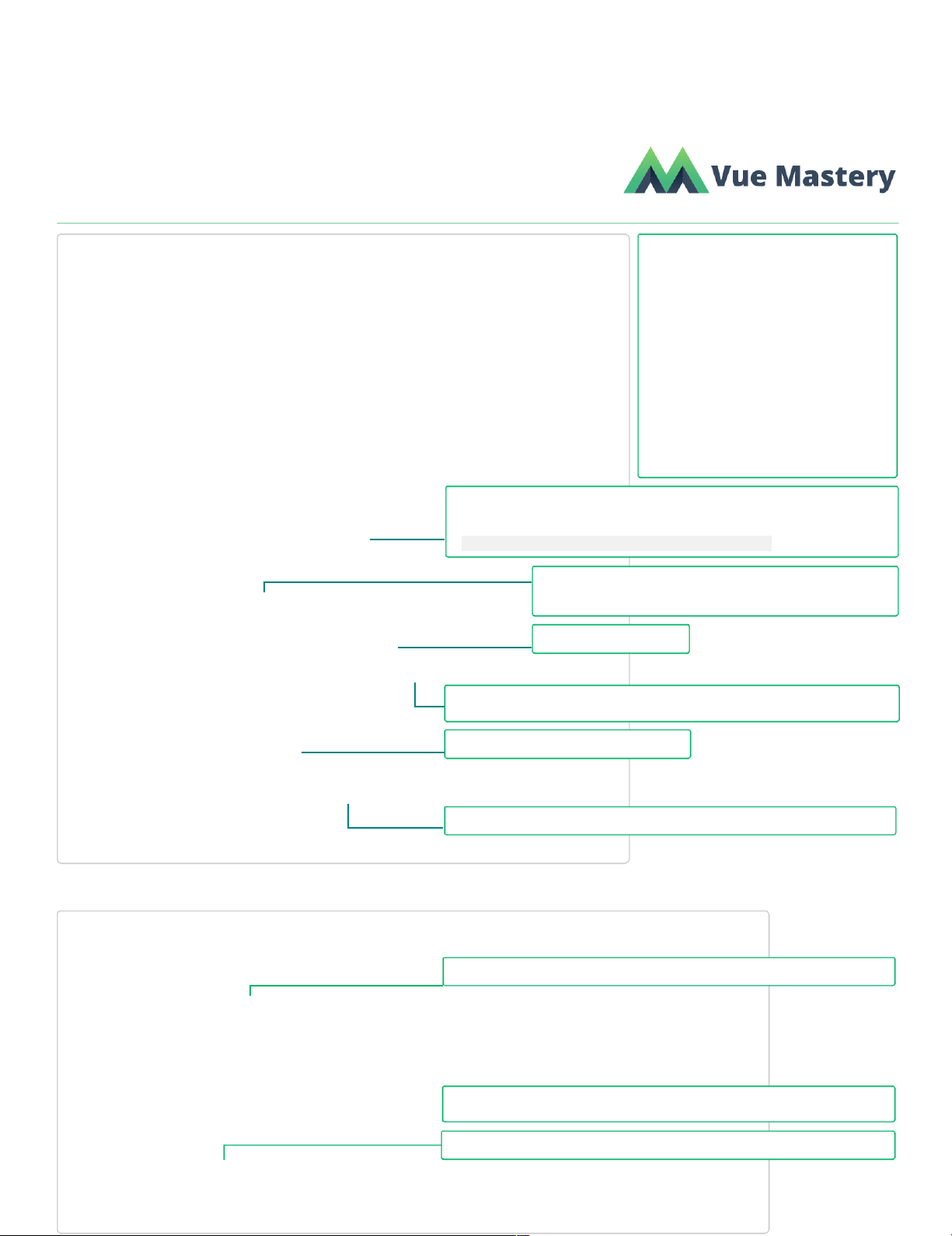
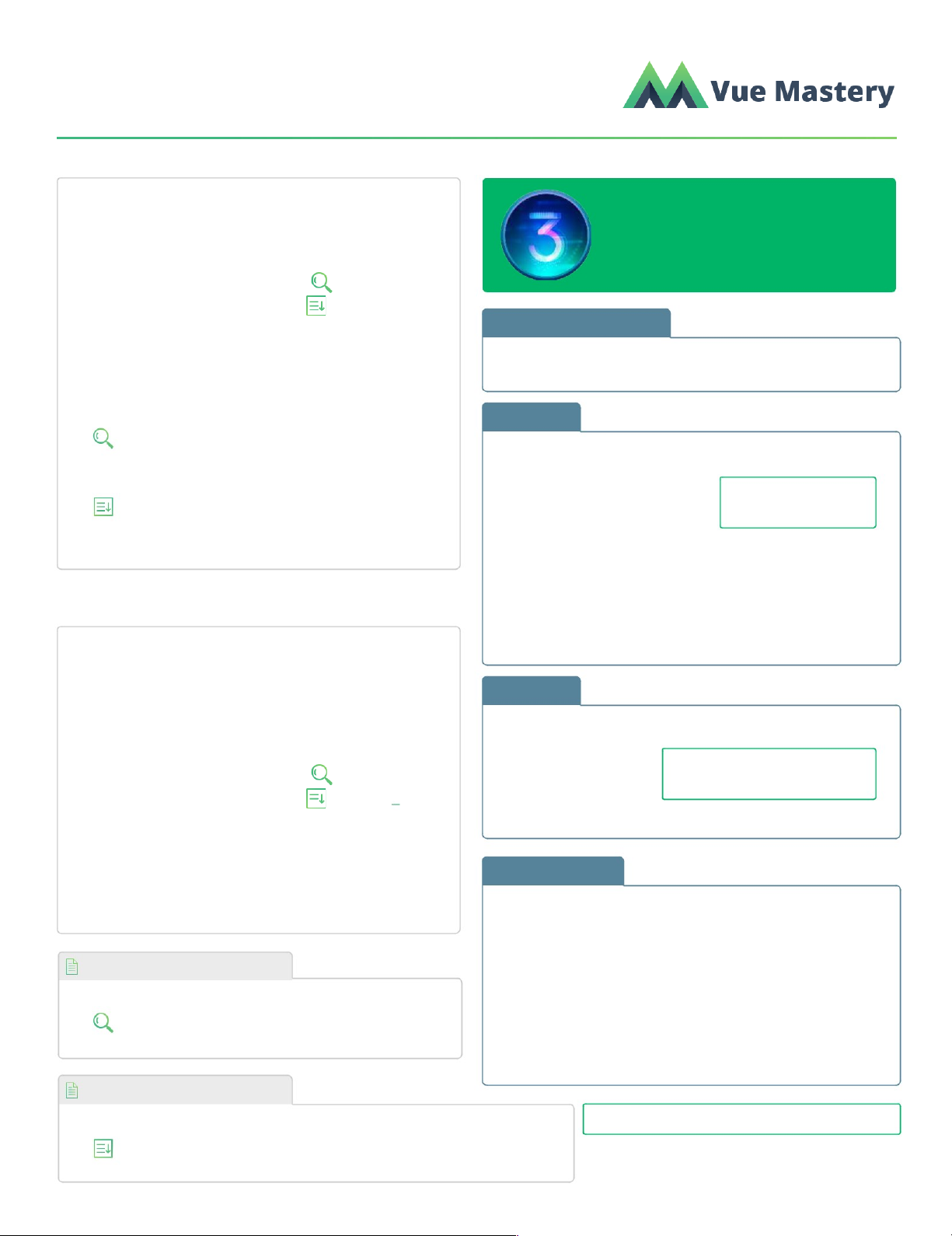
Preview text:
lOMoARcPSD|46958826 lOMoARcPSD|46958826 VUE 3 COMPOSITION API CHEAT SHEET
Use the composition API when:
The component is too large, and
Spaces Left: {{ spacesLeft }} out of {{ capacity }}
should be organized by logical Attending concerns(feature).AND / OR
v-for="(name, index) in attending" :key="index">
Code needs to be extracted and reused {{ name }}
across mulitiple components, as an
alternative to Mixins/Scoped Slots. AND / OR
click="increaseCapacity()">Increase Capacity
Type safety in TypeScript is important.
If using Vue 2 with Composition API plugin configured:
import { ref, computed } from "vue";
import { ref, computed } from "@vue/composition-api"; export default { setup() { Reactive Reference
const capacity = ref(4);
Wraps primitives in an object to track changes
const attending = ref(["Tim", "Bob", "Joe"]);
const spacesLeft = computed(() => { Computed Property
return capacity.value - attending.value.length; });
Access the value of a Reactive Reference by cal ing .value
function increaseCapacity() {
capacity.value++; }
Methods declared as functions
return { capacity, attending, spacesLeft, increaseCapacity }; } };
Gives our template access to these objects & functions CAN ALSO BE WRITTEN AS:
import { reactive, computed, toRefs } from "vue"; export default { setup() {
Reactive takes an object and returns a reactive object const
event = reactive({
capacity: 4,
attending: ["Tim", "Bob", "Joe"],
spacesLeft: computed(() => { return event.capacity - event.attending.length; }) });
function increaseCapacity() {
Notice we don’t have to use .value since the object is reactive
event.capacity++; }
toRefs creates a plain object with reactive references
return { ...toRefs(event), increaseCapacity }; } }; Watch the Vue 3 E ssentials course on Vu eMastery.com lOMoARcPSD|46958826 VUE 3 COMPOSITION API CHEAT SHEET TO ORGANIZE BY FEATURE: … Watch the Vue 3 Essentials
course at VueMastery.com, taught export default { by Gregg Pollack. setup() {
const productSearch = useSearch( )
const resultSorting = useSorting({ }) The setup() method
return { productSearch, resultSorting }
Cal ed after beforeCreate hook and before created hook. } Does not have access to this. }
function useSearch(getResults) { props
The first optional argument of setup: export default { } props: {
function useSorting({ input, options }) { name: String Props are reactive }, and can be watched } setup(props) { watch(() => {
console.log(`name is: ` + props.name) TO EXTRACT SHARED CODE: }) } … }
import useSearch from '@use/search' import context
The second optional argument of setup:
useSorting from '@use/sorting' export default {
setup(props, context) { setup() { context.attrs;
const productSearch = useSearch( ) context.slots; Exposes properties previously
accessed using this
const resultSorting = useSorting({ }) context.emit; }
return { productSearch, resultSorting } } life-cycle hooks Declare them inside setup } setup() {
onMounted(() => { ... });
onUpdated(() => { ... }); use/search.js
onUnmounted(() => { ... });
export default function useSearch(getResults) { } }
Instead of using beforeCreate or created hooks, just write code
or call functions inside setup() instead. use/sorting.js
export default function useSorting({ input, options }) {
See the API documentation for additional info. }