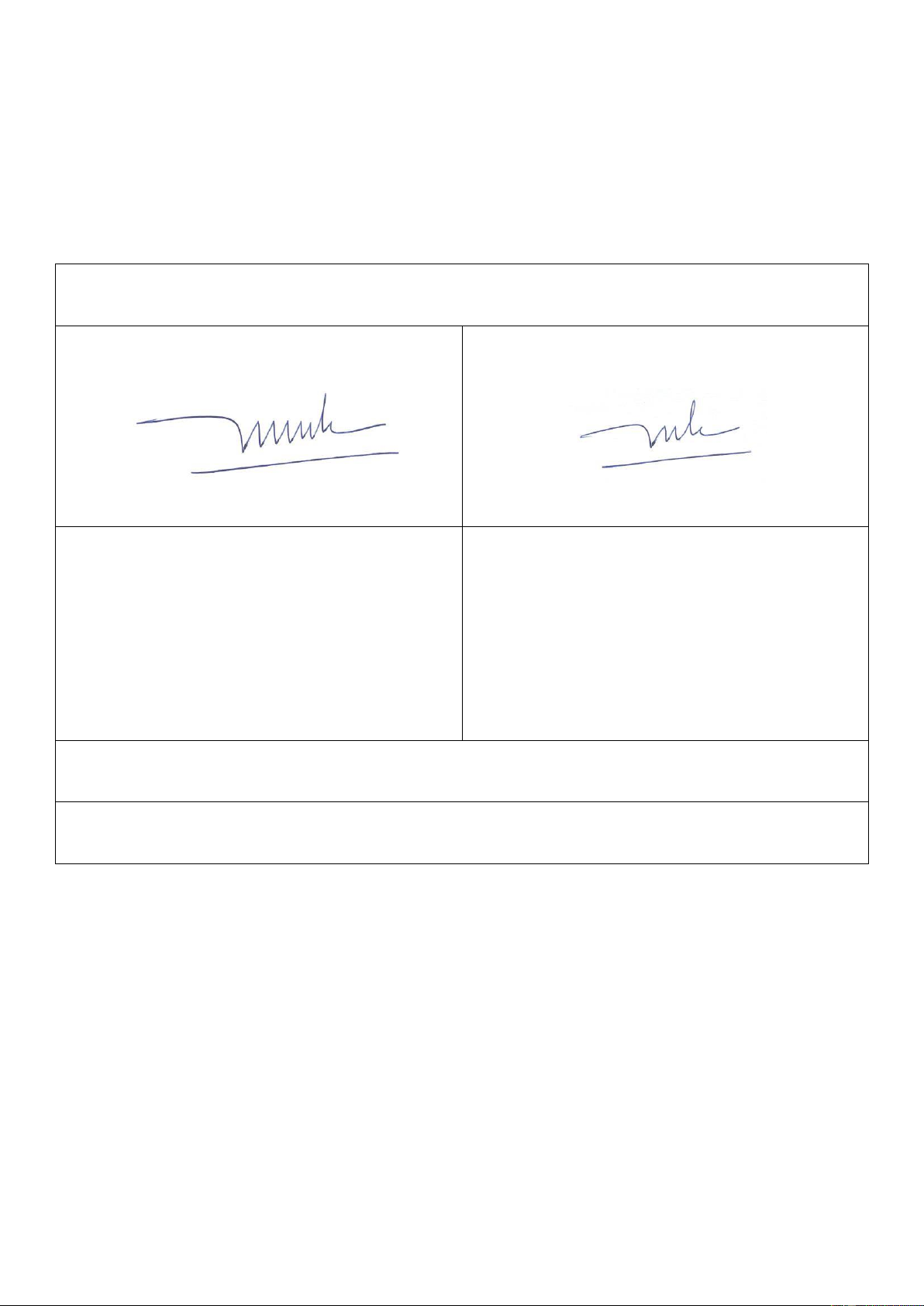
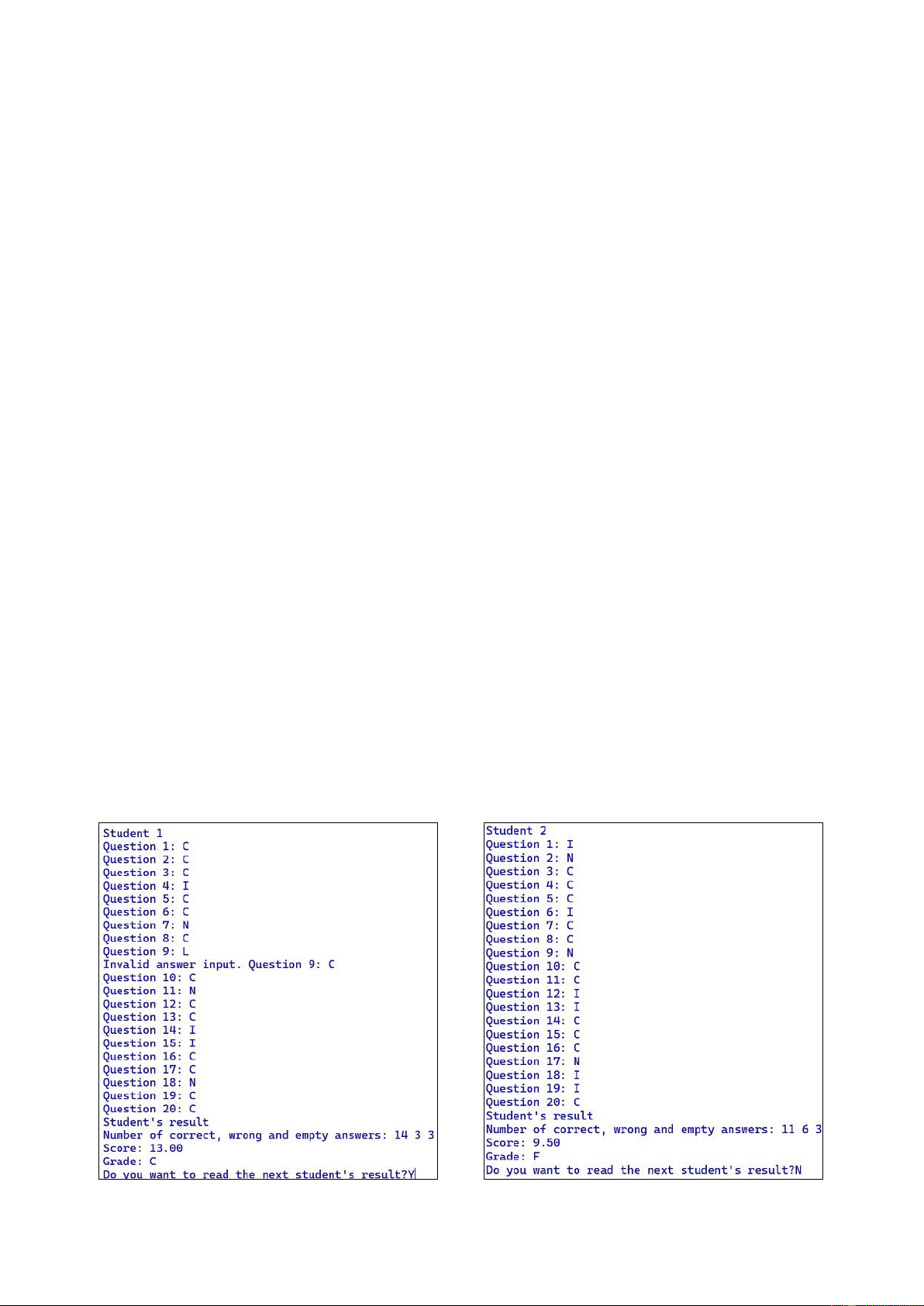
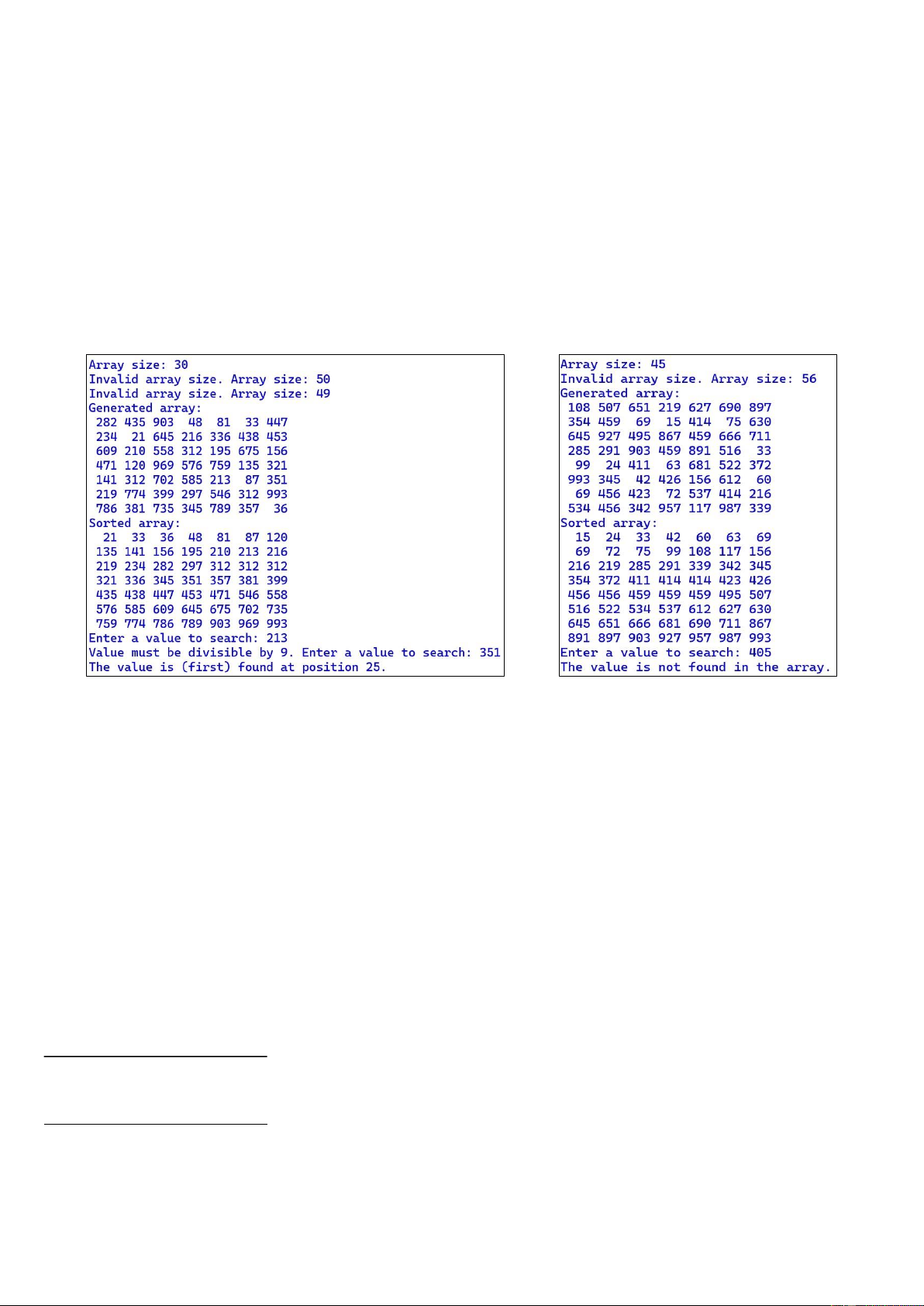
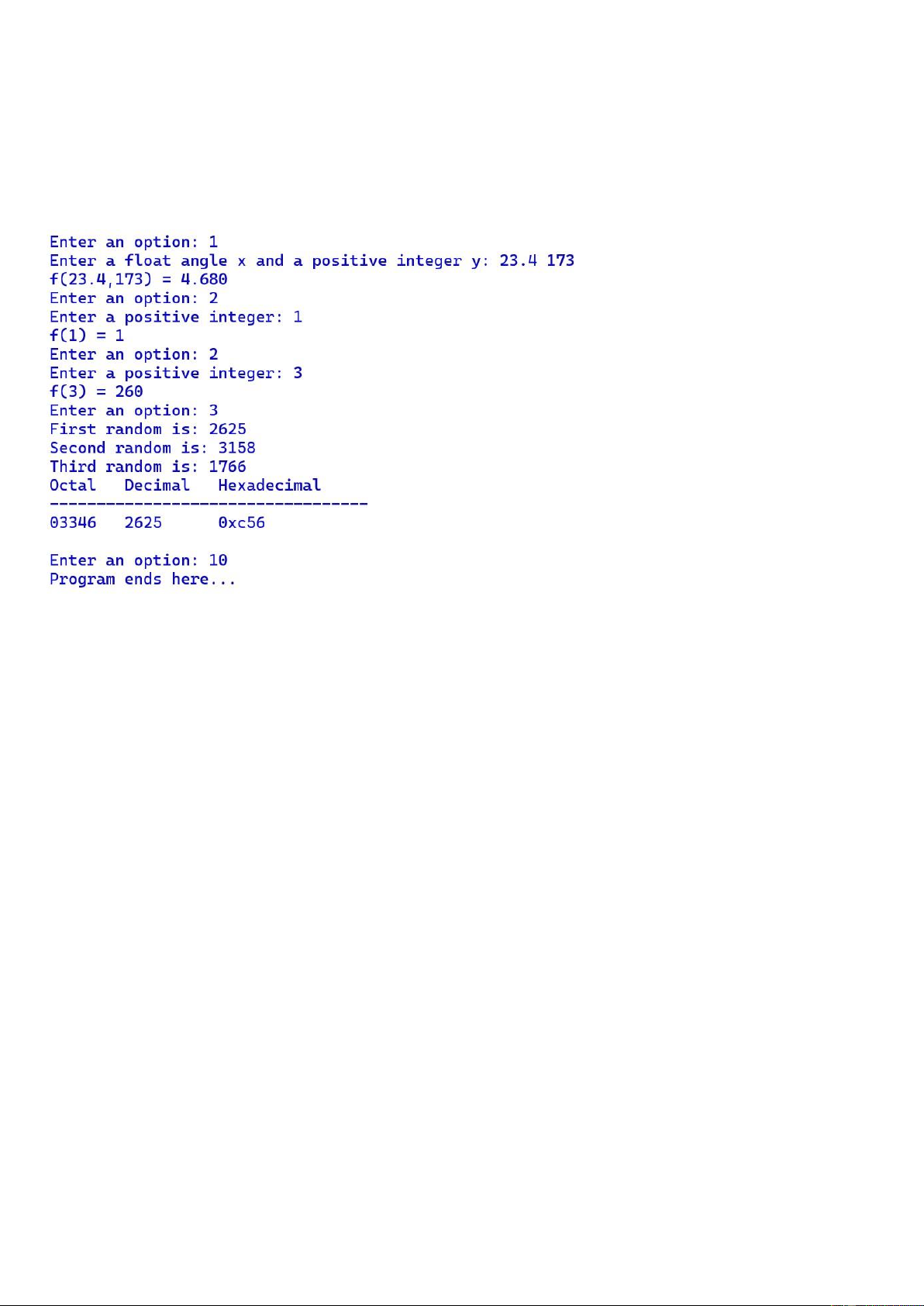
Preview text:
International University - VNUHCM
School of Electrical Engineering -------------------- Midterm Examination
Date: 23/04/2024; Duration: 90 minutes (08:00 – 09:30)
Closed book; Offline; Electronic Devices are not allowed.
SUBJECT: Programming for Engineers (ID: EE057IU)
Approval by the school of Electrical Engineering Lecturer: Signature Signature
Full name: Dr. Nguyen Ngoc Truong Minh
Full name: Dr. Nguyen Ngoc Truong Minh Proctor 1 Proctor 2 Signature Signature Full name: Full name: STUDENT INFO
Student name: _________________________
Student ID: _______________
INSTRUCTIONS: the total of point is 100 (equivalent to 30% of the course) 1. Purposes:
• Test your knowledge in the basic principles of computer programming (CLO1)
• Examine your skill in analysis and design solutions to programming problems (CLO2)
• Test your knowledge, skill in applying programming constructs and problem-solving principles
to the development of C programs (CLO3) 2. Requirements:
• Carefully read each question and answer it following the requirements
• Write the answers CLEARLY using DevC++
• Your source code will be collected by the lecturer. However, the students are also requested
to submit your exam on Blackboard (Assignment rubric).
HCMC Vietnam National University
Student Name: ………………………………… International University
Student ID: ………………… ------------
Total pages: 04 (including this page) QUESTIONS
Q1. (35 marks) Making Decision, Looping, I/O Operations
Write a C program that helps to evaluate the score of a quiz of a student. The quiz has 20
multiplechoice questions with 03 available valid answers:
• Answer = ‘C’ (Correct): the score plus 1 point
• Answer = ‘I’ (Incorrect): the score minus 0.25 points
• Answer = ‘N’ (No Answer): the score remains the same
• Answer = any other character requires to read the answer again
Note that the answer is given in the uppercase only. The score of the student should be rounded with the following rules:
• If the decimal of the score ≥ 0.75: round up
• If the decimal of the score = 0.5: remains the same • Otherwise, round down
Print the grade of the student based on the score as:
• If the score is from 18.0 to 20.0: grade ‘A’
• If the score if from 15.0 to 17.5: grade ‘B’
• If the score if from 12.5 to 15.0: grade ‘C’
• If the score if from 10.0 to 12.5: grade ‘D’ • Otherwise, grade ‘F’
At the end, the program will ask to read the next student’s quiz (‘Y’) or not (‘N’). Do not use Array and Function.
Output example:
Q2. (30 marks) Array, Sorting, Searching and Function. Must define functions. /4 2
HCMC Vietnam National University
Student Name: ………………………………… International University
Student ID: ………………… ------------ Write a C program that:
- creates an integer array with M elements (M is read from the user, must be at least 40 and divisible by 7)
- generates array elements to random numbers with two or three digits and divisible by 3 (i.e., 12, 15, 120, 996)
- prints the generated array with 7 values per line and 4 spaces for each value
- sorts the original array in ascending order, prints the sorted array
- reads an integer value (divisible by 9 and must check the condition)
- searches if this value is found in the given array or not using binary search Output example:
Q3. (35 marks) Making Decision, Looping and Function
Write a C function that accepts from the main program a float value x (angle in degree) and a positive
integer value y. The function will calculate and print the result (3 decimal-point format, i.e., 1.234)
from the following equation on the screen (do not return to the main program):
𝑓(𝑥, 𝑦) = ln(𝑦) − 3sin2(𝑥)
Write a recursive function that accepts from the main program a positive integer n and returns the
result of the following equation to the main program:
𝑓(𝑛) = 13 + 24 + 35 + ⋯ + 𝑛𝑛+2
Write a C function that generates randomly inside the function three 4-digit values. The function
should find min, middle and max value among these three. Print a summary table as follow1: Min Middle Max
1 Column 1 (Min printed in Octal base with 8 spaces); Column 2 (Middle printed in Decimal base with 10 spaces); Column 3 (Max printed
in Hexadecimal base with 16 spaces); All aligned to the left of the column. /4 3
HCMC Vietnam National University
Student Name: ………………………………… International University
Student ID: ………………… ------------
----------------------------------------------------------------------------- Octal_base Decimal_base Hexadecimal_base
Then, develop a C program that reads an option (unsigned value) to call the three functions above.
The program should repeat when option value is 1, 2 or 3, exit while option is greater than 3. Output example: – END – /4 4