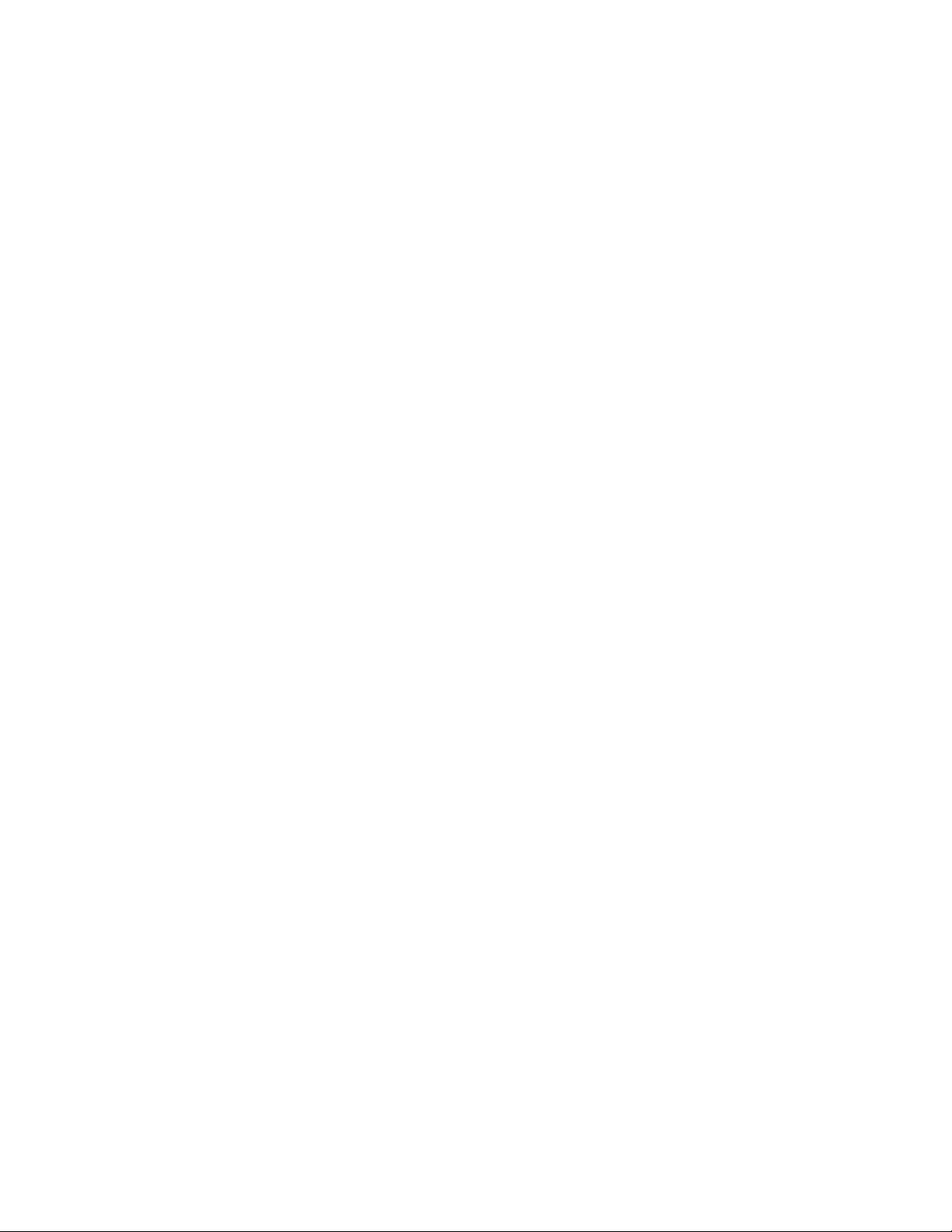
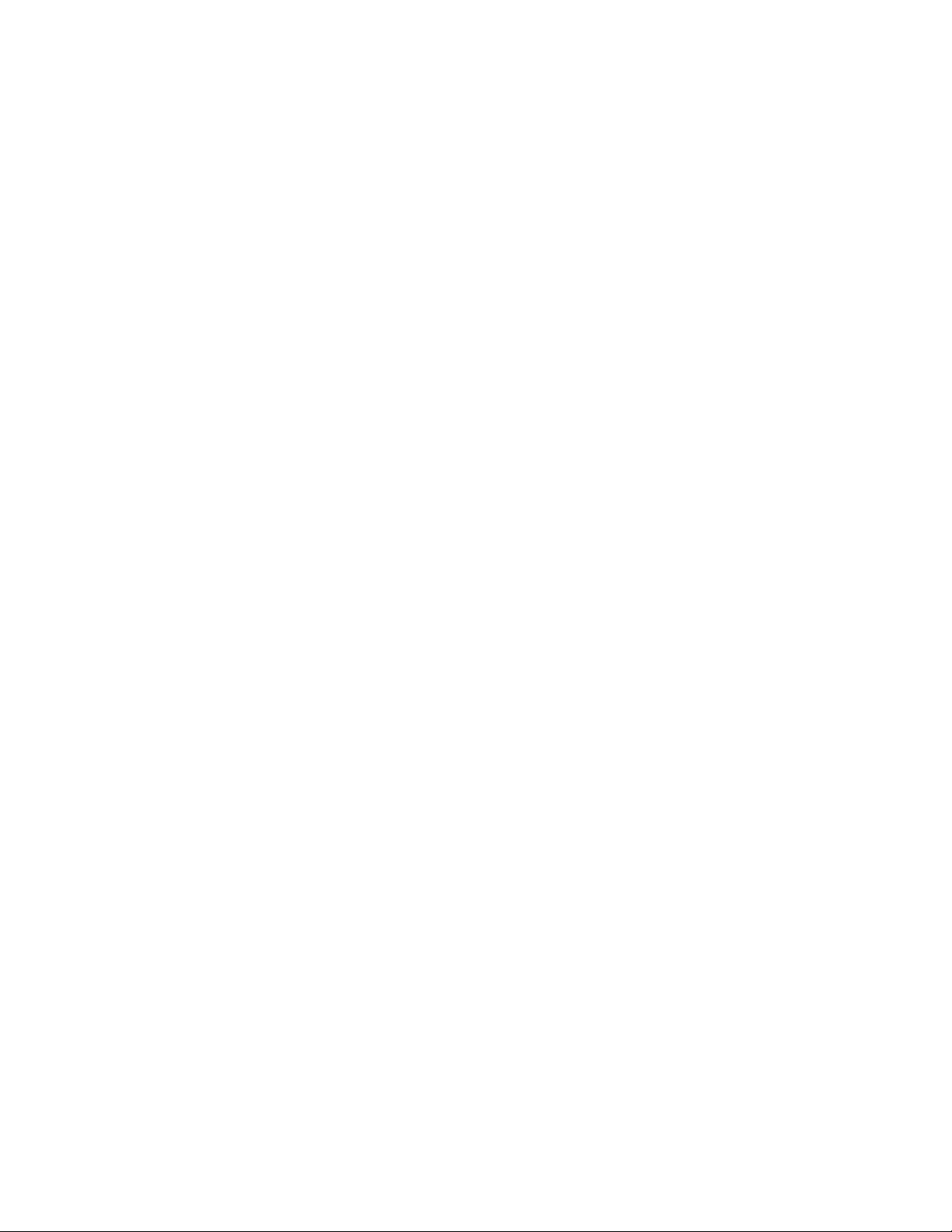
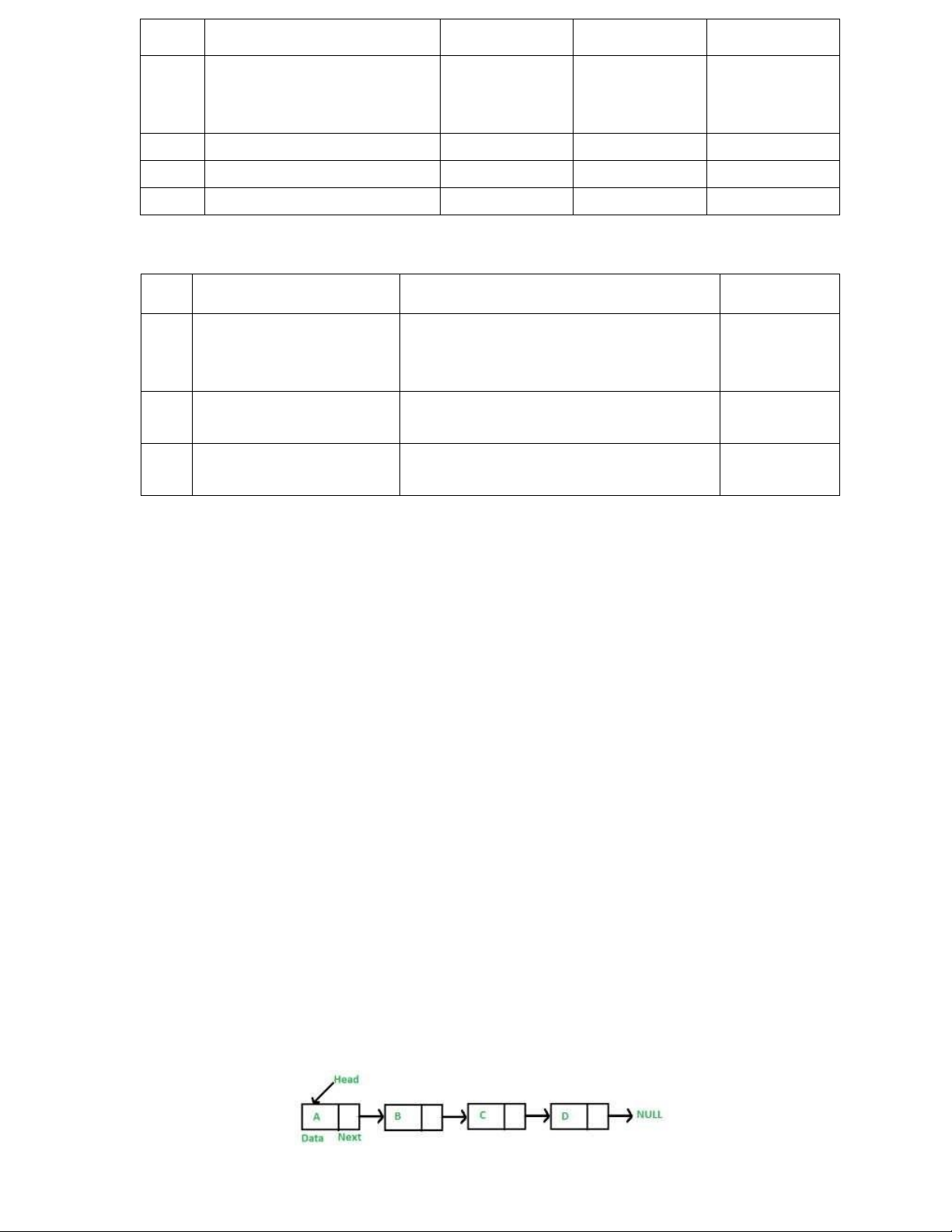
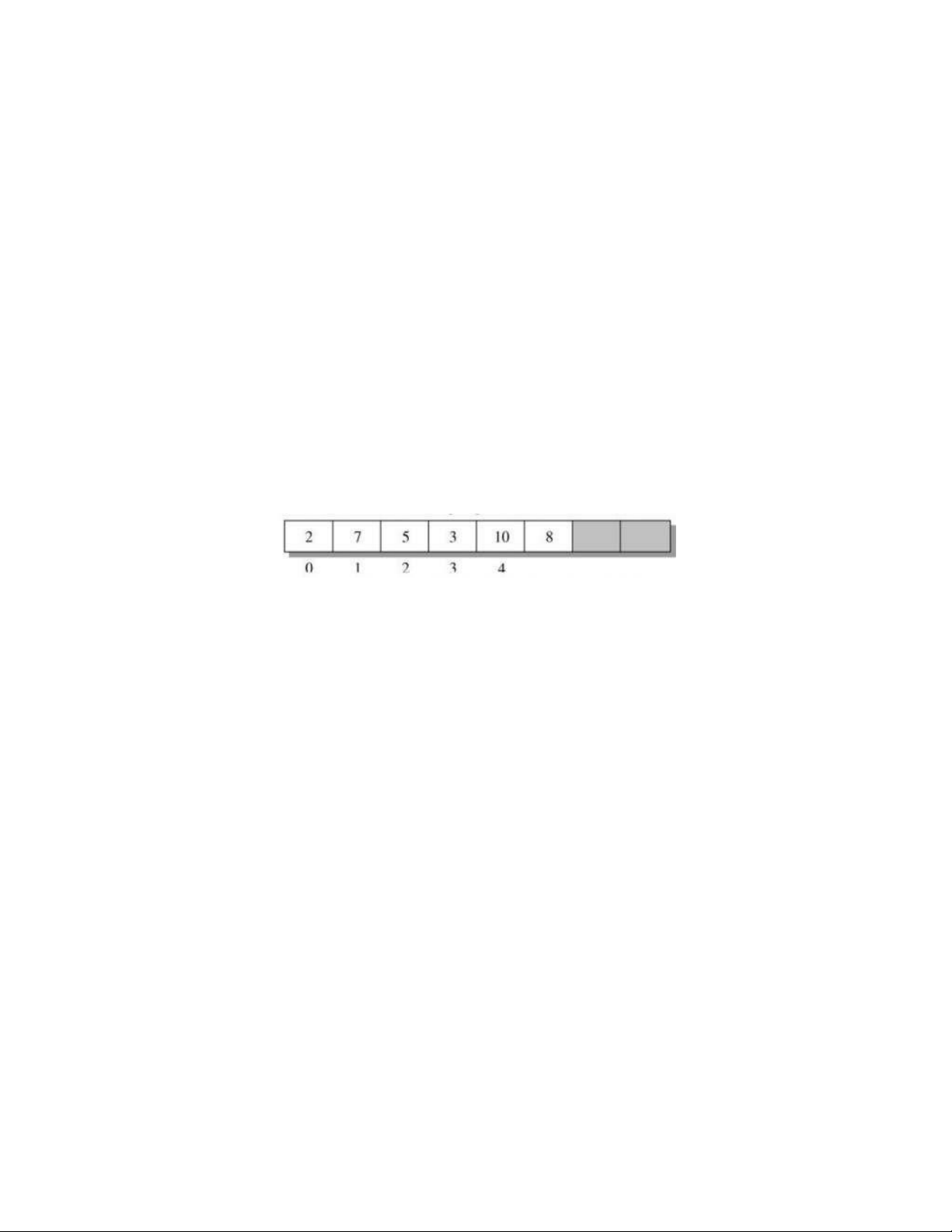
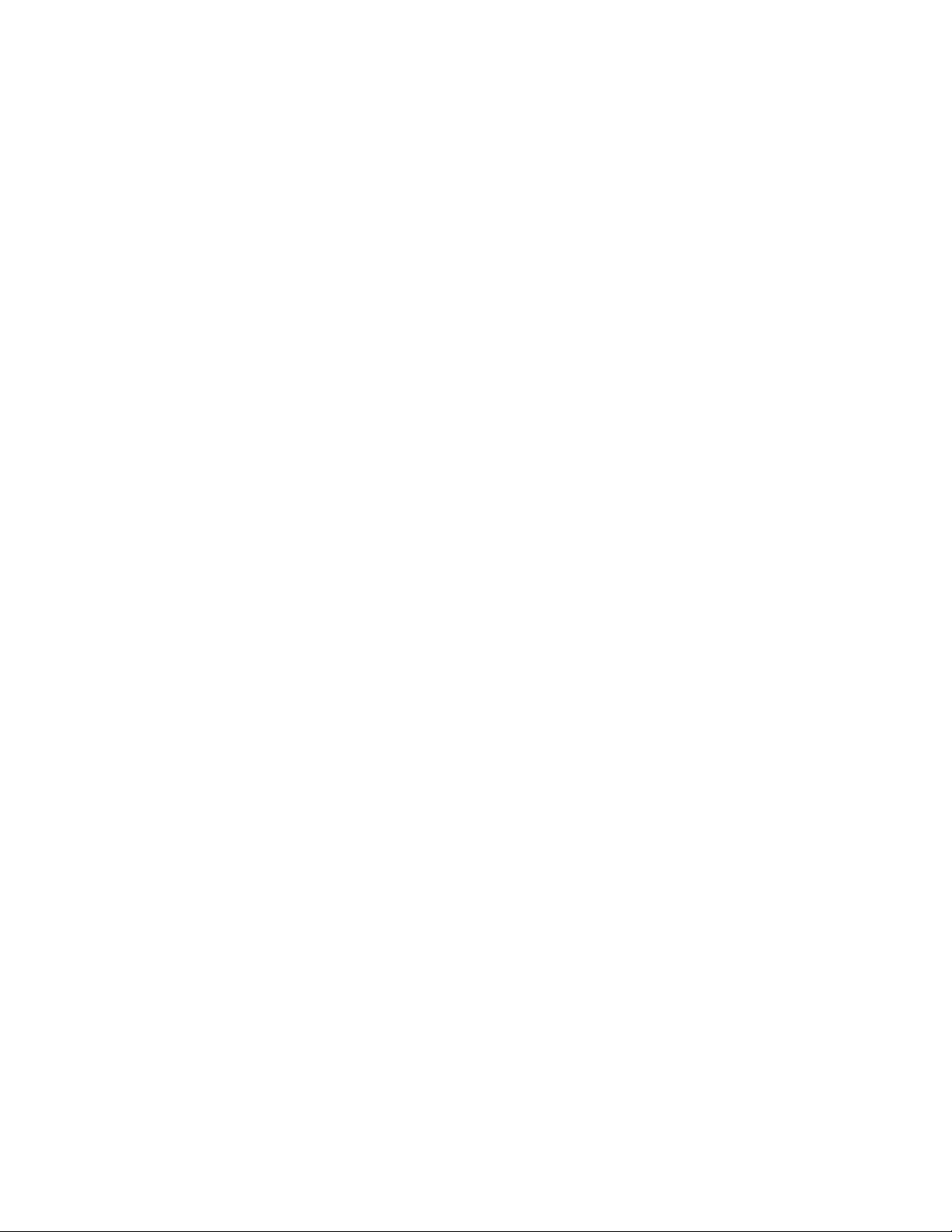
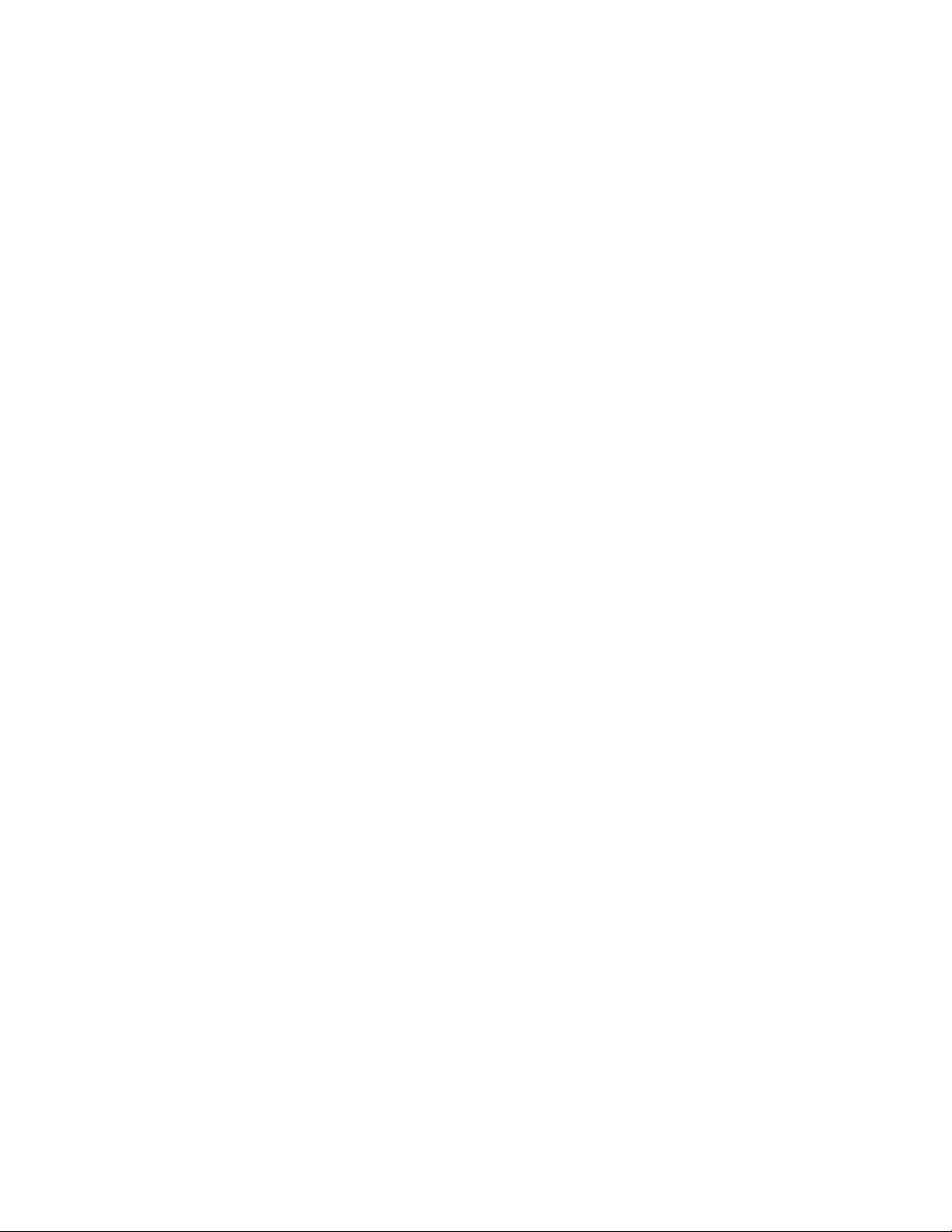
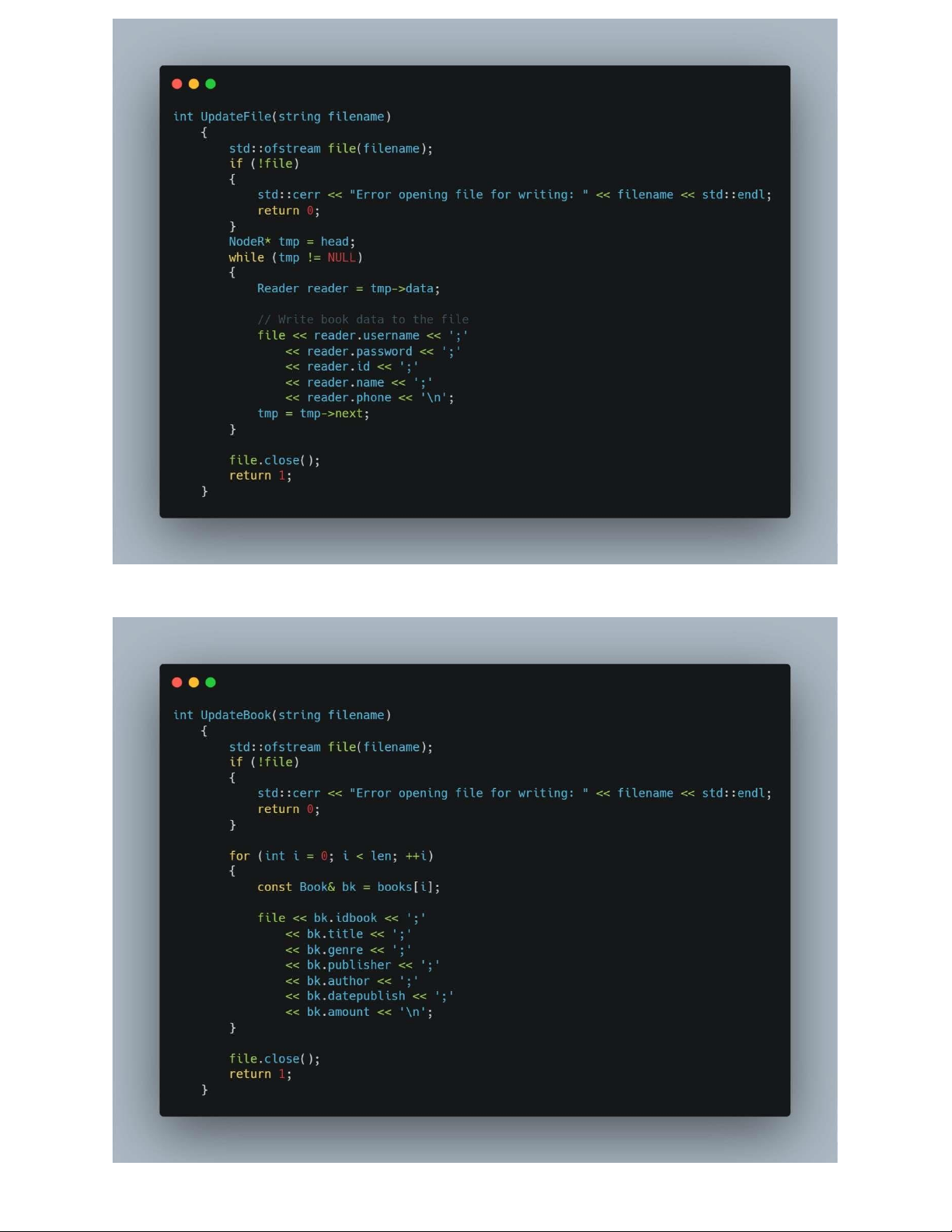
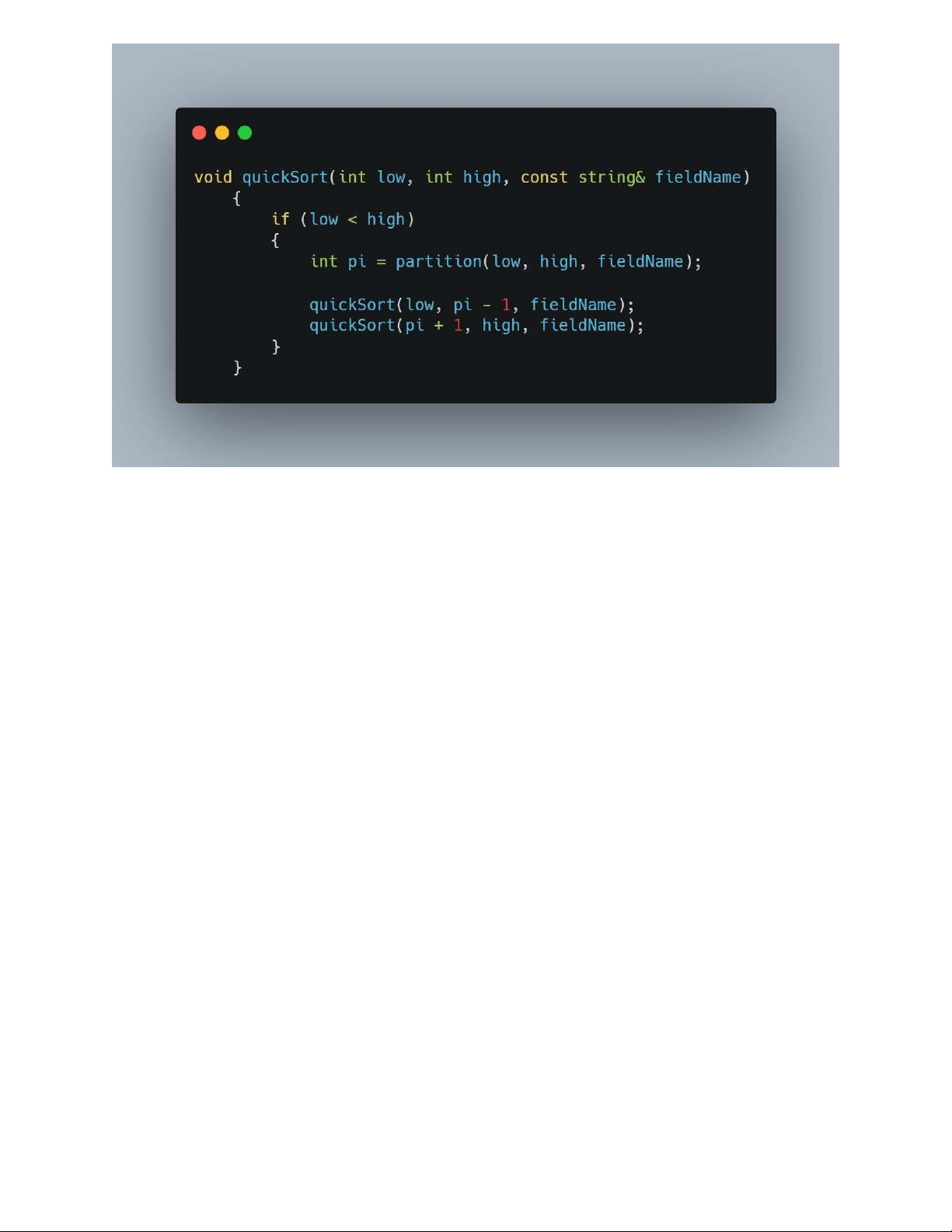
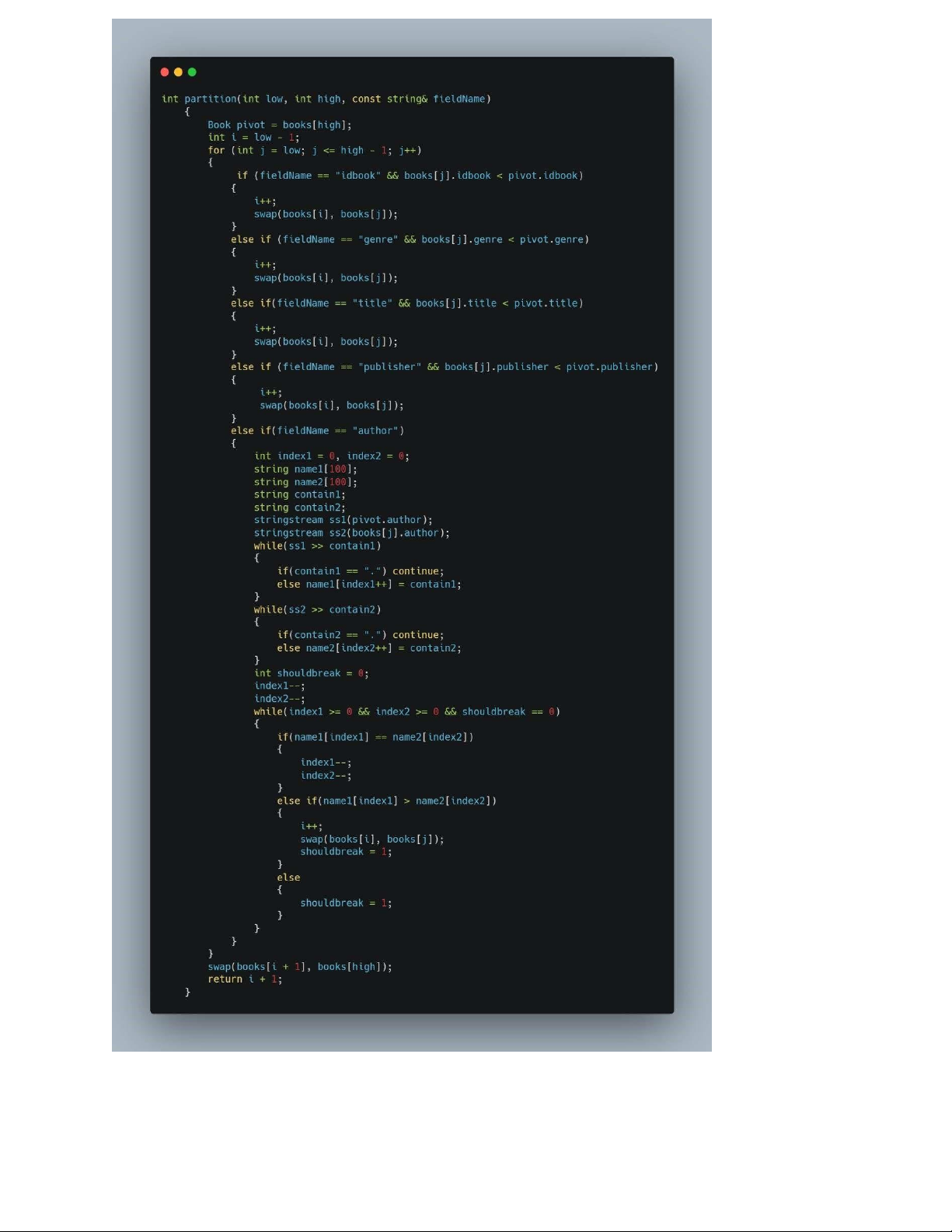
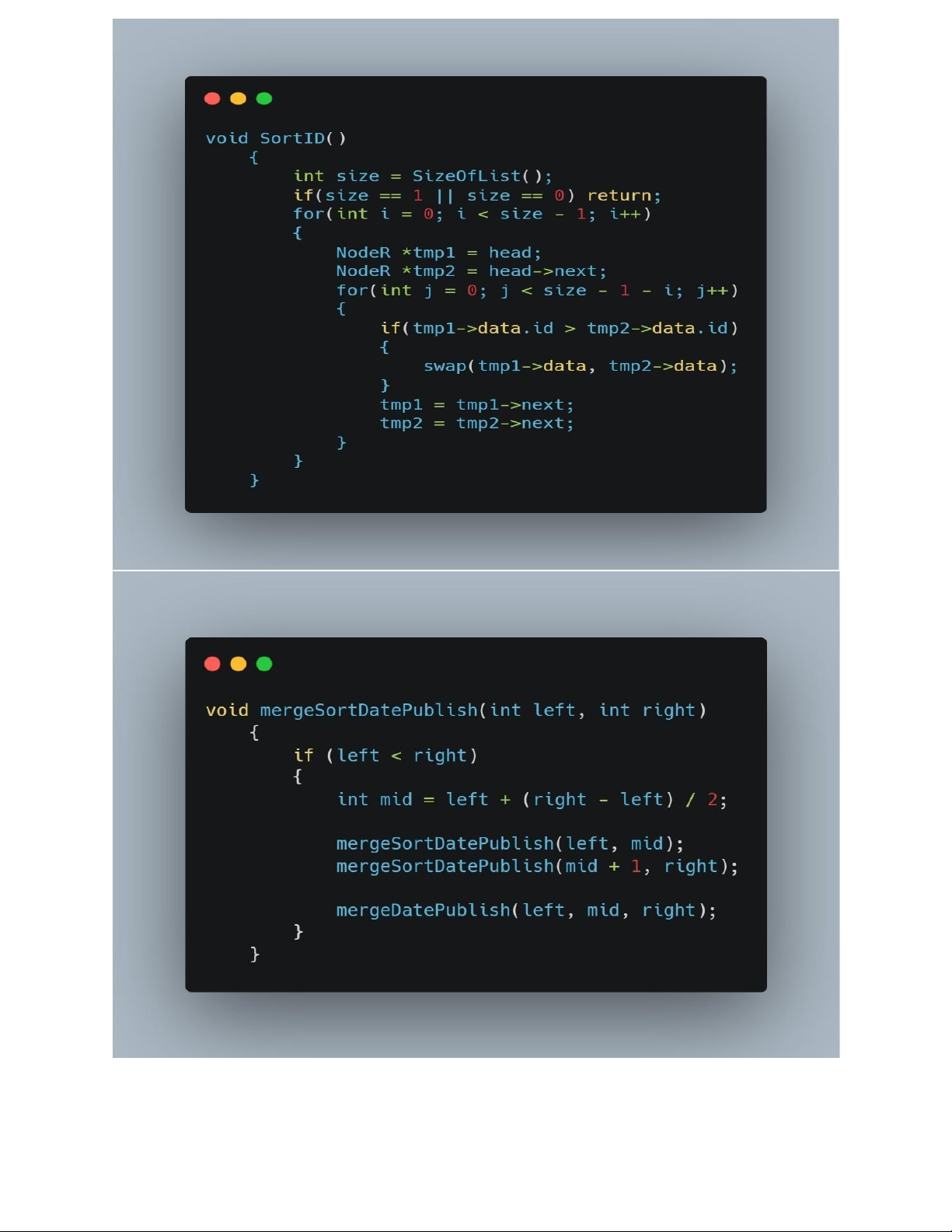
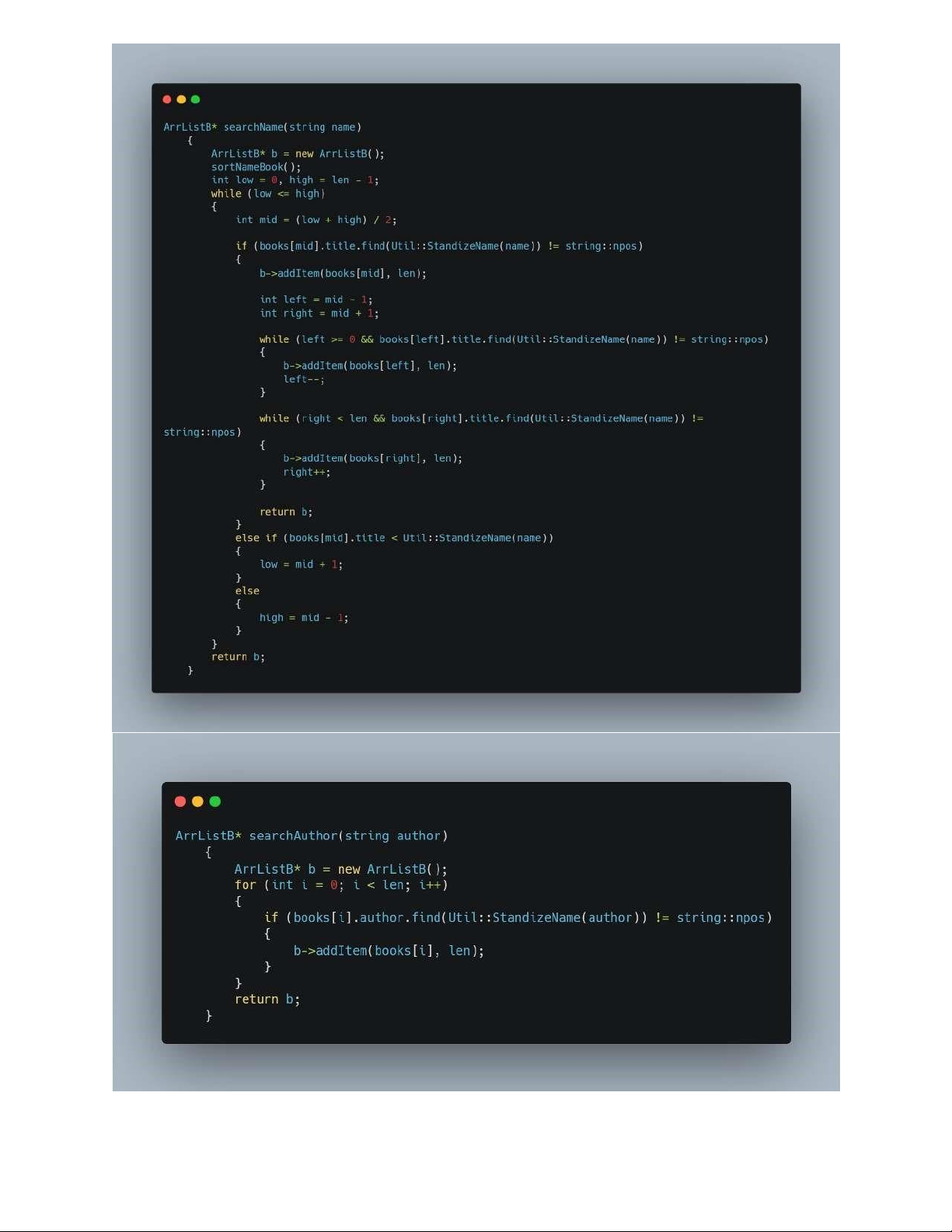
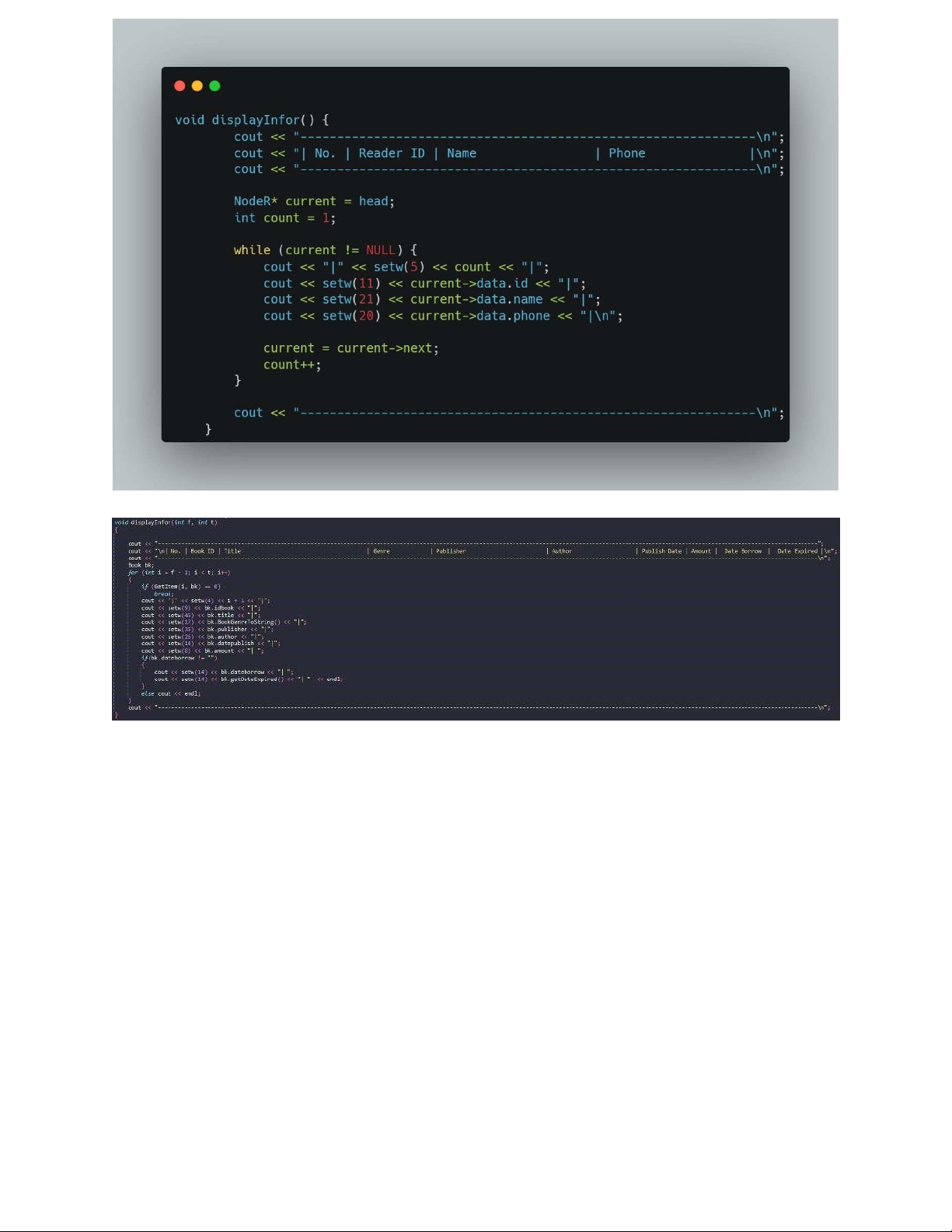
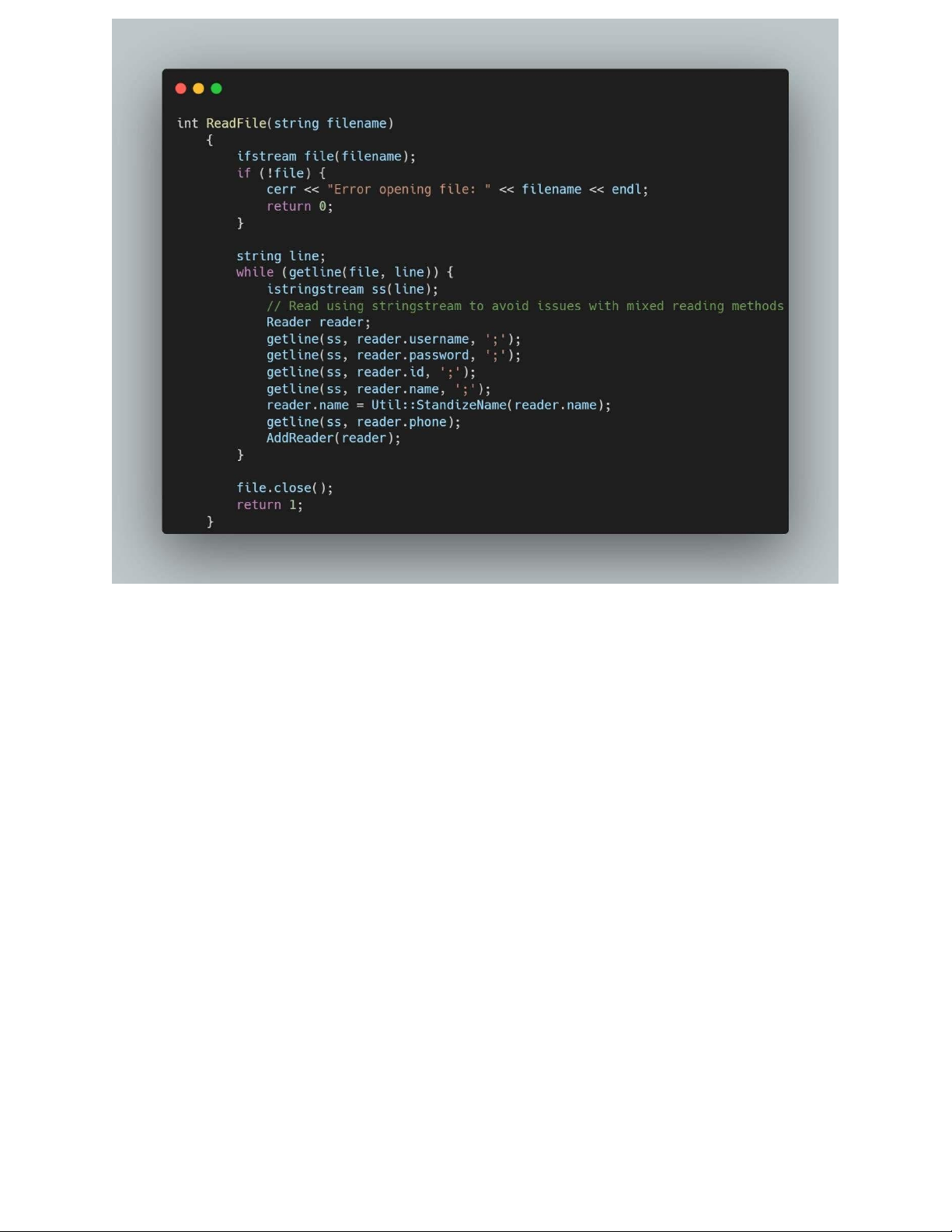
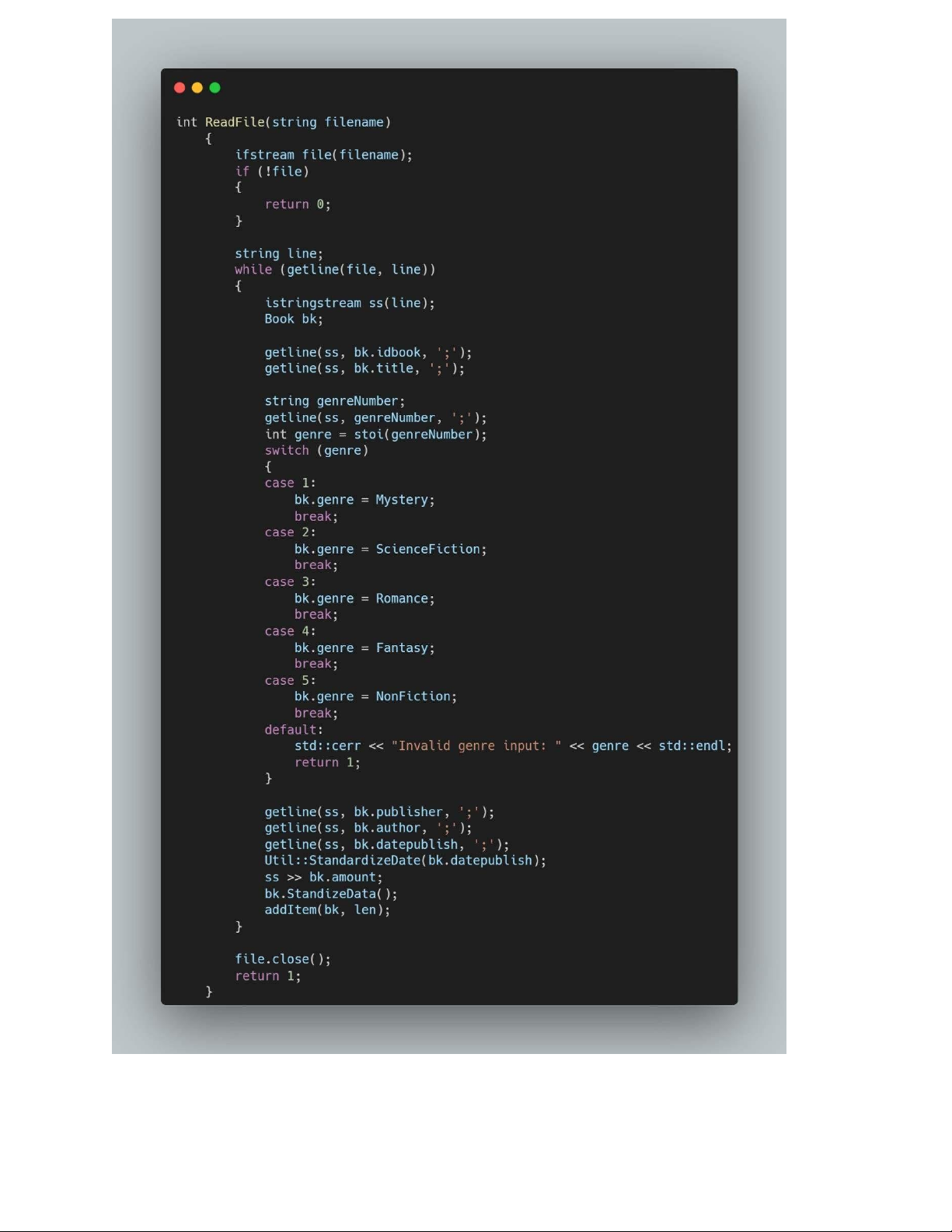
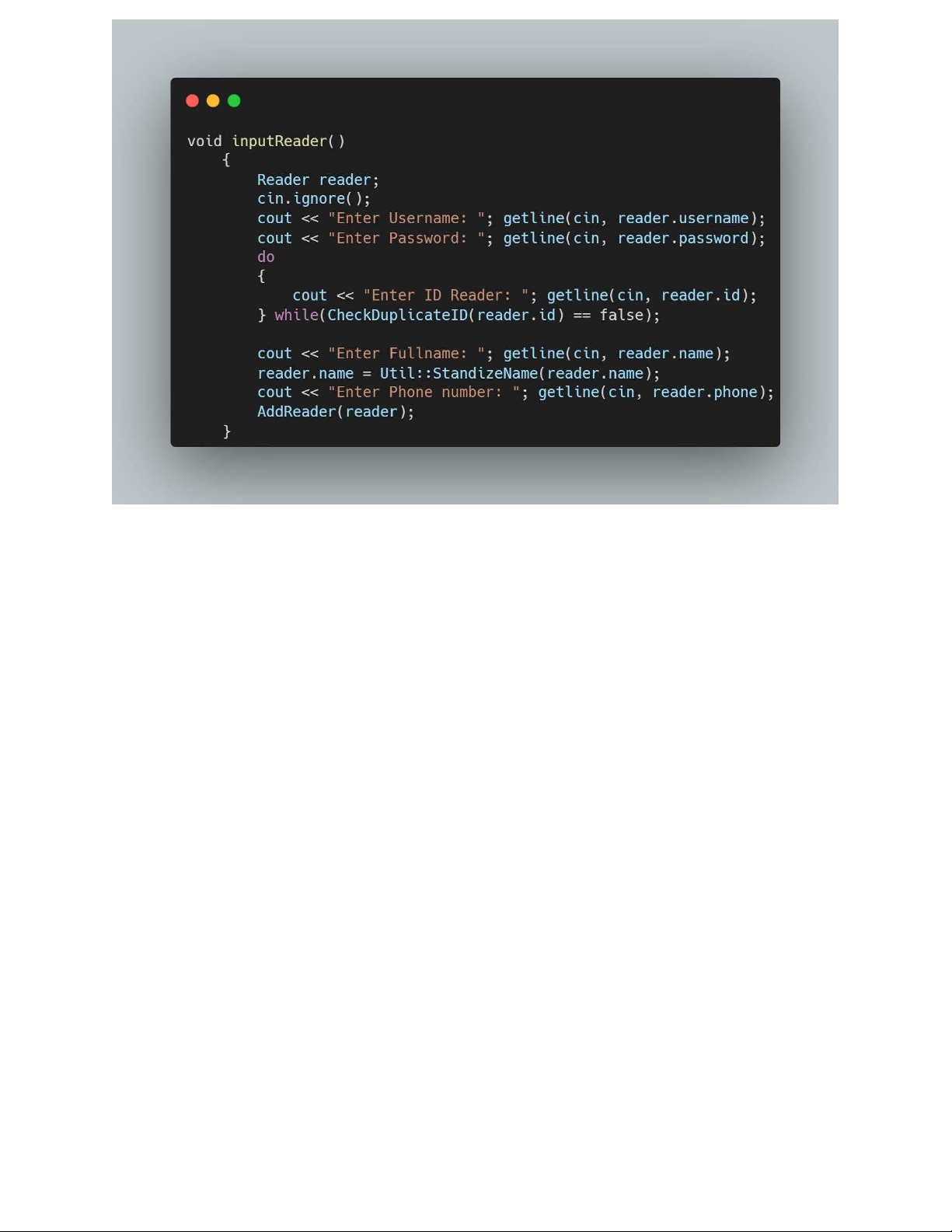
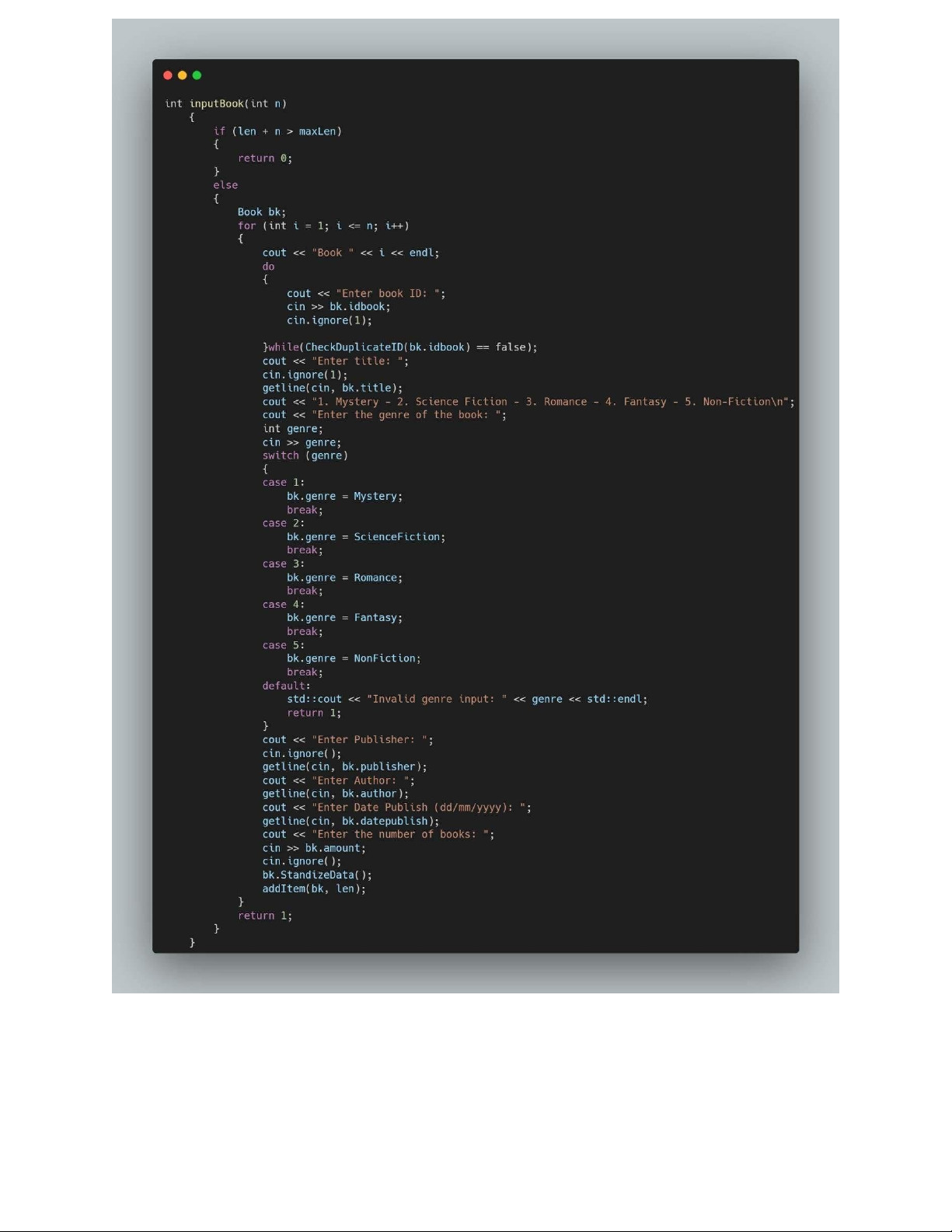
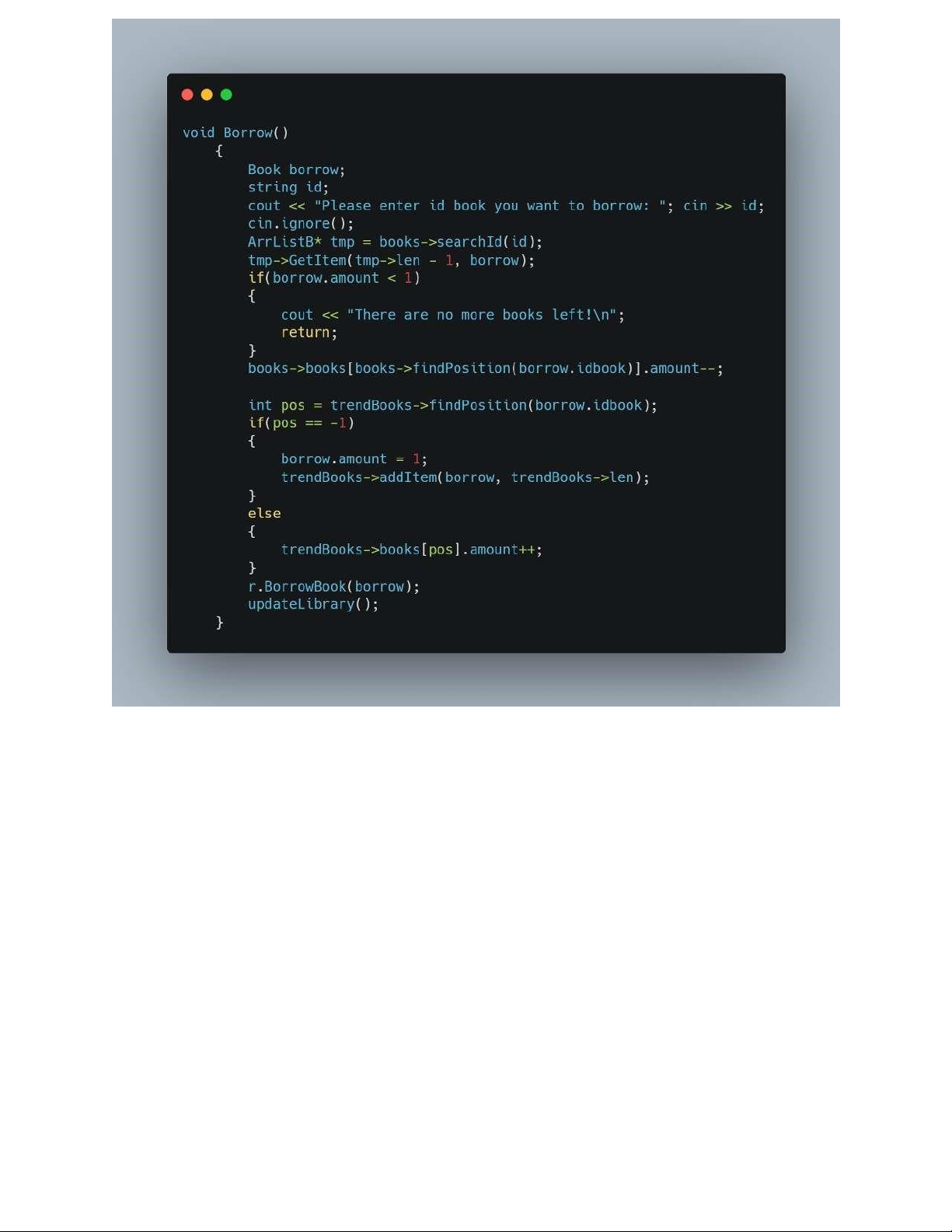
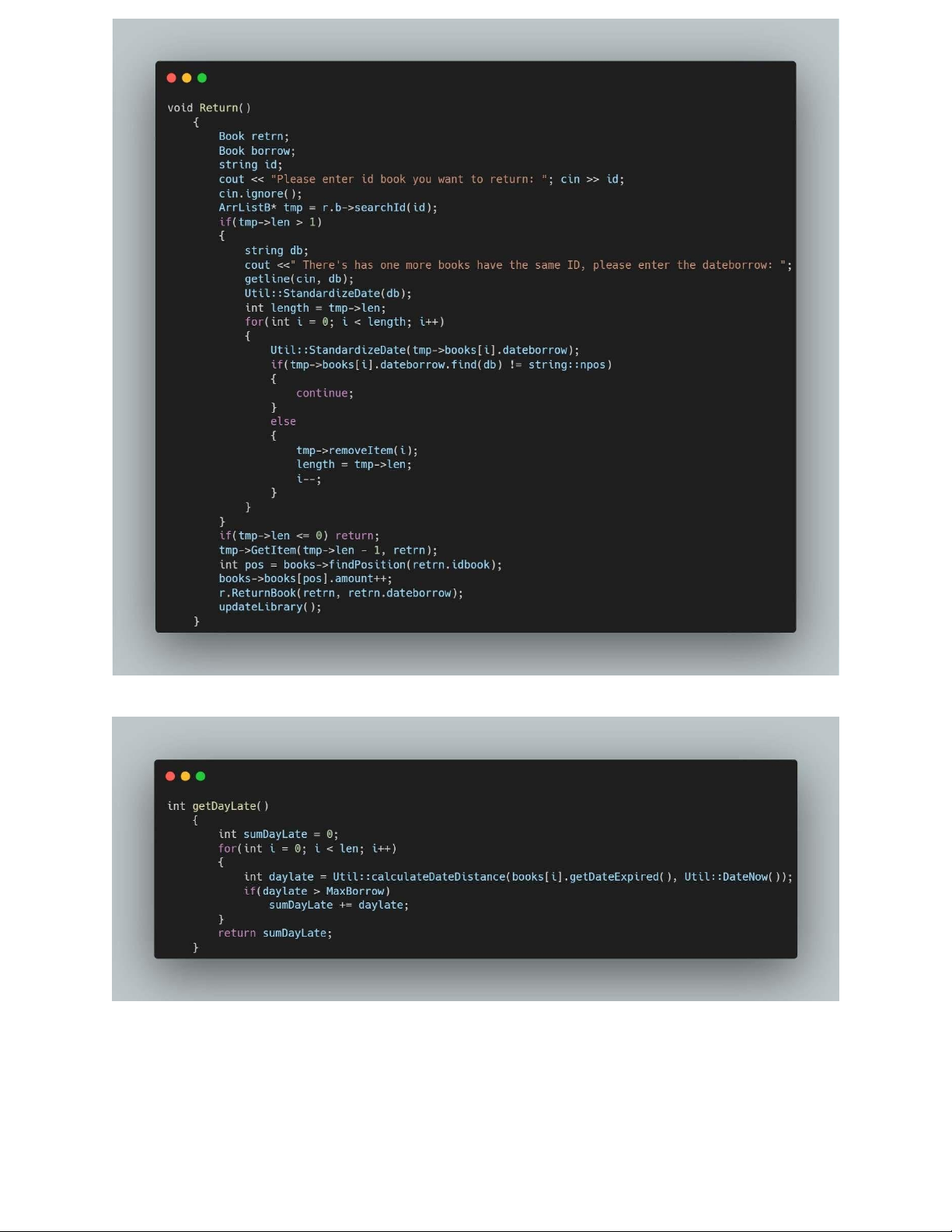
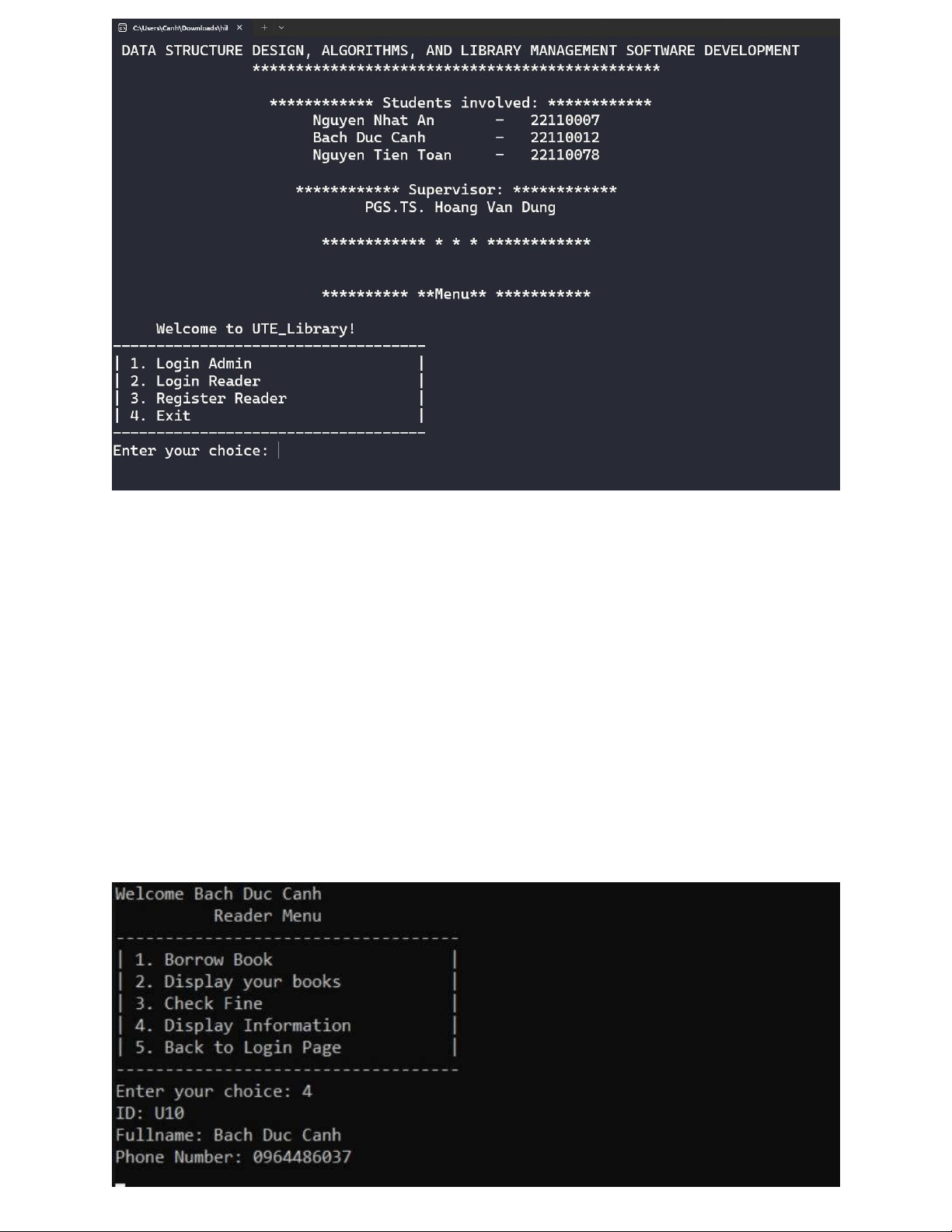
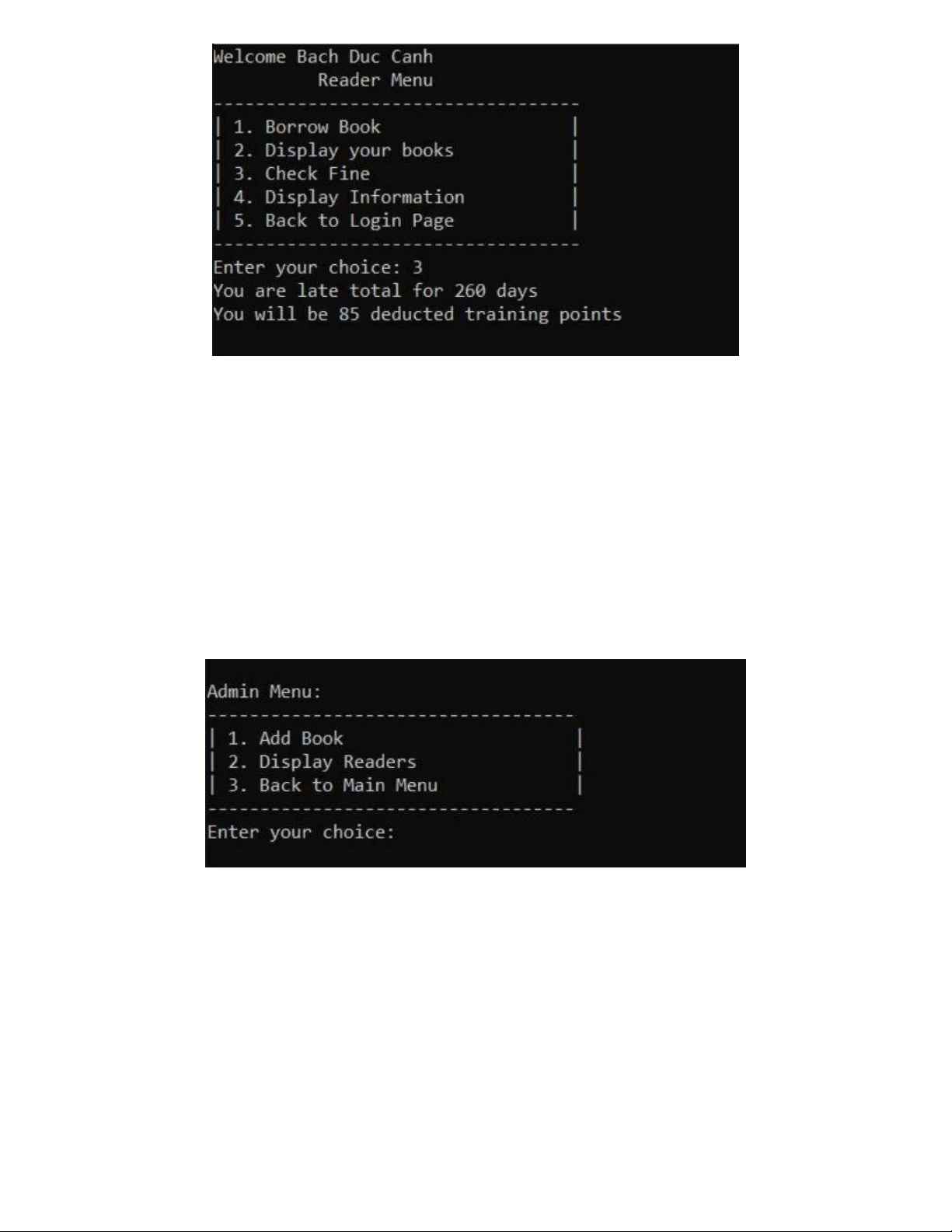
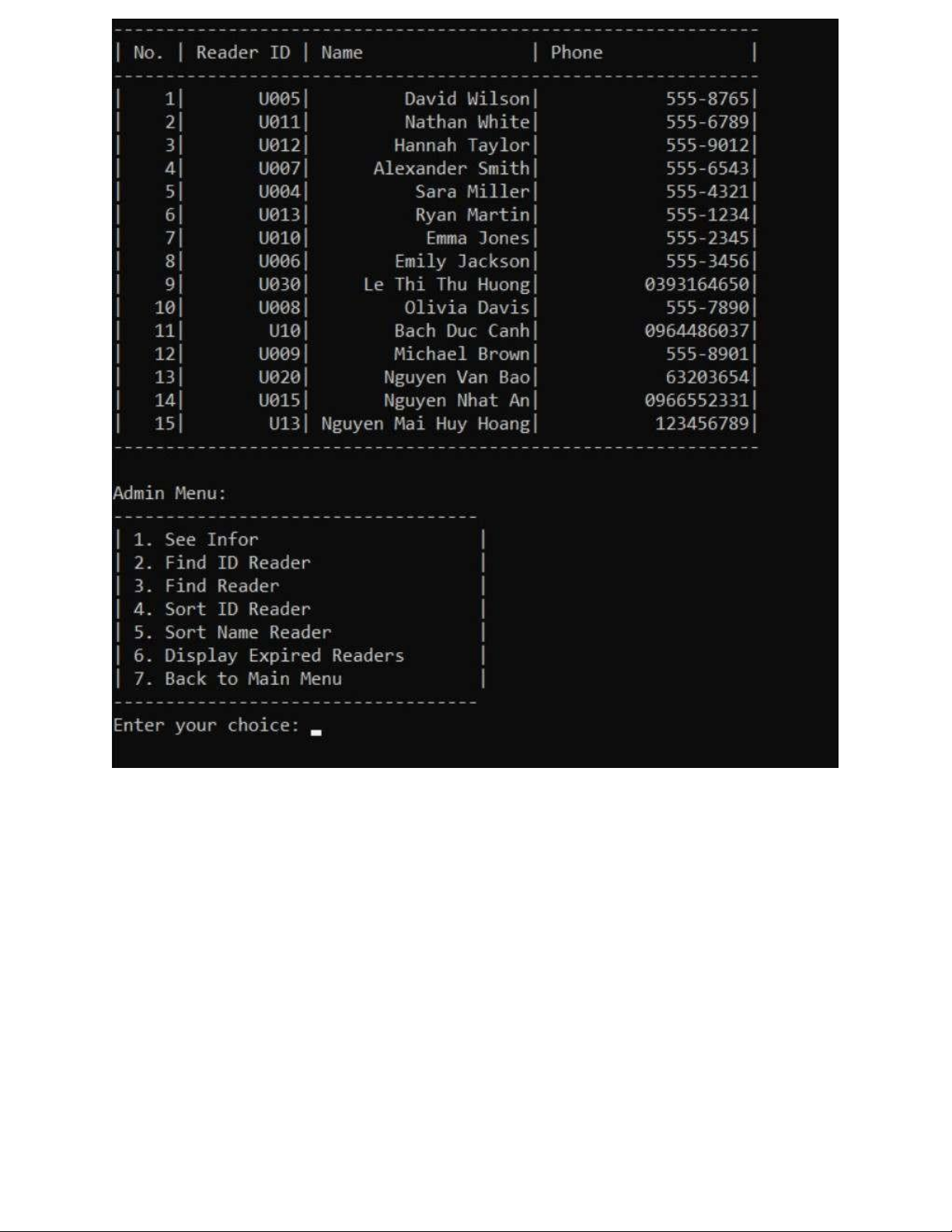
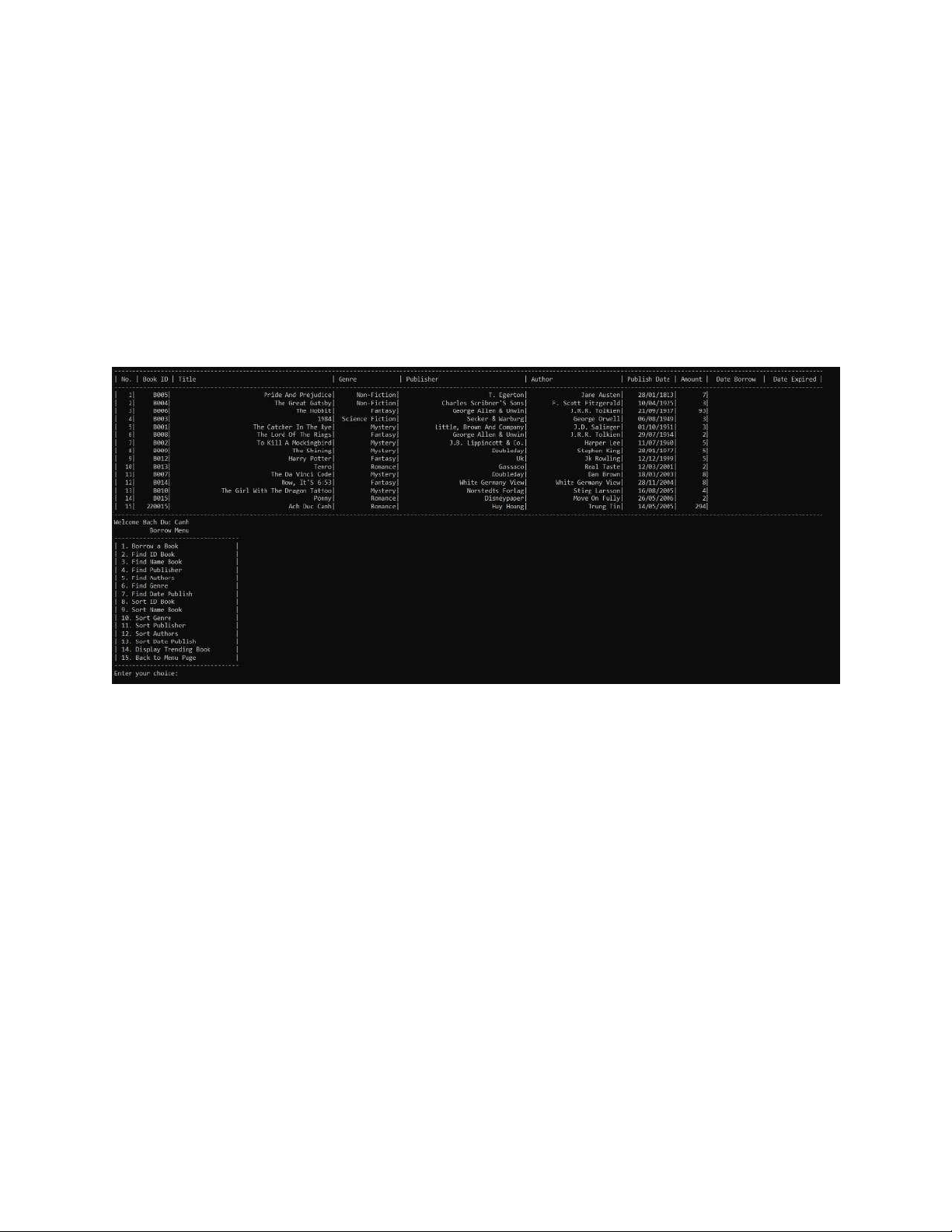
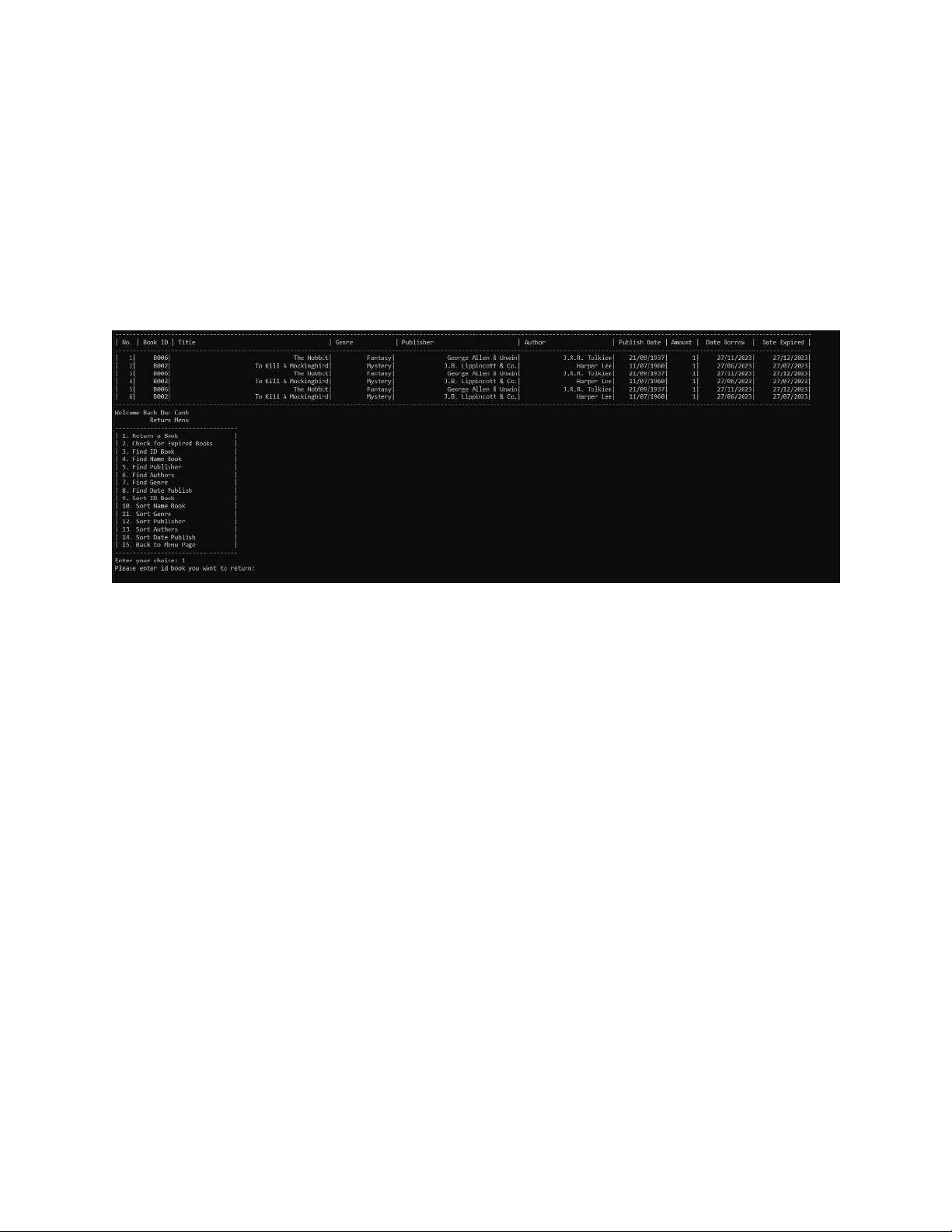
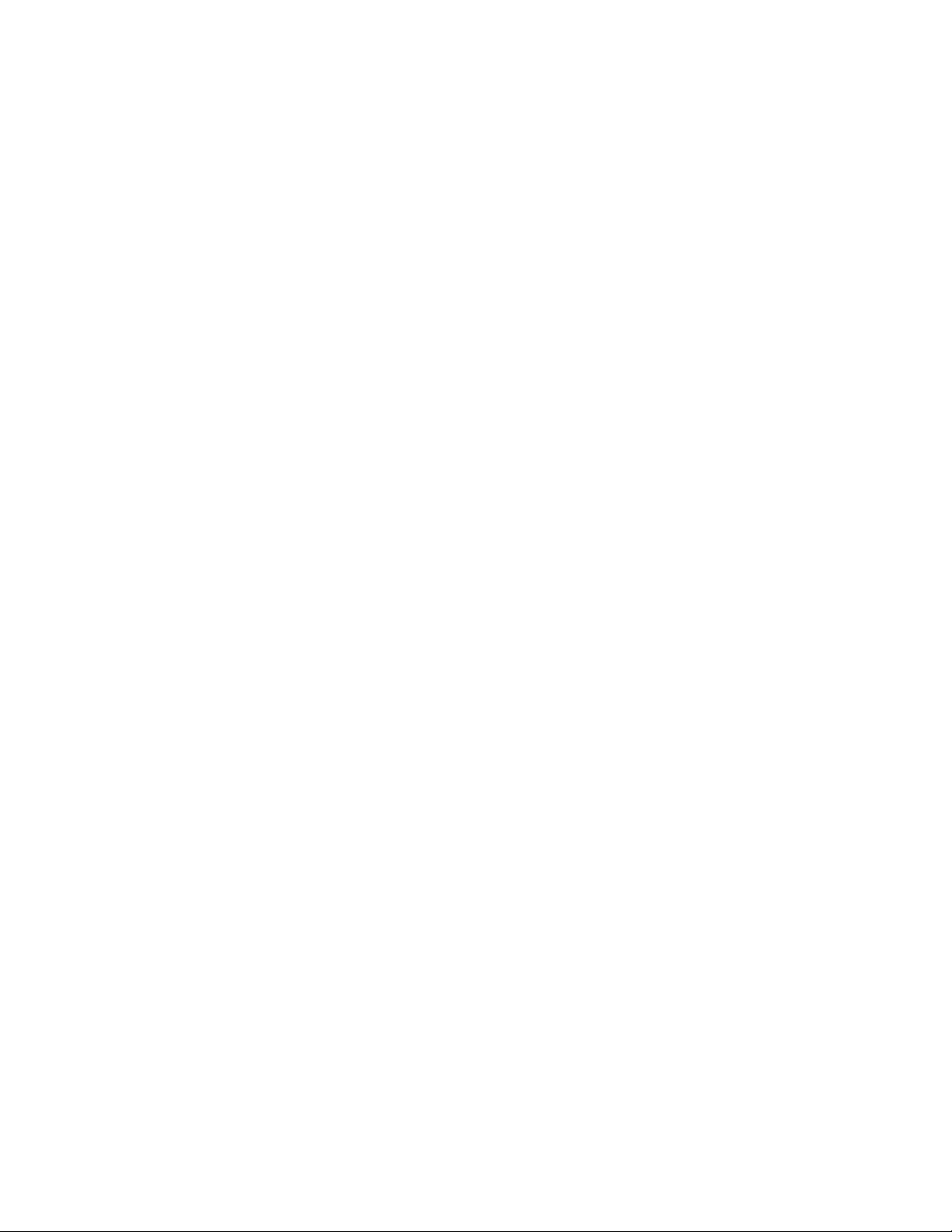
Preview text:
lOMoARcPSD| 36625228
UNIVERSITY OF TECHNOLOGY, HO CHI MINH CITY
FACULTY OF INFORMATION TECHNOLOGY FINAL PROJECT
DATA STRUCTURE DESIGN, ALGORITHMS, AND
LIBRARY MANAGEMENT SOFTWARE DEVELOPMENT
Course: Data Structures and Algorithms
Class: Tuesday, Period 8 - 11
Supervisor: Assoc. Prof. Dr. Hoang Van Dung Students: STUDENT NAME STUDENT ID CONTRIBUTION 1 Nguyễn Nhật An 22110007 100 % 2 Bạch Đức Cảnh 22110012 100 % 3 Nguyễn Tiến Toàn 22110078 100 % lOMoARcPSD| 36625228 ACKNOWLEDGEMENTS
To successfully complete this project and report, we would like to express our
sincere gratitude to all those who assisted us throughout the process.
First and foremost, we extend our deep appreciation to our supervisor, Assoc. Prof.
Dr. Hoang Van Dung, who provided guidance throughout the project. He dedicated
considerable time, effort, and enthusiasm to help us achieve the best results. His valuable
knowledge and experience enhanced our understanding of the topic and the
implementation process. He patiently addressed our questions, assisting us in overcoming challenges.
We also want to thank the esteemed professors in the High-Quality Training
Department and the Information Technology Department for their dedicated teaching,
imparting essential knowledge for our project. This knowledge provided a solid foundation
for us to undertake the project.
Additionally, we express our gratitude to our peers who supported us during the
project. They shared useful information and materials, contributing to the enhancement of our ideas.
This project and report were completed within a limited timeframe and with limited
knowledge. Consequently, there may be some shortcomings in the project. We look
forward to receiving valuable feedback from our professors to improve our understanding
and perform better in the future.
In conclusion, we wish our professors continued health, happiness, and success in their careers.
Once again, we sincerely thank everyone involved. Table of Contents
A. INTRODUCTION .......................................................................................................... 3
1. REASON FOR CHOOSING THE PROJECT............................................................. 3
2. PROJECT FEATURES ................................................................................................ 3
3. SYSTEM ANALYSIS AND DESIGN ........................................................................ 3
4. EXPECTED INTERFACE .......................................................................................... 3
5. IMPLEMENTATION PLAN ...................................................................................... 3
B. CONTENT ...................................................................................................................... 4
1. THEORETICAL FOUNDATIONS ............................................................................. 4
1.1 Object-Oriented Programming (OOP) ................................................................... 4
1.2. Programming Techniques ...................................................................................... 4
2. FUNCTION CREATION ............................................................................................ 7 lOMoARcPSD| 36625228
2.1. Update Information Function ................................................................................ 7
2.2. Sort Function ......................................................................................................... 9
2.3. Search Function ................................................................................................... 12
2.4. Print List Function ............................................................................................... 12
2.5. Read Information Function.................................................................................. 13
2.6. Add Books and Readers ...................................................................................... 15
2.7. Borrow, Return Function ..................................................................................... 17
2.8. Penalty Function (if the book is returned late) .................................................... 19
3. INTERFACE DESIGN, TESTING, AND INSTALLATION .................................. 19
3.1. Menu Login ......................................................................................................... 19
3.2. Menu Reader ....................................................................................................... 20
3.3. Menu Admin ........................................................................................................ 21
3.4. Menu Borrow book .............................................................................................. 22
3.5. Menu Return book ............................................................................................... 23
C. Conclusion .................................................................................................................... 24
1. Conclusion .................................................................................................................. 24
2. Development directions ............................................................................................. 25 lOMoARcPSD| 36625228 A. INTRODUCTION
1. REASON FOR CHOOSING THE PROJECT
This project has high practicality in real-life situations. Library management is a
practical issue that libraries worldwide are facing. The project allows students to apply
knowledge of data structures and algorithms to build an efficient library management
system. An effective system facilitates easy management of information about books,
documents, readers, as well as book borrowing and return activities. This project helps
students develop programming skills, including problem analysis, coding, testing, and code maintenance.
Moreover, the project demonstrates creativity as students can design a library
management system according to their ideas, tailored to the specific needs of a library. 2. PROJECT FEATURES
- Add, edit, delete, and update books if there are changes.
- Add, edit, delete, and update customer information if there are changes.
- Sort lists of books and customers (in various ways).
- Search for books and customers (using various criteria).
- Print lists of books and customers. - Save updated information.
- Library management (borrow, return, late return, etc.).
3. SYSTEM ANALYSIS AND DESIGN
A library needs a program to manage bookshelves, customer information, and the
status of books, including borrowed and returned books. The system includes:
- Each book has a Book Code, borrowing date, return date, borrowing information,
reader information, and status (returned or not).
- Readers have a reader code, reader name, phone number, account, and password. 4. EXPECTED INTERFACE
The main menu page has five main functions: Login, Admin, Reader, Borrow Books, and Return Books:
- The Login or Register page is used to access the system.
- The admin's homepage includes options for finding, editing, and adding books.
- The reader's homepage includes options for borrowing, returning books, late days, and reader information.
- The Borrow Books page includes options for borrowing, finding, and sorting books.
- The Return Books page includes options for returning, finding, and sorting books. 5. IMPLEMENTATION PLAN a) Plan lOMoARcPSD| 36625228 Week Tasks Start Date End Date Results Choose a topic, analyze the 12
project, clarify information 6/11/2023 11/11/2023 Completed to be managed 13 Code writing 13/11/2023 18/11/2023 Completed 14 Code optimization 20/11/2023 25/11/2023 Completed 15 Report writing 25/11/2023 27/11/2023 Completed Table 1: Project plan b) Task Assignment Student Name Description of Task Contribution Code writing 1 Nguyễn Nhật An Refining algorithms and data 100% structure Design interface, functions. 2 Bạch Đức Cảnh 100% Code writing Project analysis 3 Nguyễn Tiến Toàn 100% Code writing Table 2: Task Assignment B. CONTENT
1. THEORETICAL FOUNDATIONS
1.1 Object-Oriented Programming (OOP)
Object-Oriented Programming (OOP) is a programming technique that allows
programmers to create abstract objects in code, abstracting real-life objects.
An object comprises two components: attributes and methods. Attributes represent
information or characteristics, while methods represent actions an object can perform. 1.2. Programming Techniques
1.2.1. Linked List Data Structure
A linked list is a data structure composed of nodes connected through links. Each
node contains data and a reference to the next node in the sequence. Linked lists are the
second most commonly used data structure after arrays. Basic concepts related to linked lists include:
• Link: Each link of a linked list can hold data, referred to as an element.
• Next: Each link contains a reference to the next link, known as Next.
• Head: A linked list comprises links connected to the first link called Head. lOMoARcPSD| 36625228
Figure 1.1: Representation of a linked list
Basic operations in linked lists:
• Insert operation: adding an element to the beginning of the linked list.
• Delete operation (at the head): removing an element from the beginning of the linked list.
• Display: showing the entire linked list.
• Search operation: searching for an element using a provided key.
1.2.2. Array List Data Structure
An array list is a dynamic array-like data structure that allows for flexible resizing.
Unlike a linked list, an array list stores elements in contiguous memory locations. Basic
concepts related to array lists include:
• Element: Each element in an array list holds data.
• Capacity: The array list has a capacity that determines the maximum number of elements it can hold.
• Size: The size represents the current number of elements in the array list.
Figure 1.2: Representation of an array list
Basic operations in array lists:
• Insert operation: Adding an element to the array list.
• Delete operation: Removing an element from the array list.
• Display: Showing the entire array list.
• Search operation: Searching for an element using a provided key.
1.2.3. Merge Sort Sorting Method
Merge Sort is a popular sorting algorithm that follows the divide-and-conquer
paradigm. It divides the input array into two halves, recursively sorts each half, and then
merges the sorted halves. The key steps in the Merge Sort algorithm are as follows:
- Divide: Divide the unsorted array into two halves.
- Conquer: Recursively sort each half.
- Merge: Merge the sorted halves to produce a single sorted array.
The merging process involves comparing elements from the two halves and placing
them in the correct order. Merge Sort is known for its stability and consistent performance,
making it suitable for large datasets. Algorithm Steps:
- If the array has fewer than two elements, return as it is already sorted. lOMoAR cPSD| 36625228
- Divide the array into two halves.
- Recursively apply Merge Sort to each half.
- Merge the two sorted halves into a single sorted array.
1.2.4. Bubble Sort Sorting Method
Bubble Sort is a simple sorting algorithm that repeatedly steps through the list,
compares adjacent elements, and swaps them if they are in the wrong order. The pass
through the list is repeated until the list is sorted. Bubble Sort is named for the way smaller
elements "bubble" to the top of the list during each pass. Algorithm Steps:
- Start from the beginning of the list.
- Compare each pair of adjacent elements.
- If the elements are in the wrong order, swap them.
- Continue comparing and swapping until the end of the list is reached.
- Repeat the process from the beginning until no swaps are needed (indicating the list is sorted).
1.2.5. Quick Sort Sorting Method
Quick Sort is a widely used sorting algorithm known for its efficiency and speed. It
is a divide-and-conquer algorithm that works by selecting a 'pivot' element from the array
and partitioning the other elements into two sub-arrays according to whether they are less
than or greater than the pivot. The sub-arrays are then sorted recursively. Algorithm Steps:
- Choose a pivot element from the array.
- Partition the array into two sub-arrays: elements less than the pivot and elements greater than the pivot.
- Recursively apply Quick Sort to the two sub-arrays.
- Concatenate the sorted sub-arrays along with the pivot to get the final sorted array. 1.2.6. Linear Search Method
Linear Search, also known as sequential search, is a simple searching algorithm that
looks for a target value within a list. It sequentially checks each element of the list until a
match is found or the entire list has been traversed. Algorithm Steps:
- Start from the first element of the list.
- Compare the target value with the current element. lOMoARcPSD| 36625228
- If the target value matches the current element, return the index of the element.
- If no match is found, move to the next element in the list.
- Repeat steps 2-4 until a match is found or the end of the list is reached.
- If the end of the list is reached without finding a match, return a special value (e.g.,
-1) to indicate that the target value is not in the list. 1.2.7. Binary Search Method
Binary Search is an efficient searching algorithm that seeks a target value within a
sorted list. The process involves repeatedly dividing the search interval in half, reducing
the search space by half with each comparison. Algorithm Steps:
- Initialization: Define the search interval, typically the entire sorted list.
- Midpoint Calculation: Find the midpoint of the current interval.
- Comparison: Compare the target value with the midpoint.
- Adjust Interval: Narrow down the search interval based on the comparison. If the
target is less than the midpoint, search the left half. If the target is greater than the
midpoint, search the right half.
- Repeat: Repeat the process until the target is found or the search interval becomes empty.
Binary Search has a time complexity of O(log n), making it highly efficient for large datasets. 2. FUNCTION CREATION
2.1. Update Information Function 2.1.1. For Readers lOMoARcPSD| 36625228 2.1.2. For Books lOMoARcPSD| 36625228 2.2. Sort Function lOMoARcPSD| 36625228 lOMoARcPSD| 36625228 lOMoARcPSD| 36625228 2.3. Search Function 2.4. Print List Function 2.4.1. For Readers lOMoARcPSD| 36625228 2.4.2. For Books
2.5. Read Information Function 2.5.1. For Readers lOMoARcPSD| 36625228 2.5.2. For Books lOMoARcPSD| 36625228 2.6. Add Books and Readers 2.6.1. For Readers lOMoARcPSD| 36625228 2.6.2. For Books lOMoARcPSD| 36625228 2.7. Borrow, Return Function 2.7.1 Borrow Book lOMoARcPSD| 36625228 2.7.2 Return Book lOMoARcPSD| 36625228
2.8. Penalty Function (if the book is returned late)
3. INTERFACE DESIGN, TESTING, AND INSTALLATION 3.1. Menu Login
The interface of the library management software is designed to be simple and user-
friendly, facilitating easy interaction for users. The main interface is designed in the style
of a website or application with functions such as login for the admin, login for readers,
and reader account registration. It includes a banner displaying information about the contributors. lOMoARcPSD| 36625228
Figure 3.1: The interface upon launching the program. 3.2. Menu Reader
The reader’s menu options include:
1. Borrow Book: Allows the reader to borrow books from the library collection.
2. Display Your Books: Displays a list of books currently borrowed by the reader.
3. Check Fine: Enables the reader to check if there are any fines associated with their account.
4. Display Information: Shows essential information about the reader, such as ID, name, and phone number.
5. Back to Login Page: Provides an option to return to the login page for further actions. lOMoARcPSD| 36625228
Figure 3.2: The reader menu interface is performing the information display function.
Figure 3.3: The reader menu interface is performing the check fine function. 3.3. Menu Admin
The admin’s menu options include:
1. Add Book: Facilitates the addition of new books to the library collection.
2. Display Readers: Allows the admin to check basic information of the readers and
and provide functions to interact the reader's information
3. Back to Main Menu: Provides an option to return to the main menu for further actions.
Figure 3.4: The admin menu interface. lOMoARcPSD| 36625228
Figure 3.5: The admin menu interface is performing the display readers function. 3.4. Menu Borrow book.
When you select the "Borrow Book" function from the reader menu, you will be
redirected to the "Borrow menu” where display a list of all books in library and some
options for you to perform tasks such as borrowing books by ID, searching for books, and
sorting books to facilitate your borrowing process. The “Borrow Menu” options include:
1. Borrow Book: Initiate the process of borrowing a book.
2. Find ID Book: Search for a book by its ID.
3. Find Name Book: Search for a book by its name.
4. Find Publisher: Locate books by a specific publisher.
5. Find Authors: Discover books written by specific authors.
6. Find Genre: Explore books based on their genre. lOMoARcPSD| 36625228
7. Find Date Publish: Search for books published on a particular date.
8. Sort ID Book: Arrange the list of books based on their ID.
9. Sort Name Book: Alphabetically sort the list of books by name.
10.Sort Genre: Organize the list of books by genre.
11.Sort Publisher: Sort books based on their publisher.
12.Sort Authors: Arrange books based on the authors.
13.Sort Date Publish: Sort books based on their date of publication.
14.Display Trending Book: Display frequently borrowed books
15.Back to Menu Page: Return to the main menu for additional actions.
Figure 3.6: The borrow menu interface. 3.5. Menu Return book.
When you select the "Display your book" function from the reader menu, you will
be redirected to the "Return menu” where display a list of all books that you have borrowed
and some options for you to perform tasks such as returning books by ID, checking for the
expired books, searching for books, and sorting books to facilitate your returning process.
The “Return Menu” options include:
1. Return a Book: Initiate the process of returning a book.
2. Check for Expired Books: Verify if there are any books with overdue returns.
3. Find ID Book: Search for a book by its ID.
4. Find Name Book: Search for a book by its name.
5. Find Publisher: Locate books by a specific publisher.
6. Find Authors: Discover books written by specific authors.
7. Find Genre: Explore books based on their genre. lOMoARcPSD| 36625228
8. Find Date Publish: Search for books published on a particular date.
9. Sort ID Book: Arrange the list of books based on their ID.
10. Sort Name Book: Alphabetically sort the list of books by name.
11. Sort Genre: Organize the list of books by genre.
12. Sort Publisher: Sort books based on their publisher.
13. Sort Authors: Arrange books based on the authors.
14. Sort Date Publish: Sort books based on their date of publication.
15. Back to Menu Page: Return to the main menu for additional actions.
Figure 3.7: The return menu interface. C. Conclusion 1. Conclusion
The project was successfully completed, meeting the needs of users. Specifically,
we developed the following additional features:
• Search by conditions: This feature allows users to easily search for books
information by criteria such as book ID, Title, Author, etc.
• Sort book, reader by ID, by name or by Title, etc: This feature allows users to easily
manage book reader by ID, by name or by Title, etc.
• Borrow/Return book easy: This feature allows users to easily borrow/return from
library and store to .txt file. Strengths
• The interface is concise, intuitive, easy to access and use: This helps users to use the
program easily even if they do not have much experience in information technology.
• The program runs stably, provides accurate results, without errors or lag: This
ensures the best experience for users.
• The code uses the algorithms we have learned: This demonstrates the team's ability
to learn and apply knowledge. Weaknesses
• The search feature does not have many options: We can add more search options
such as by popular, by republish, etc. lOMoARcPSD| 36625228
• The sorting of lists is still limited: We can add more sorting options such as in
ascending order, descending order, or alphabetical order.
• The program is not fully optimized due to processing a lot of information: We can
continue to optimize the program to reduce processing time and increase data retrieval speed.
2. Development directions
Optimize data and program processing: This will help programs run faster and more efficiently.
Add features to fine for returning books late, lock account, print list of books by
popularity: This will meet user needs.
Complete, user-friendly interface design: This will help users use the program more easily.