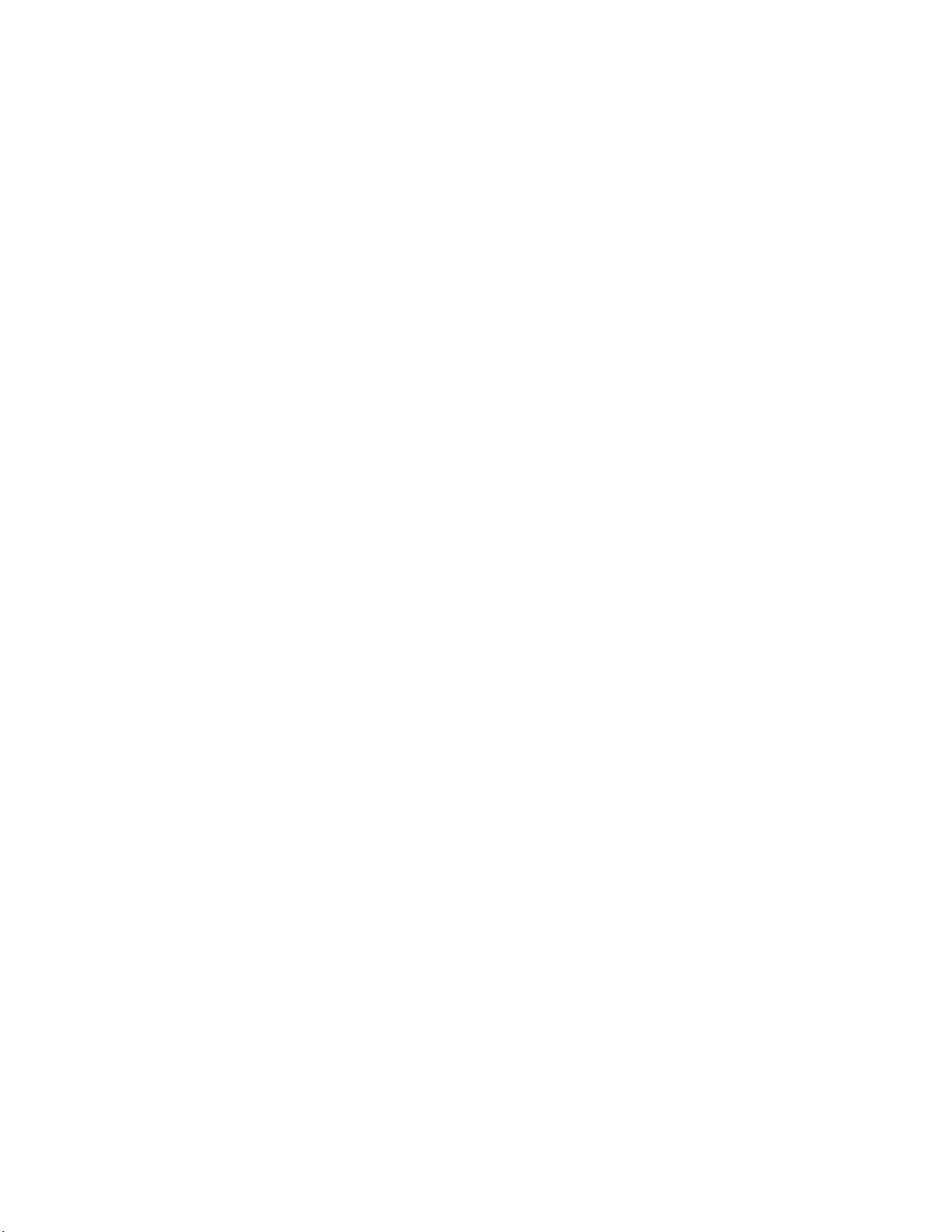
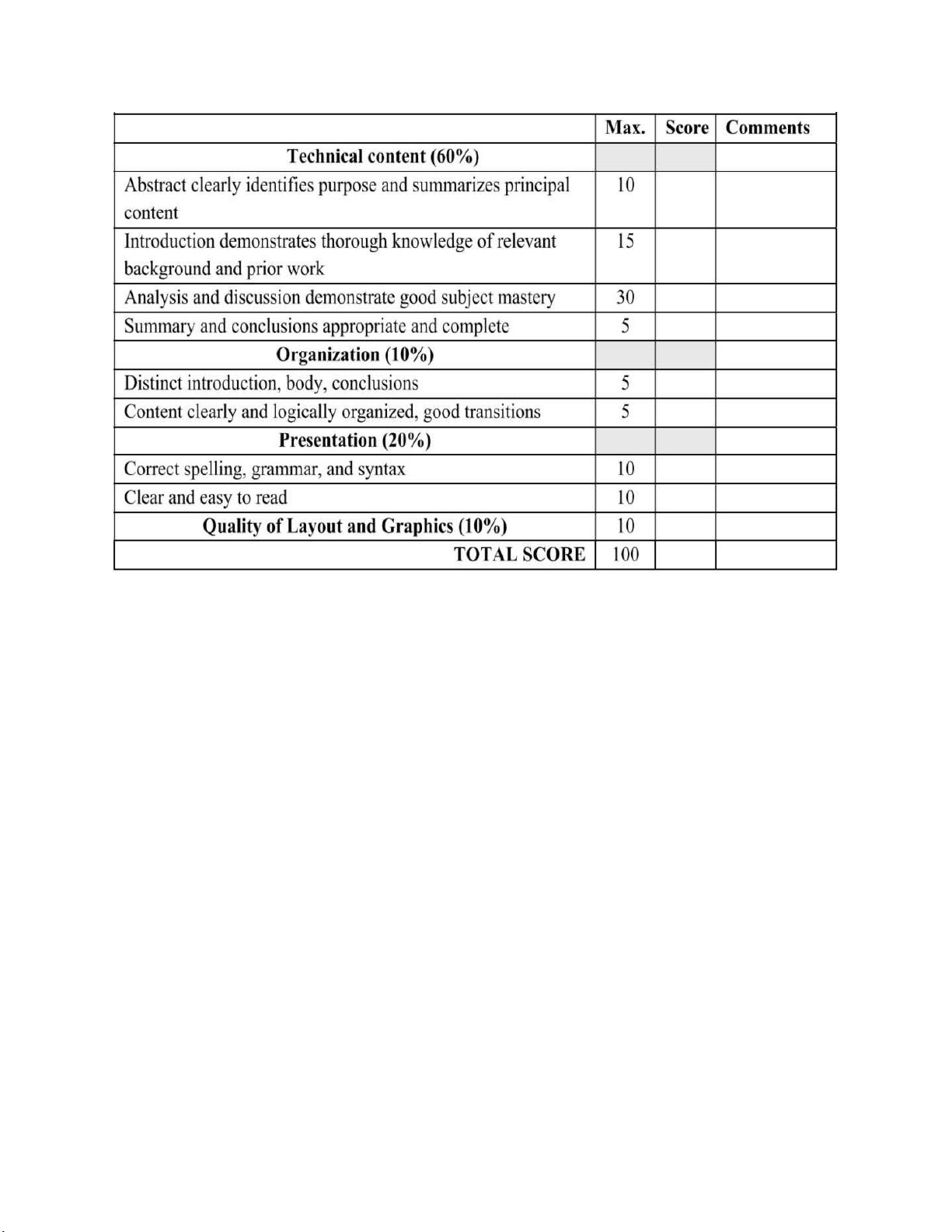
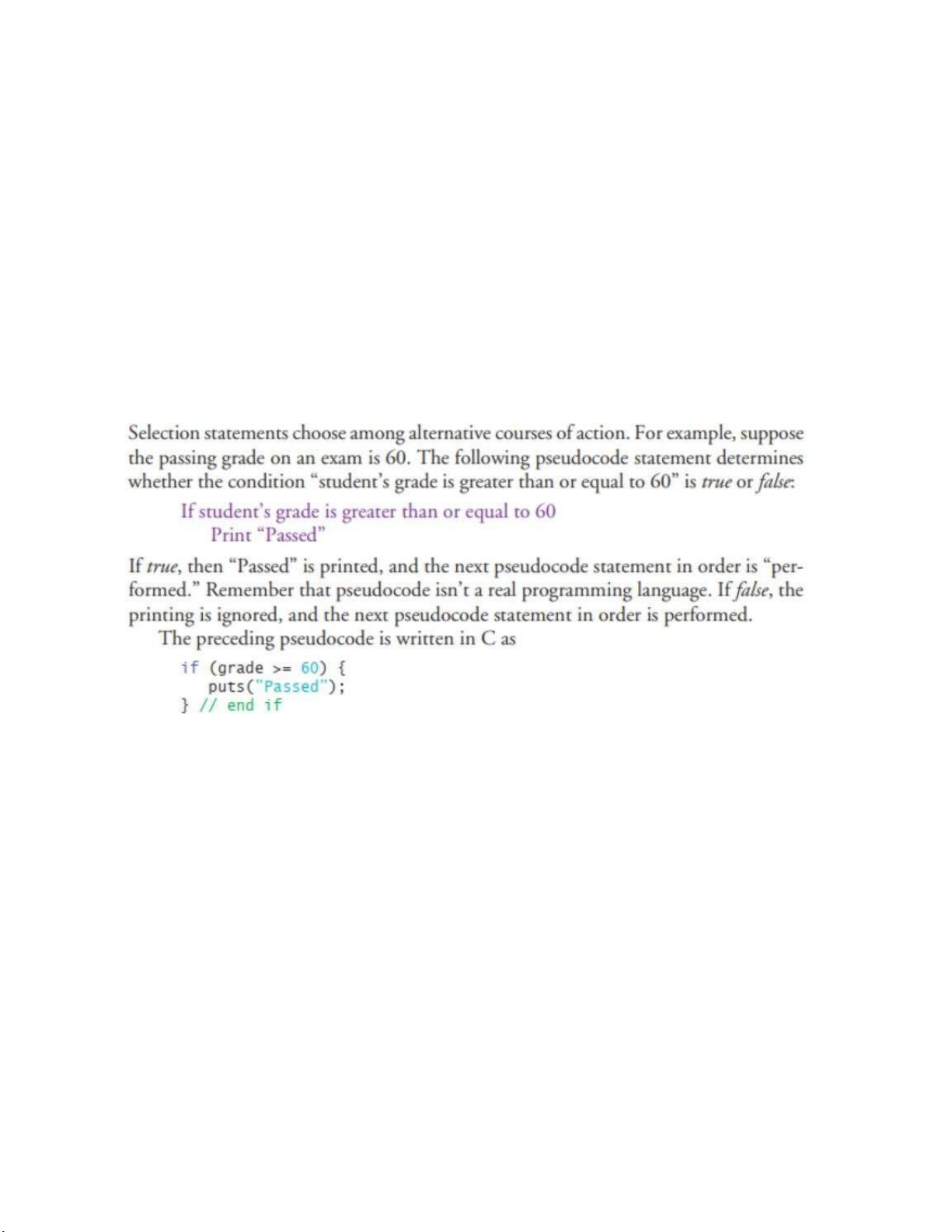
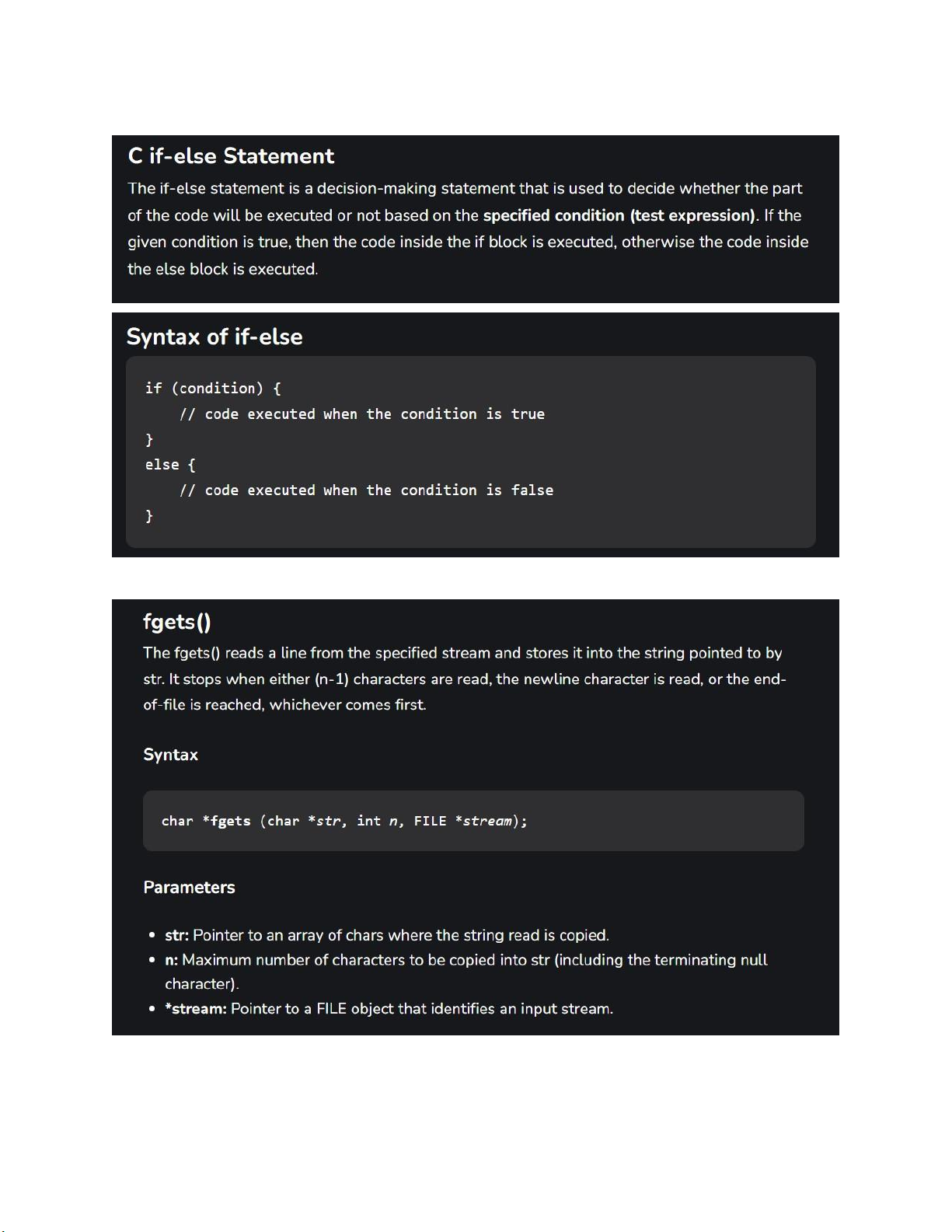
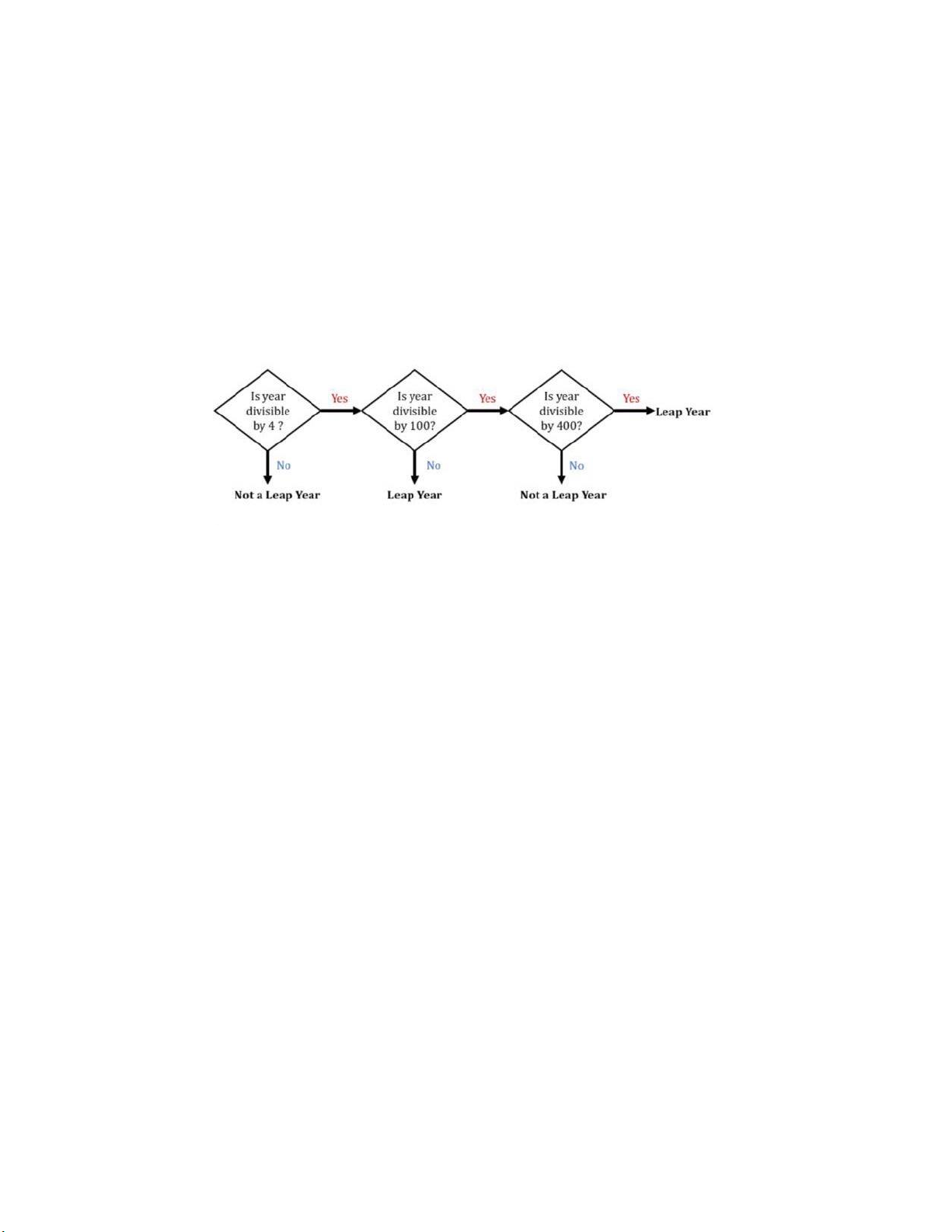
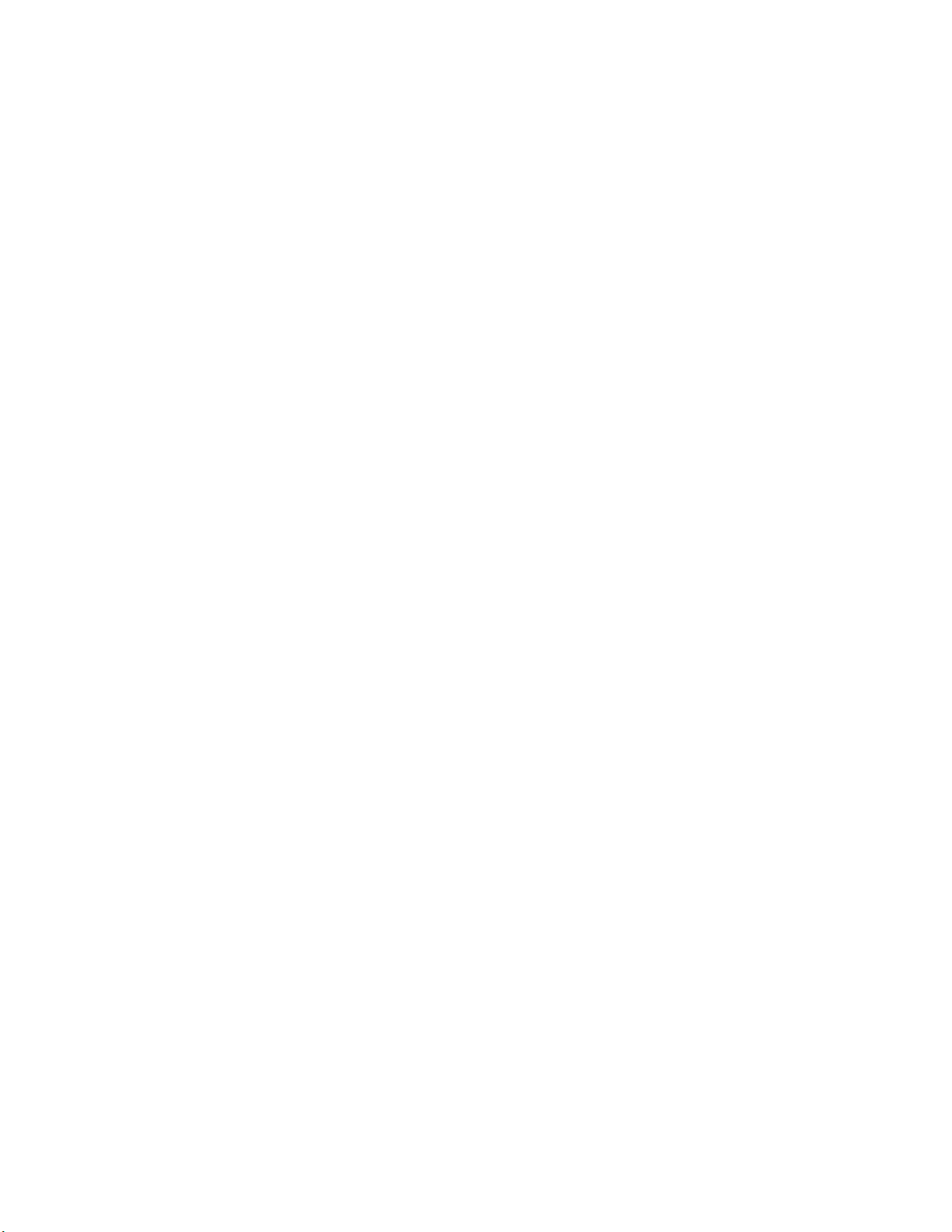
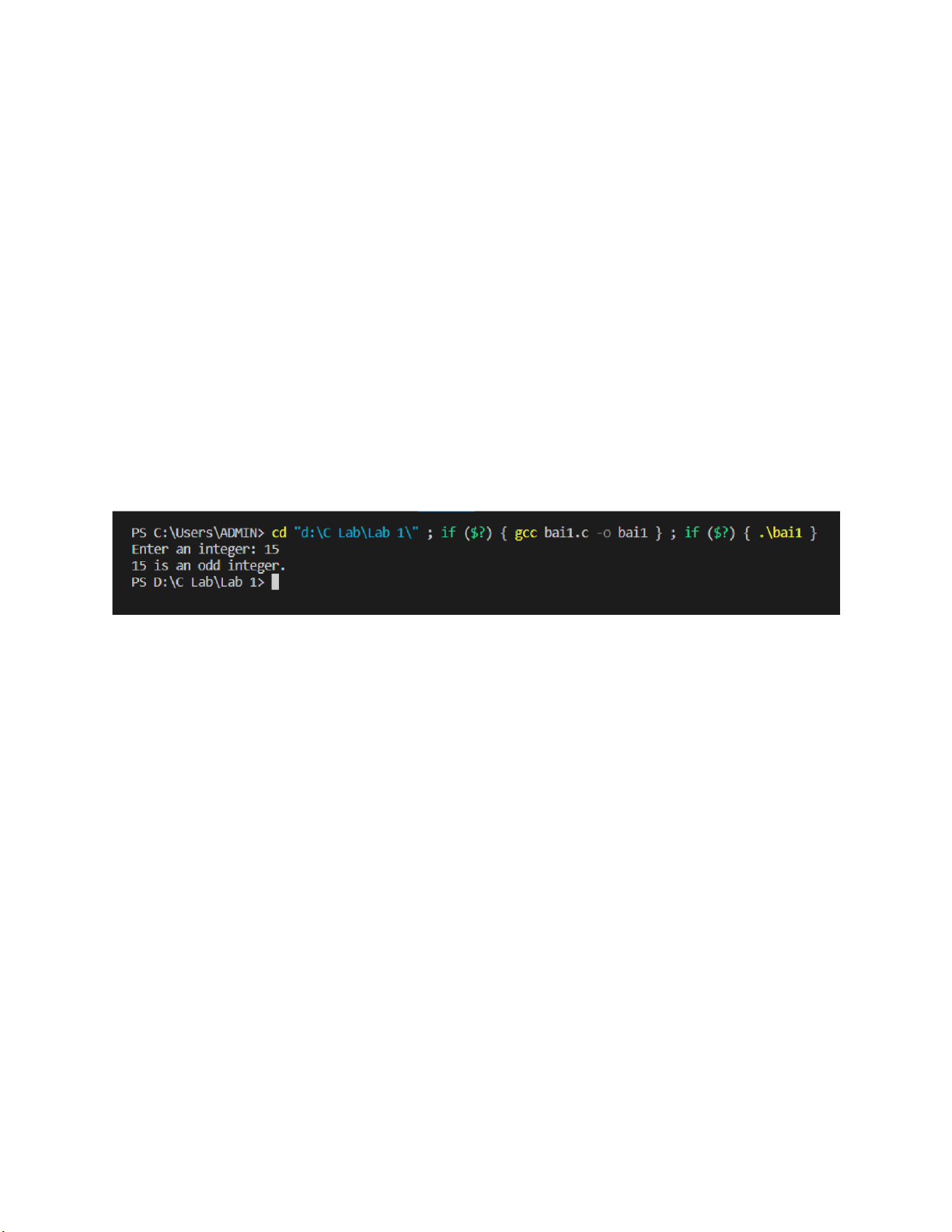
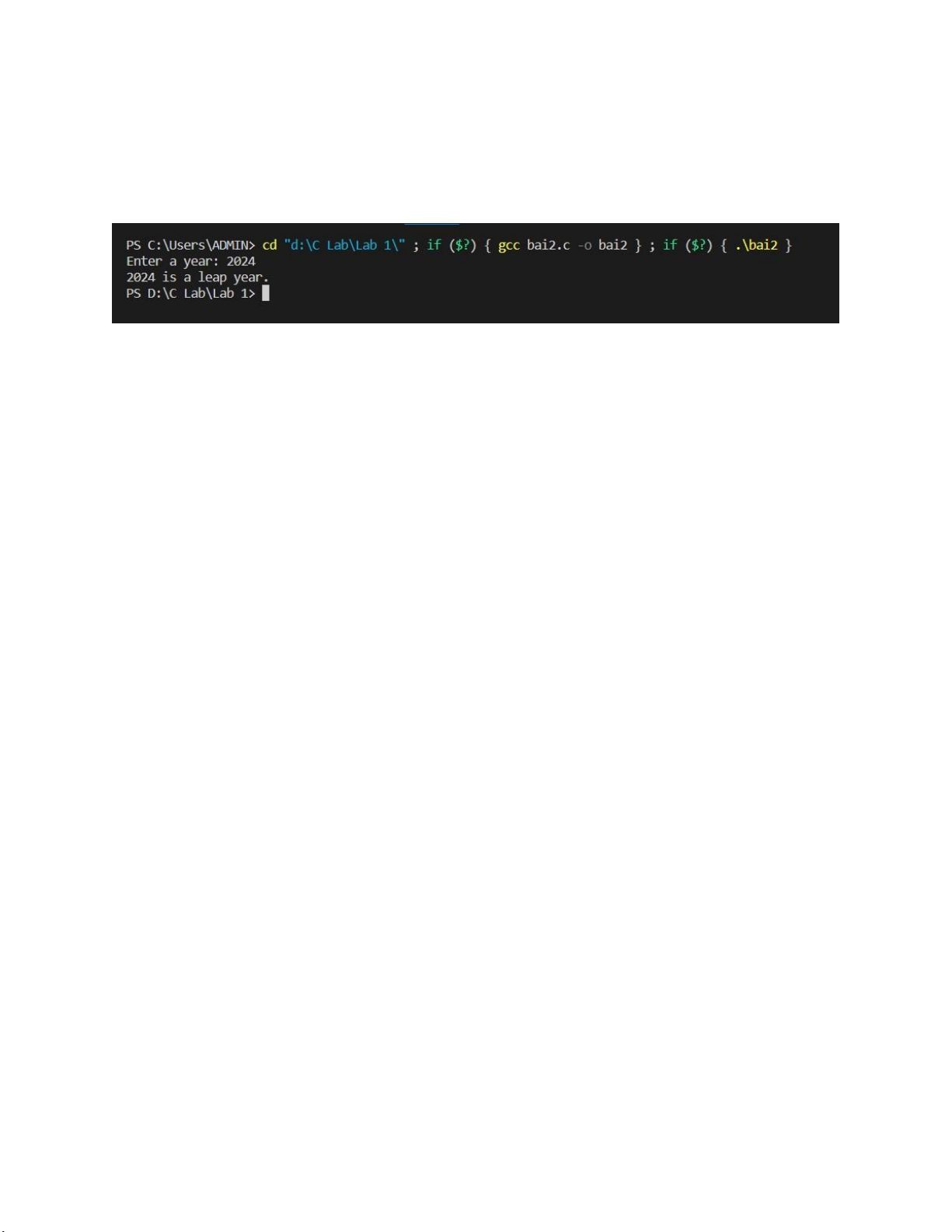
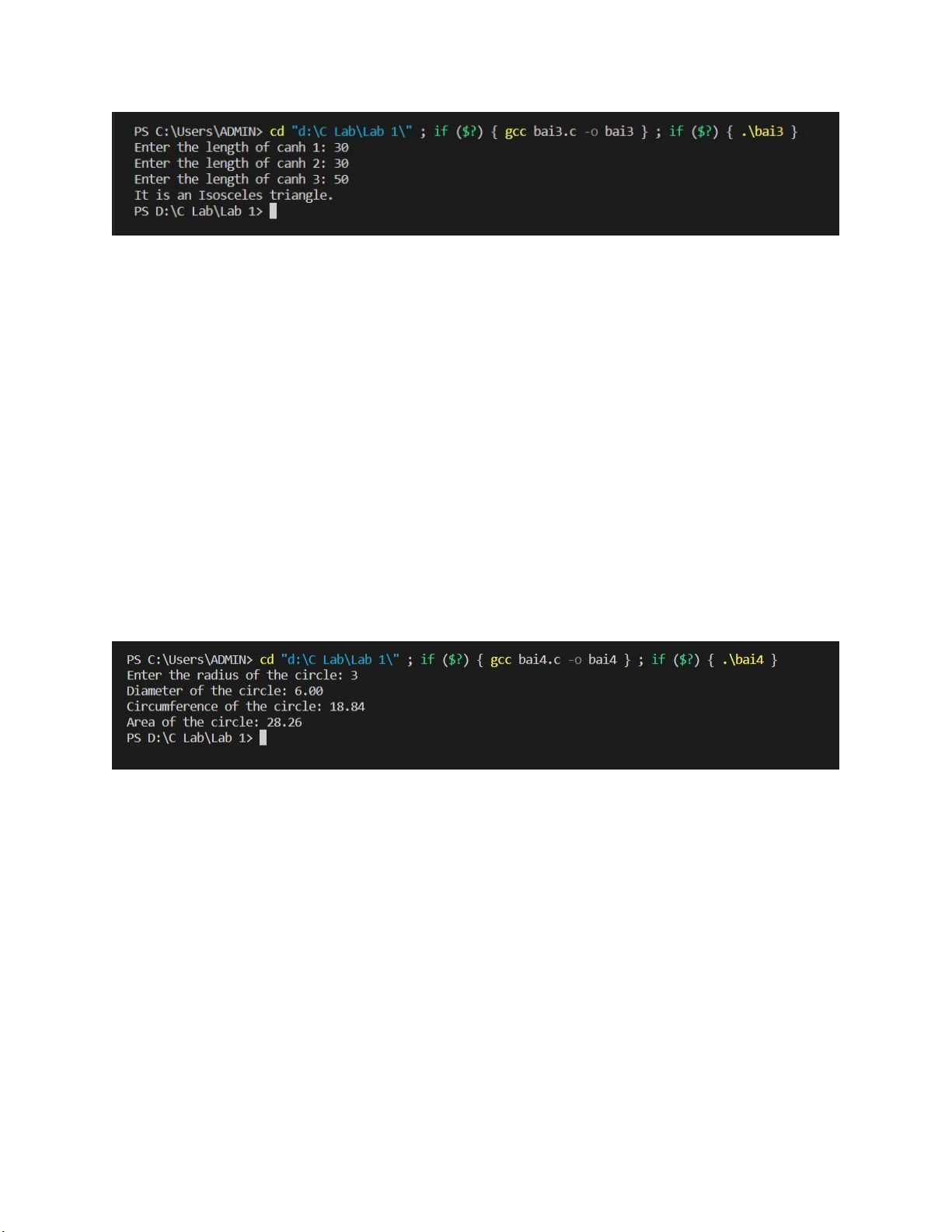
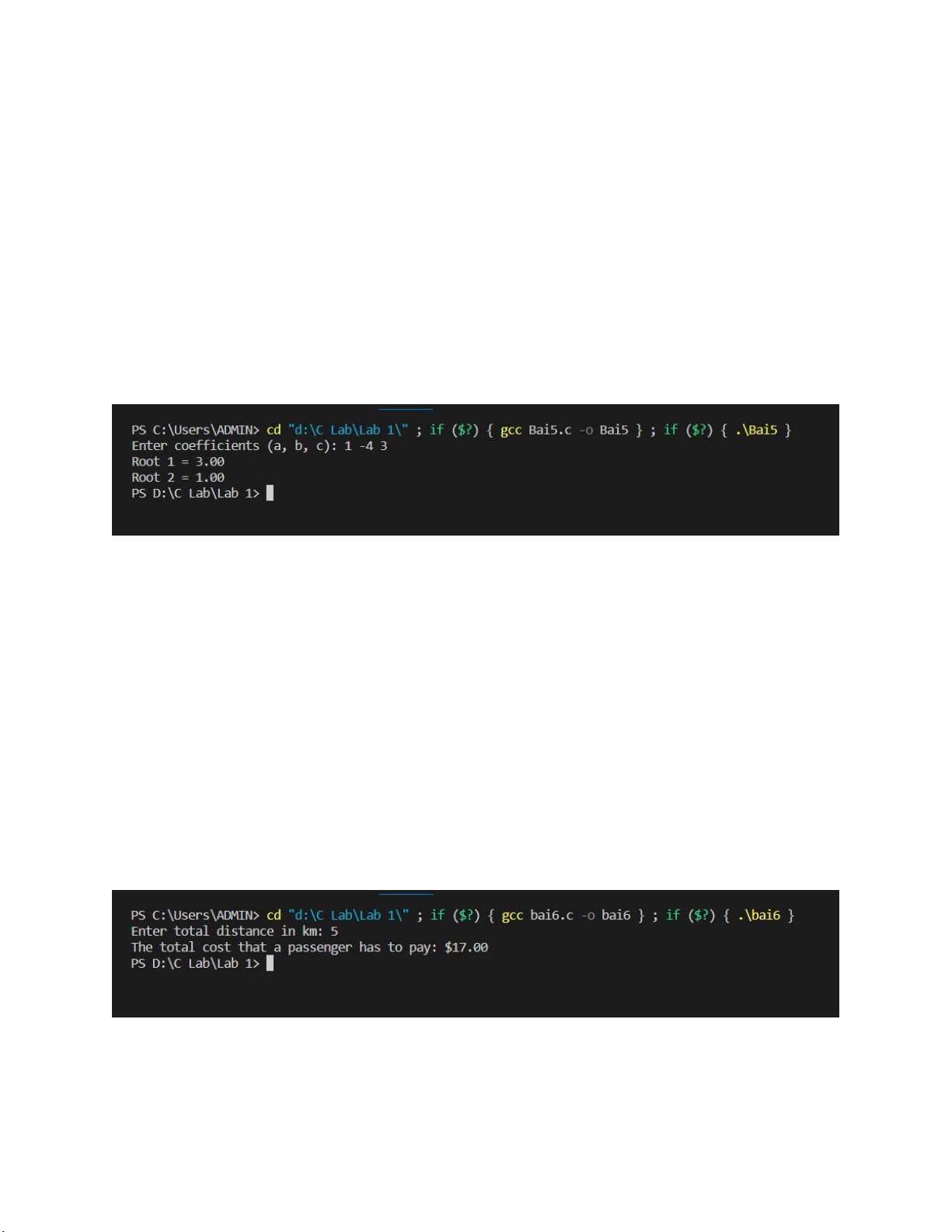
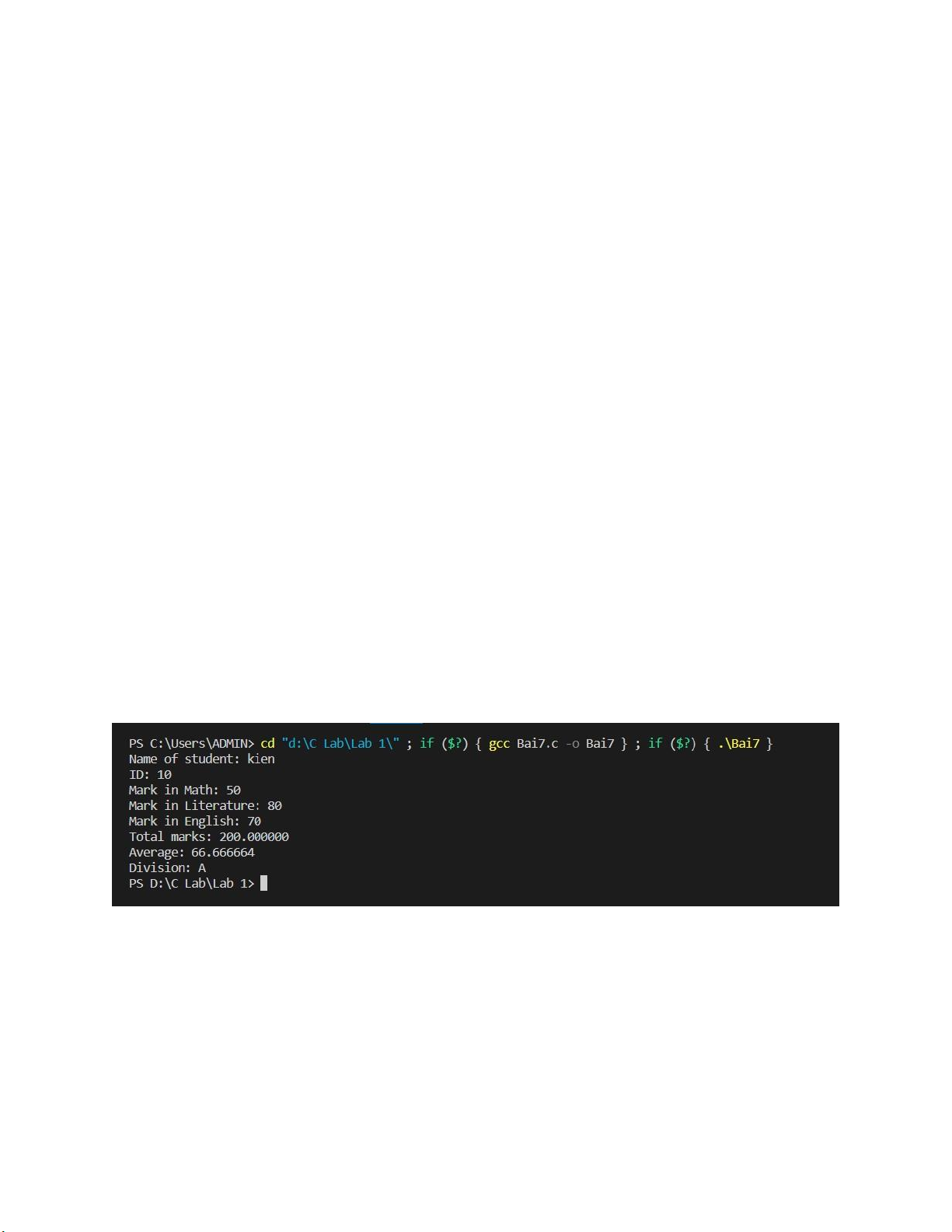
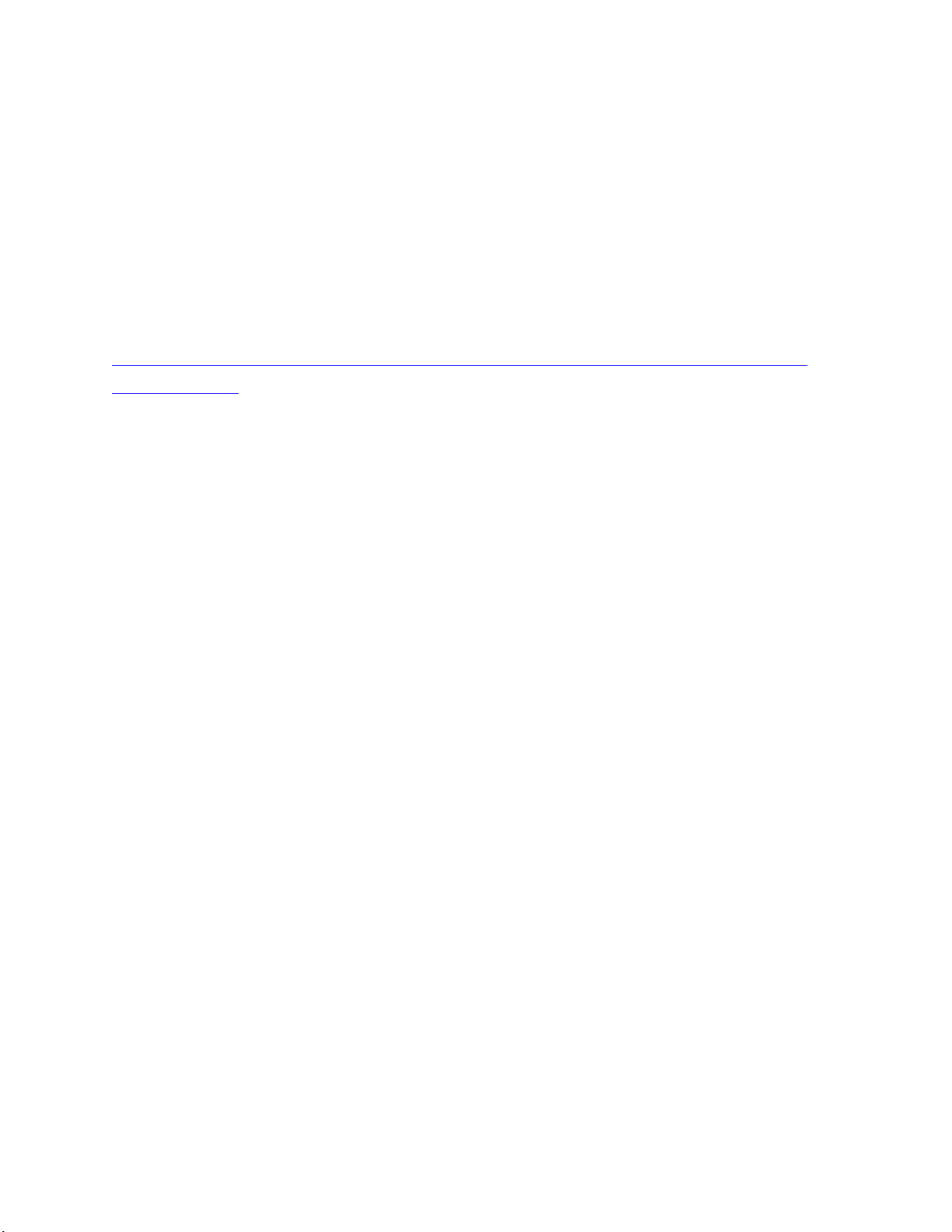
Preview text:
lOMoARcPSD|364 906 32
International University
School of Computer Science and Engineering C/C++ Programming IT116IU
Lab 1: Branching statements Submitted by
[Hồ Ngọc An – ITITIU21146]
[Võ Nguyên Thanh Liêm – ITITIU21069]
[Ngô Trịnh Bảo Anh – ITITIU21154] Date Performed: [8/10/2023] Date Submitted: [11/10/2023] Lab Section: Lab 1 Course Instructor: M. Eng Nguyen Minh Thien GRADING CHECKLIST
International University 1 IT116IU School of CSE lOMoARcPSD|364 906 32 Date: Signature Table of Contents
List of Figures ............................................................................................................................. 2
List of Tables .............................................................................................................................. 3
Discussion of Fundamentals ........................................................................................................ 3
Experimental Procedure .............................................................................................................. 4
Results ........................................................................................................……... 4
Conclusions………...................................................................................................................... 4 List of Figures
Figure 1 –………………………….……...…………………………………………………… x
Figure 2 – …………………………………………………………………..……………...….. x
Figure 3 – ……………………………………………………………………………………... x
International University 2 IT116IU School of CSE lOMoARcPSD|364 906 32
Figure 4 –………………………………………….………………………..……………...….. x
Figure 5 – ……………………………………………………………………………………... x
Figure 6 – ……………............................................................................................................... x List of Tables
Table 1 – ……………………………………..……………………........................................... x
Table 2 – ……………………………………..……………………........................................... x
Table 3 – ……………………………………..……………………........................................... x
Discussion of Fundamentals If Selection Statement
International University 3 IT116IU School of CSE lOMoARcPSD|364 906 32 If … else … statement Fgets function Experimental Procedure Pseudo Code:
International University 4 IT116IU School of CSE lOMoARcPSD|364 906 32 Exercise 1: Step 1: Input a random number
Step 2: We use the modulo operator % to check if the number is even or odd. If num % 2
is equal to 0, it's even; otherwise, it's odd. Step 3: Print the answer. Exercise 2: Step 1: Input a random year
Step 2: If the random year is divisible by 4; 100; 400, thus, the year is the leap year.
Step 3: Else the random year that input is not the leap year Step 4: Print the answer Ex ercise 3:
Step 1: We declare three variables side1, side2, and side3 to store the lengths of the sides of the triangle.
Step 2: We input into the program the lengths of the three sides.
Step 3: We check whether the input values can form a valid triangle by applying the triangle inequality theorem.
Step 4: If the input forms a valid triangle, we proceed to check whether it's equilateral,
isosceles, or scalene based on the lengths of the sides. Step 5: Print the answer. Exercise 4:
Step 1: We declare four double variables: radius to store the user's input, and diameter,
circumference, and area to store the calculated values.
Step 2: We enter the radius of the circle and store it in the radius variable.
Step 3: We calculate the diameter by simply multiplying the radius by 2.
Step 4: We calculate the circumference using the formula 2 * π * radius.
Step 5: We calculate the area using the formula π * radius^2.
Step 6: Finally, we display the calculated values for the diameter, circumference, and
area with appropriate labels. Step 7: Print the answer Exercise 5:
Step 1: We declare variables a, b, and c to store the coefficients of the quadratic equation,
discriminant to calculate the discriminant, and root1 and root2 to store the roots.
Step 2: We prompt the user to enter the coefficients a, b, and c using scanf.
International University 5 IT116IU School of CSE lOMoARcPSD|364 906 32
Step 3: We calculate the discriminant using the formula discriminant = b^2 - 4ac. Step 4:
We use conditional statements to determine the type of roots based on the value of the discriminant:
Step 5: We check the condition of roots.
1. If the discriminant is positive, there are two real and distinct roots.
2. If the discriminant is zero, there is one real root (a double root).
3. If the discriminant is negative, there are complex roots.
Step 6: We calculate and display the roots accordingly, including real and imaginary parts
for complex roots. Step 7: Print the answer Exercise 6:
Step 1: Enter the total distance in kilometers
Step 2: It then uses conditional statements to calculate the total cost based on the entered distance:
1. If the distance is less than or equal to 1 km, the total cost is set to $5.0.
2. If the distance is greater than 1 km but less than or equal to 30 km, the total cost
iscalculated as $5.0 plus $3.0 for each kilometer beyond the first kilometer.
3. If the distance is greater than 30 km, the total cost is calculated as $5.0 for the
first kilometer, $3.0 for each kilometer between 2 km and 30 km, and $2.0 for each kilometer beyond 30 km.
Step 3: Print the calculated total cost with two decimal places using printf. Exercise 7:
Step 1: Declare several variables to store information about a student, including their
name, ID, marks in Math, Literature, and English, as well as variables to calculate the total marks and average.
Step 2: Prompts the user to input the student's name using the fgets function. The fgets
function reads a line of text from the standard input (stdin) and stores it in the name
array, which has a size of 20 characters.
Step 3: Prompts the user to input the student's ID, marks in Math, Literature, and English using the scanf function. Step 4:
1. Calculates the total marks by adding the marks in Math, Literature, and English
and stores the result in the total variable.
2. Calculates the average marks by dividing the total marks by 3 (the number of
subjects) and stores the result in the average variable.
3. Uses conditional statements (if-else if) to determine the student's division based
ontheir average score. There are four possible divisions: "A," "B," "Pass," or
"Fail." The program prints the corresponding division based on the calculated
average. Step 5: Print the answer.
International University 6 IT116IU School of CSE lOMoARcPSD|364 906 32 Experimental Results Problem 1: #include int main() { int number;
printf("Enter an integer: ");
scanf("%d", &number);
if (number % 2 == 0) {
printf("%d is an even integer.\n", number); } else {
printf("%d is an odd integer.\n", number); } return 0; } Result: Problem 2: #include int main() { int year;
// Input year from the user printf("Enter a year: "); scanf("%d", &year);
// Check if the year is divisible by 4 if (year % 4 == 0) {
// Check if the year is divisible by 100
if (year % 100 == 0) {
// Check if the year is divisible by 400 if (year % 400 == 0) {
printf("%d is a leap year.\n", year); } else {
printf("%d is not a leap year.\n", year); } } else {
printf("%d is a leap year.\n", year); } } else {
International University 7 IT116IU School of CSE lOMoARcPSD|364 906 32
printf("%d is not a leap year.\n", year); } return 0; } Result: Problem 3: #include int main() {
double canh1, canh2, canh3;
printf("Enter the length of canh 1: "); scanf("%lf", &canh1);
printf("Enter the length of canh 2: ");
scanf("%lf", &canh2);
printf("Enter the length of canh 3: ");
scanf("%lf", &canh3);
// Check for valid input according to the triangle inequality theorem if
(canh1 + canh2 > canh3 && canh1 + canh3 > canh2 && canh2 + canh3 > canh1) {
if (canh1 == canh2 && canh2 == canh3)
{ printf("It is an Equilateral triangle.\n");
} else if (canh1 == canh2 || canh2 == canh3 || canh1 == canh3)
{ printf("It is an Isosceles triangle.\n"); } else {
printf("It is a Scalene triangle.\n"); } } else {
printf("Invalid input: Not a valid triangle.\n"); } return 0; } Result:
International University 8 IT116IU School of CSE lOMoARcPSD|364 906 32 Problem 4: #include #include int main() {
double radius, diameter, circumference, area;
printf("Enter the radius of the circle: ");
scanf("%lf", &radius); diameter = 2 *
radius; circumference = 2 * 3.14 * radius;
area = 3.14 * radius * radius;
printf("Diameter of the circle: %.2lf\n", diameter);
printf("Circumference of the circle: %.2lf\n", circumference);
printf("Area of the circle: %.2lf\n", area); return 0; } Result: Problem 5: #include #include int main() {
double a, b, c, discriminant, root1, root2;
printf("Enter coefficients (a, b, c): ");
scanf("%lf %lf %lf", &a, &b, &c);
discriminant = b * b - 4 * a * c;
// Check if the discriminant is positive, negative, or zero
if (discriminant > 0) { // Two real and distinct roots
root1 = (-b + sqrt(discriminant)) / (2 * a); root2 =
(-b - sqrt(discriminant)) / (2 * a); printf("Root 1
= %.2lf\n", root1); printf("Root 2 = %.2lf\n",
International University 9 IT116IU School of CSE lOMoARcPSD|364 906 32
root2); } else if (discriminant == 0) { // One
real root (double root) root1 = -b / (2 * a);
printf("Root 1 = Root 2 = %.2lf\n", root1); } else {
// Complex roots double
realPart = -b / (2 * a);
double imaginaryPart = sqrt(-discriminant) / (2 * a);
printf("Root 1 = %.2lf + %.2lfi\n", realPart, imaginaryPart);
printf("Root 2 = %.2lf - %.2lfi\n", realPart, imaginaryPart); } return 0; } Result: Problem 6: #include int main() {
double distance, totalCost;
printf("Enter total distance in km: ");
scanf("%lf", &distance); if (distance
<= 1) totalCost = 5.0; else if (distance <= 30)
totalCost = 5.0 + (distance - 1) * 3.0; else
totalCost = 5.0 + 29 * 3.0 + (distance - 30) * 2.0;
printf("The total cost that a passenger has to pay: $%.2lf\n", totalCost); return 0; } Result: Problem 7: #include int main(){
International University 10 IT116IU School of CSE lOMoARcPSD|364 906 32
float average, id, math, liter, Eng, total; char name[20];
printf("Name of student: ");
fgets(name, sizeof(name)+1, stdin); printf("ID: "); scanf("%f", &id);
printf("Mark in Math: "); scanf("%f", &math);
printf("Mark in Literature: "); scanf("%f", &liter); printf("Mark in English: "); scanf("%f", &Eng);
total = math + liter + Eng;
printf("Total marks: %f\n", total); average = total/3;
printf("Average: %f\n", average); if(average >= 60)
printf("Division: A"); else if(average >= 48) printf("Division: B"); else if(average >= 36)
printf("Division: Pass"); else
printf("Division: Fail"); return 0; } Result: Conclusion
In conclusion, the C lab experiment "If-Else" provided valuable insights into the basic concept of
conditional statements and their practical applications in programming. Through this lab, we
gained a deeper understanding of how to use "if" and "else" statements to control the flow of our
program based on specific conditions.
International University 11 IT116IU School of CSE lOMoARcPSD|364 906 32
Most members participate in completing assigned assignments. Ngoc An completed exercises 1,
4, 5. Bao Anh completed exercises 3, 6. Thanh Liem completed exercises 2,7.
We have successfully implemented conditional statements to address various tasks and scenarios,
such as decision-making, error handling, and user interactions. This exercise also emphasizes the
importance of logical thinking and problem-solving skills when designing and implementing conditional logic. Reference
https://www.geeksforgeeks.org/c-if-else-statement/ https://www.geeksforgeeks.org/fgets- gets-c-language/ THE END
International University 12 IT116IU School of CSE
Document Outline
- Table of Contents
- List of Figures
- List of Tables
- Discussion of Fundamentals
- Experimental Procedure
- Experimental Results
- Conclusion
- Reference