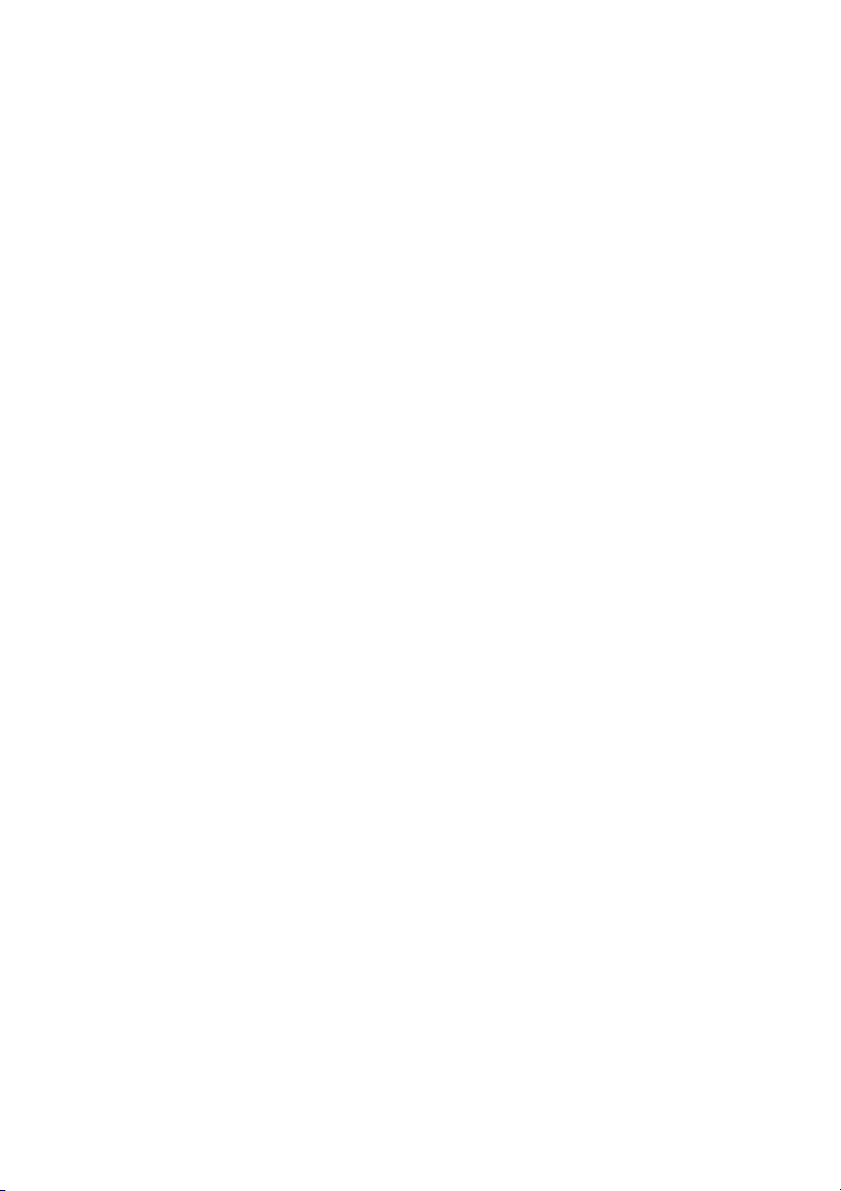
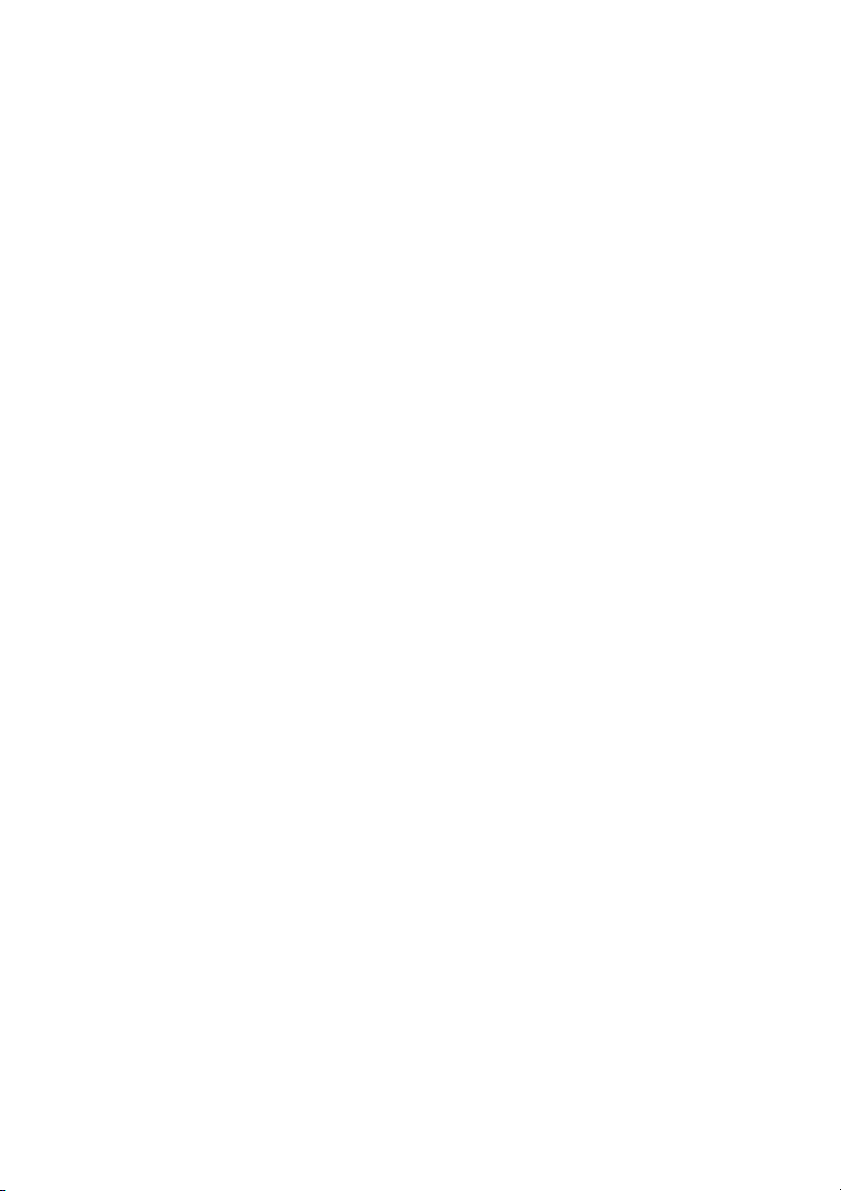
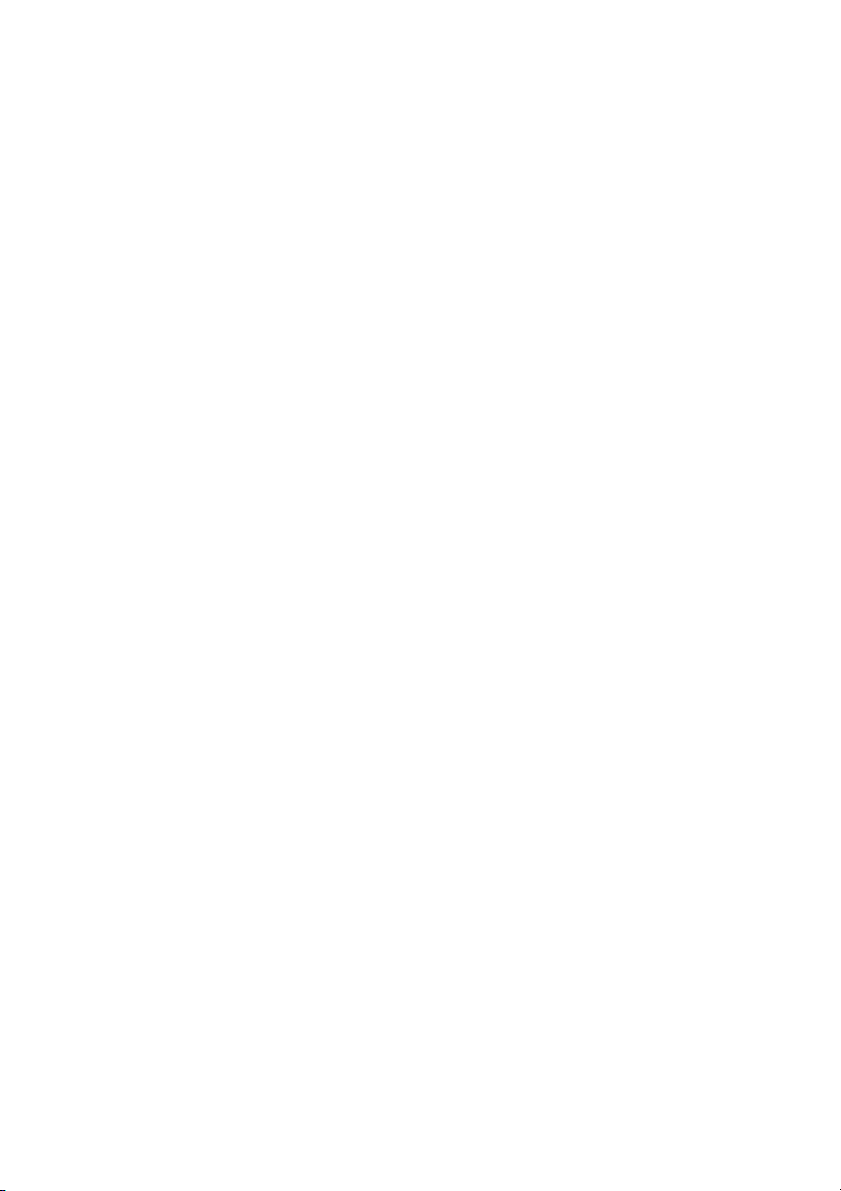
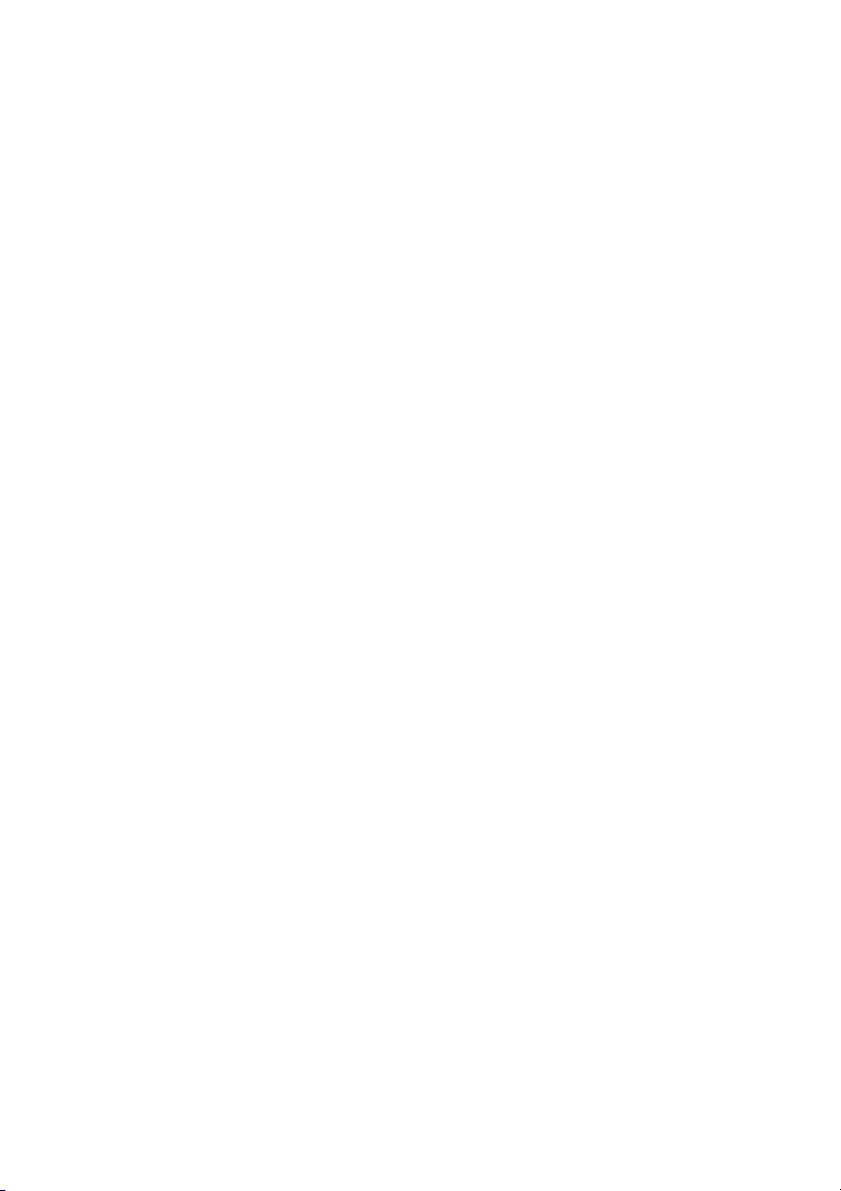
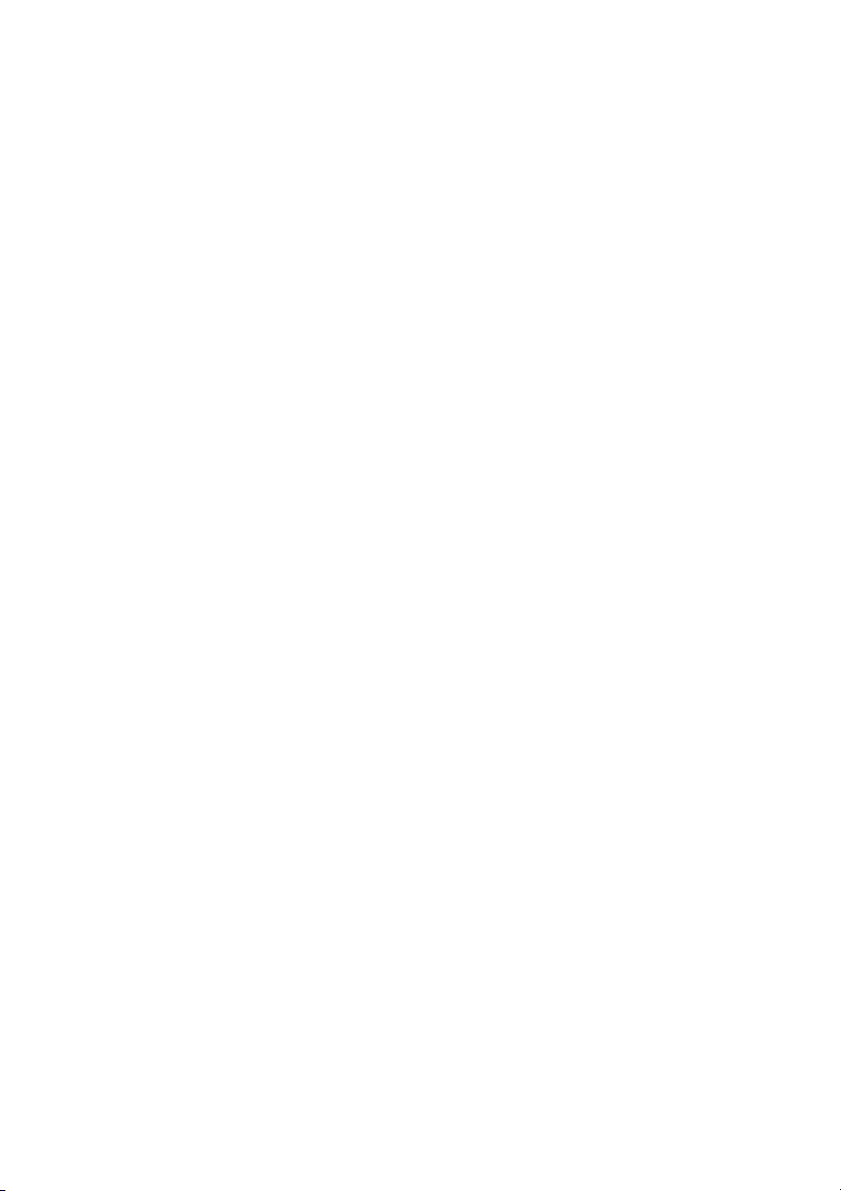
Preview text:
Practice Exercises - Chapter: 02 * Exercise 2.1:
Write a program that prompts a user for two integers num1, num2 and print out the sum of them. >#include int main(){ int a,b;
printf("Nhap vao 2 so nguyen a va b\n"); printf("So nguyen a la: "); scanf("%d",&a); printf("So nguyen b la: "); scanf("%d", &b);
printf("Tong cua 2 so nguyen a va b la: %d", a+b); return 0; * Exercise 2.2: Restaurant Bill
Write a program that computes the tax and tip on a restaurant bill for a patron with a
$88.67 meal cost. The tax is 6.75 percent of the meal cost and the tip is 20 percent of the
meal cost after adding the tax. Display the total cost, tax amount, tip amount, and total bill on the screen. >#include int main(){ float fee,tax,tip; fee=88.67; tax=6.75;
tip=(fee+(fee*tax/100)*20/100); printf("=====Bill===== \n");
printf("Total cost: %.2f\n", fee+(fee*tax/100)+tip);
printf("Meal costs: %.2f\n", fee); printf("Tax: %.2f\n", tax); printf("Tip: %.2f", tip); getchar(); Solution 2.2: * Exercise 2.3: Future Ocean Levels
During the past decade ocean levels have been rising faster than in the past, an average of
approximately 3.1 millimeters per year. Write a program that computes the ocean levels
expected to rise in the next 20 years if they continue rising at this rate. Display the result
in both centimeters and inches measures. Solution 2.3: >#include int main(){
printf("Sea levels are expected to rise over the next 20 years if they continue to rise at this rate: \n");
printf("%f cm\n",(3.1*20)/10);
printf("%f inch",(3.1*20)/10*0.39370078740157); return 0; } * Exercise 2.4:
Write a program to swap values of two variables. >#include int main(){ double a,b,c;
printf("Nhap vao 2 so a va b: \n"); printf("Nhap vao a: "); scanf("%lf",&a); printf("Nhap vao b: "); scanf("%lf",&b); c=a; a=b; b=c;
printf("Gia tri 2 so sau khi hoan doi la: \n"); printf("a: %lf\n",a); printf("b: %lf", b); getchar(); return 0; } * Exercise 2.5:
Write a program that prompts a user for two integers and performs all arithmetic
operations (addition, subtraction, multiplication and division). >#include int main(){ int a,b;
printf("Nhap vao 2 so nguyen\n"); printf("Nhap vao a: "); scanf("%d",&a); printf("Nhap vao b: "); scanf("%d",&b); printf("a + b = %d\n", a+b);
printf("a - b = %d\n", a-b); printf("a * b = %d\n", a*b);
printf("a / b = %f", (float)a/b); return 0; * Exercise 2.6:
Write a program that prompts a user for the radius of a circle and computes the perimeter and the area of the circle. #include int main(){ float a; const float pi=3.14;
printf("Nhap vao ban kinh hinh tron: "); scanf("%f", &a);
printf("Chu vi hinh tron la: %.2f\n", (float)2*pi*a);
printf("Dien tich hinh tron la: %.2f", (float)pi*a*a); return 0; } * Exercise 2.7:
Write a program that prompts a user for height and width of a rectangle and then
computes the perimeter and the area of the rectangle. >#include int main(){ float a,b;
printf("Nhap chieu dai va rong cua hinh chu nhat:\n");
printf("Nhap chieu dai: "); scanf("%f",&a);
printf("Nhap chieu rong: "); scanf("%f", &b);
printf("Chu vi hinh chu nhat la: %.2f\n",(float)(a+b)*2);
printf("Dien tich hinh chu nhat la: %.2f", (float)a*b); getchar(); return 0; } * Exercise 2.8:
Write a program that prompts a user for an integer and computes the weeks and days from the entered number. For example: Number of days: 1329 Expected output Weeks: 189 Days: 6 >#include int main(){ int a; printf("Nhap vao a: "); scanf("%d", &a);
printf("Number of days: %d\n",a); printf("Expected output\n"); printf("Weeks: %d\n",a/7); printf("Days: %d",a%7); getchar(); return 0; } * Exercise 2.9:
Write a program that prompts a user for two angles of a triangle and computes the third one. For example: Sample Input 50 70 Sample Output 60 >#include int main(){ float a,b;
printf("Nhap vao lan luot 2 goc cua tam giac: "); scanf("%f%f",&a,&b);
printf("2 goc da cho: %.2f va %.2f\n",a,b);
printf("goc con lai la: %.2f ", (float)180-a-b); getchar(); return 0; } * Exercise 2.10:
Write a program that prompts a user for hours and minutes and then calculates the
number of minutes from the entered values. For example: Sample Input 5 37 Sample Output 337 >#include int main(){ int a,b; printf("Nhap vao so gio: "); scanf("%d",&a); printf("Nhap vao so phut: "); scanf("%d",&b);
printf("Thoi gian da nhap: %d gio %d phut\n",a,b);
printf("So phut: %d", a*60+b); getchar(); return 0; }