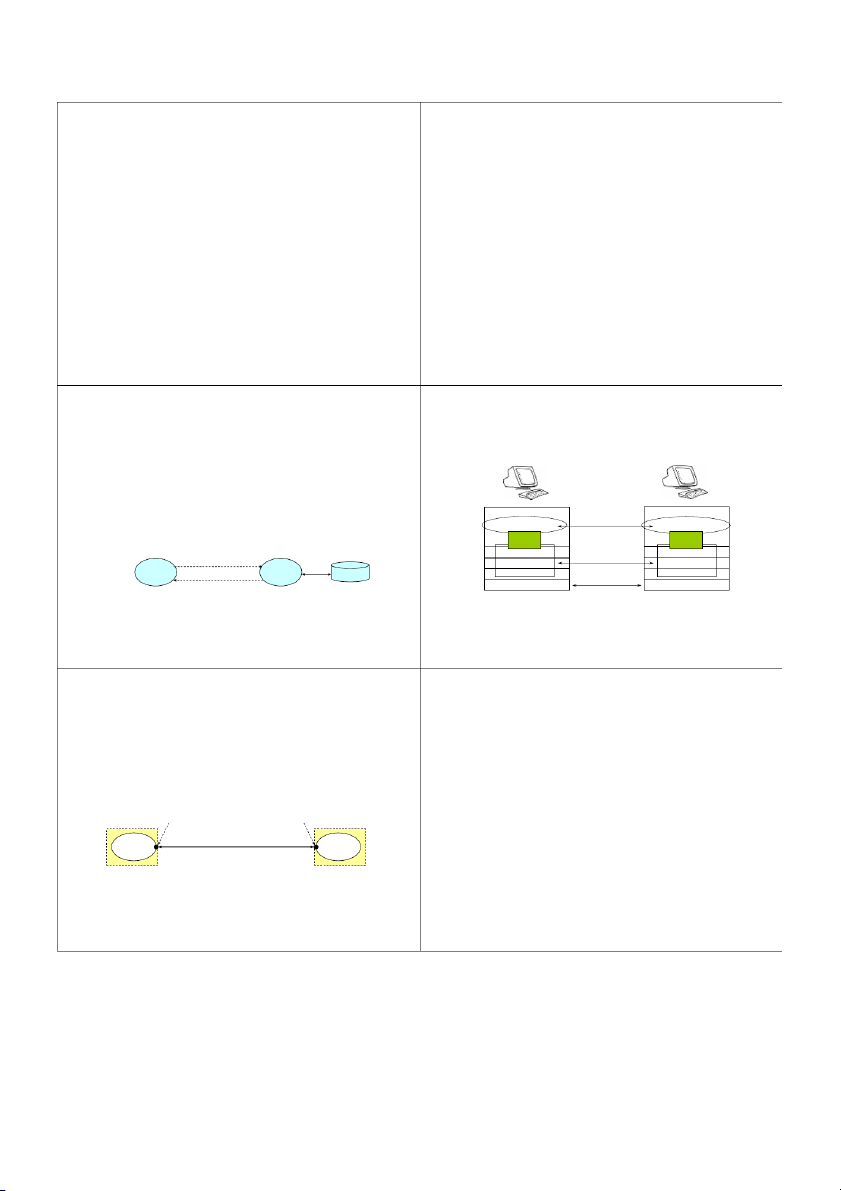
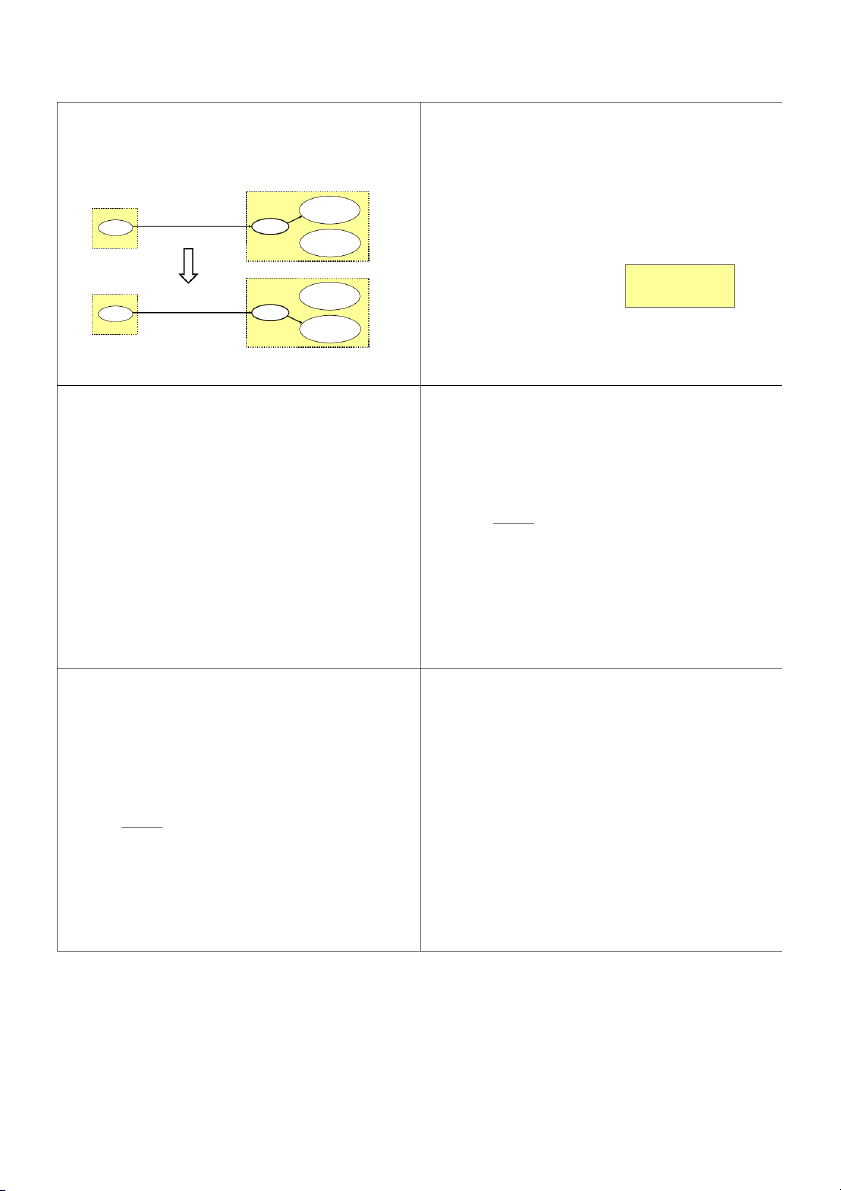
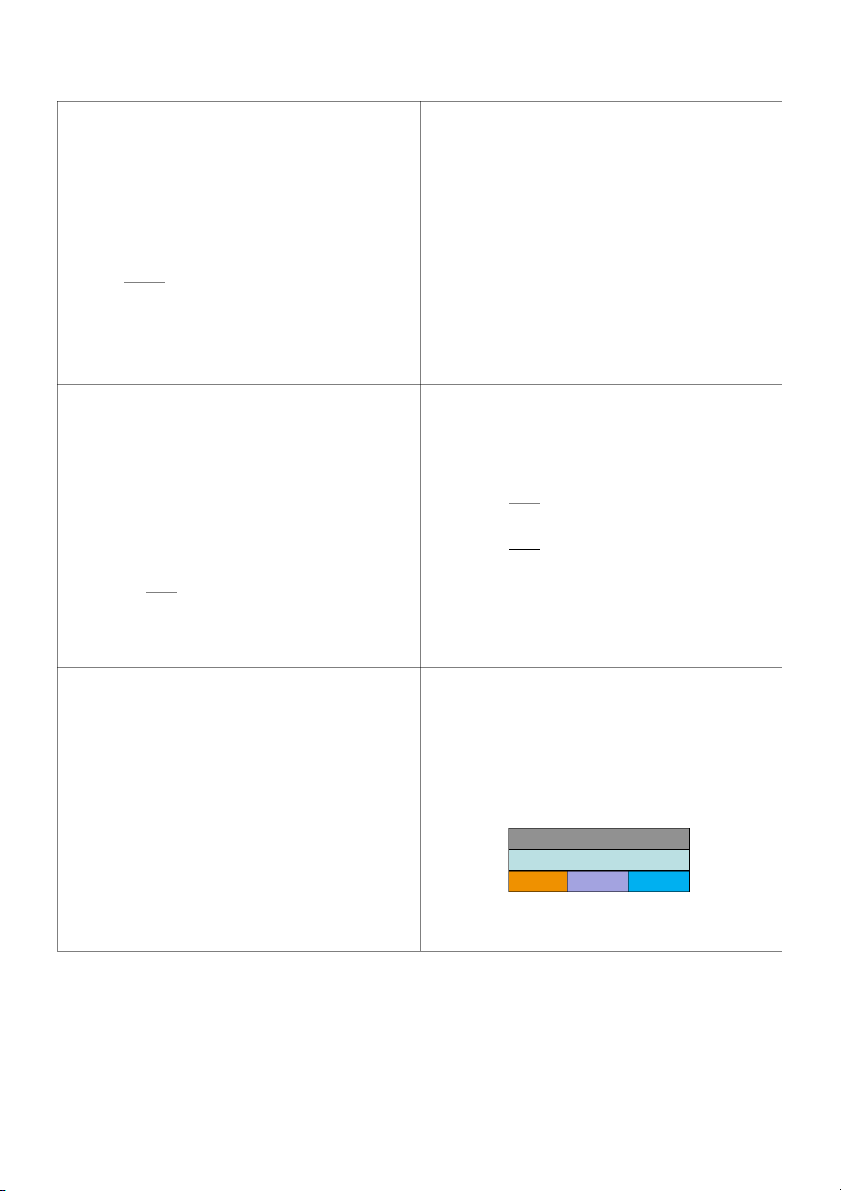
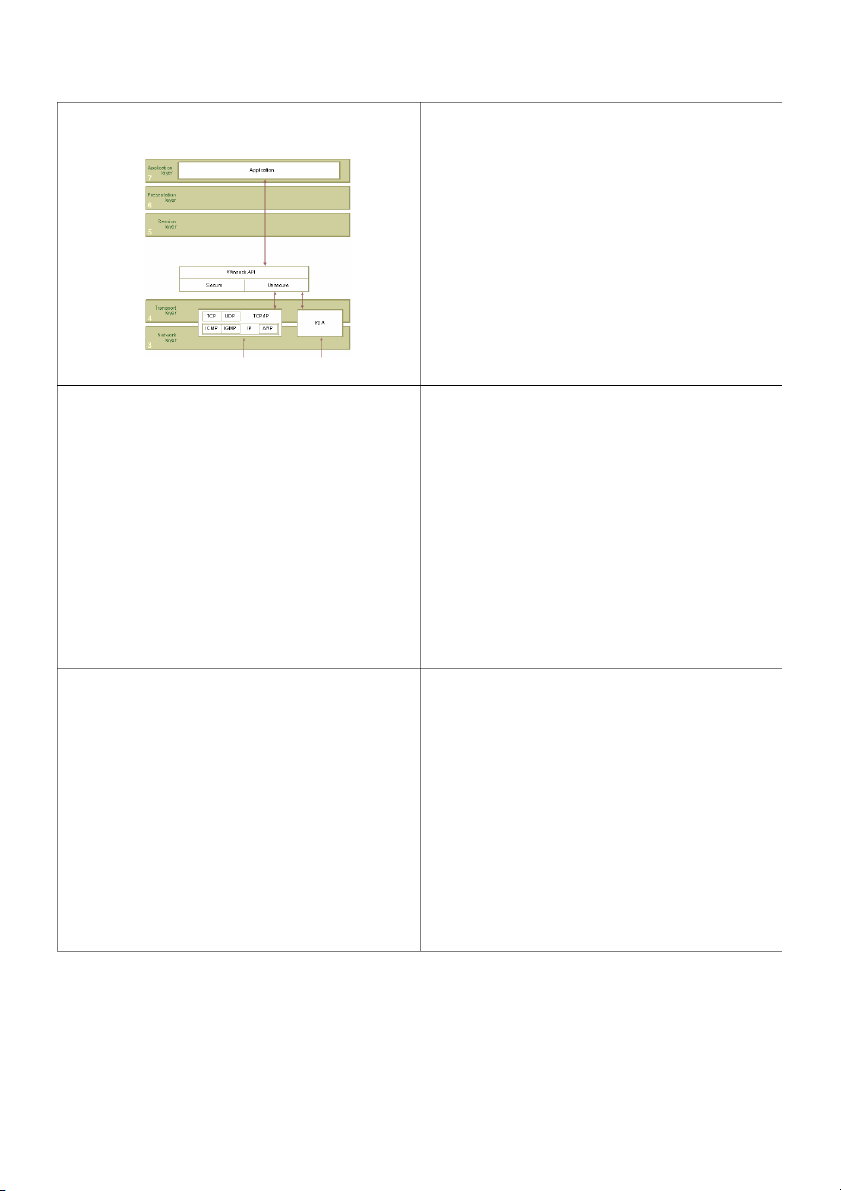
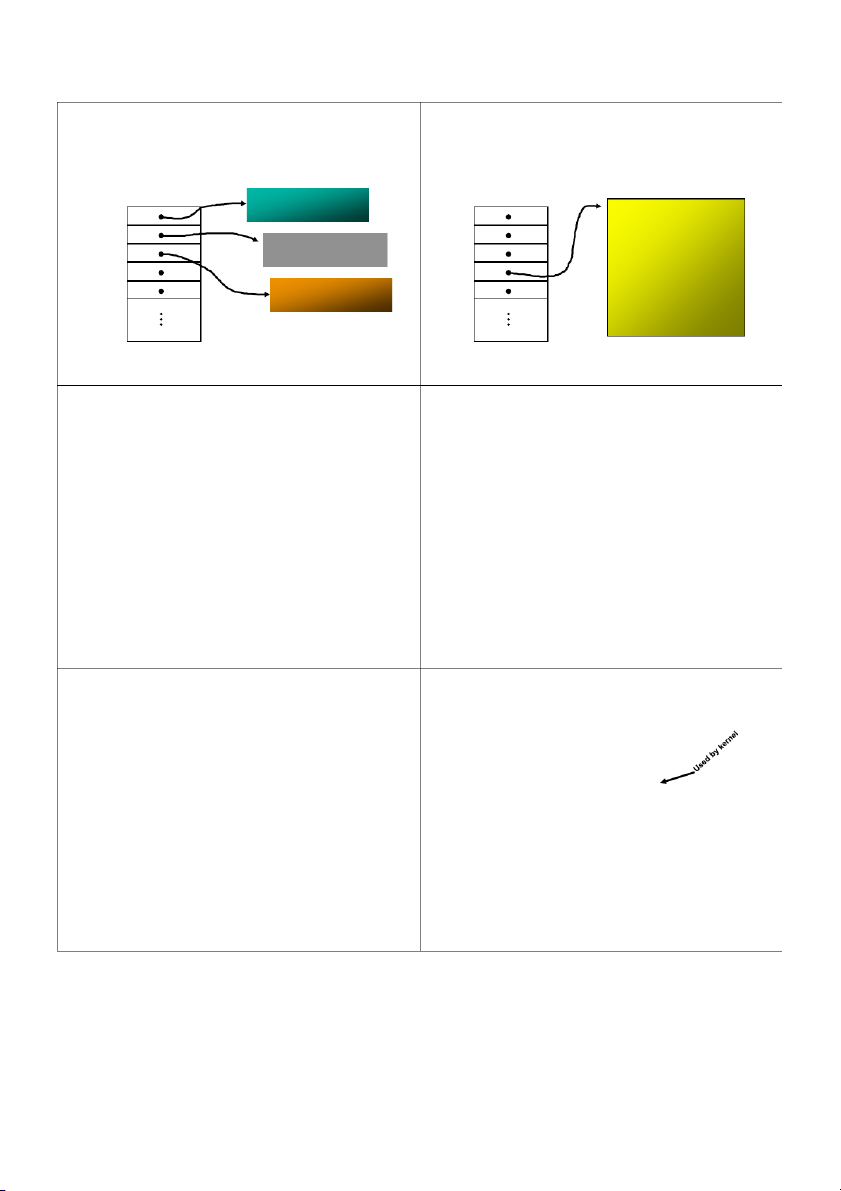
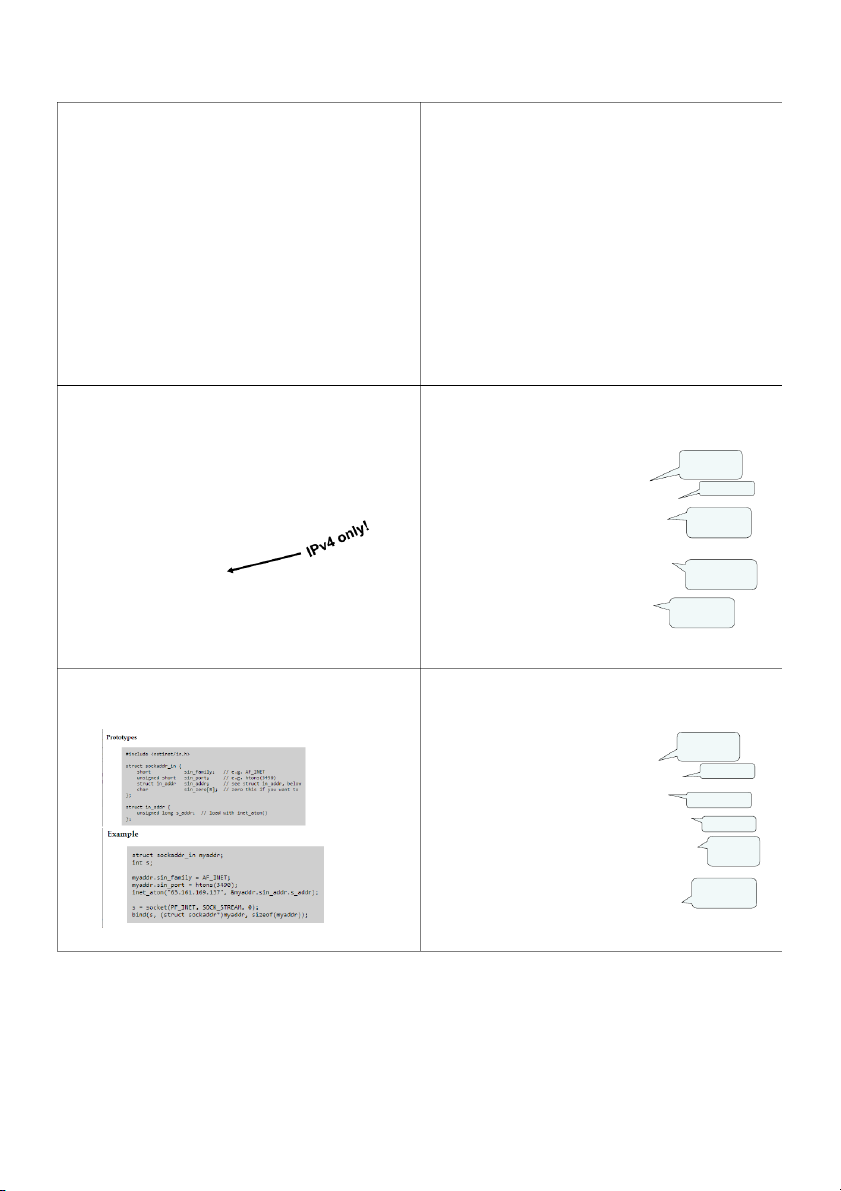
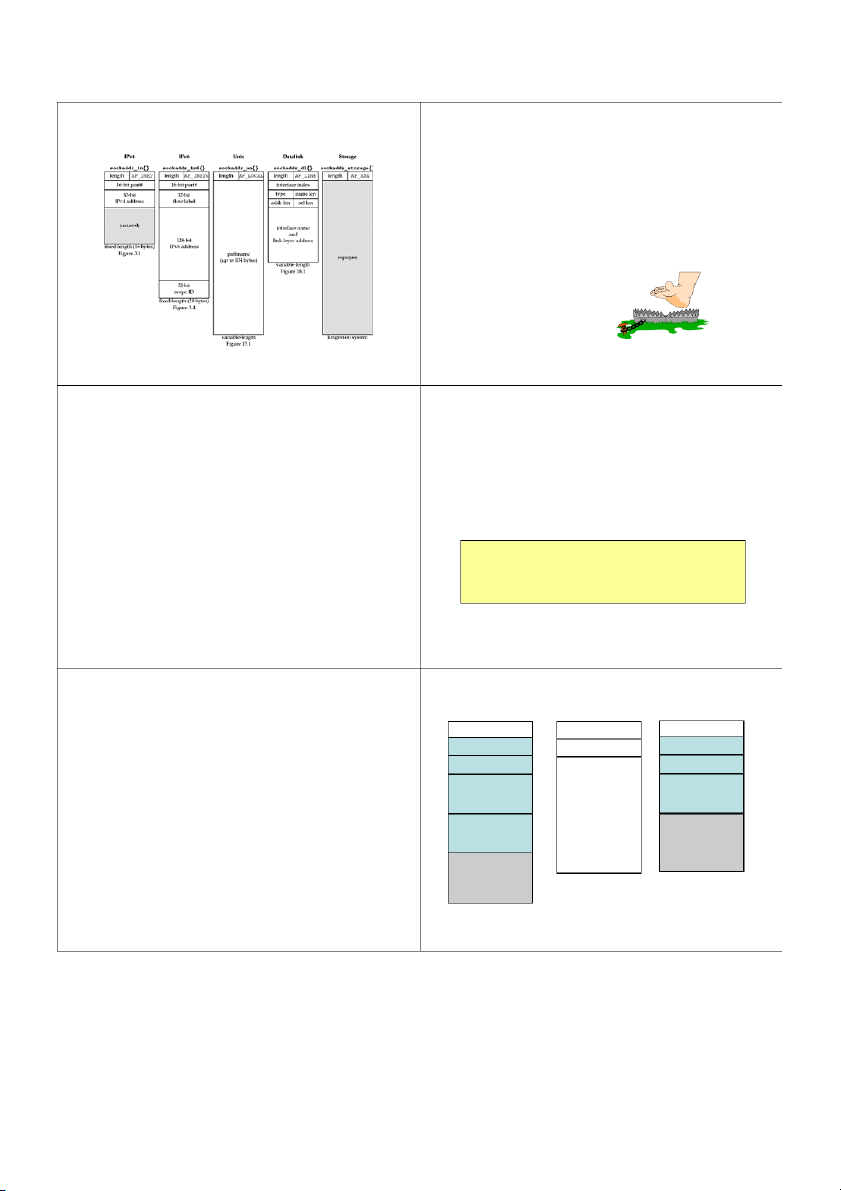
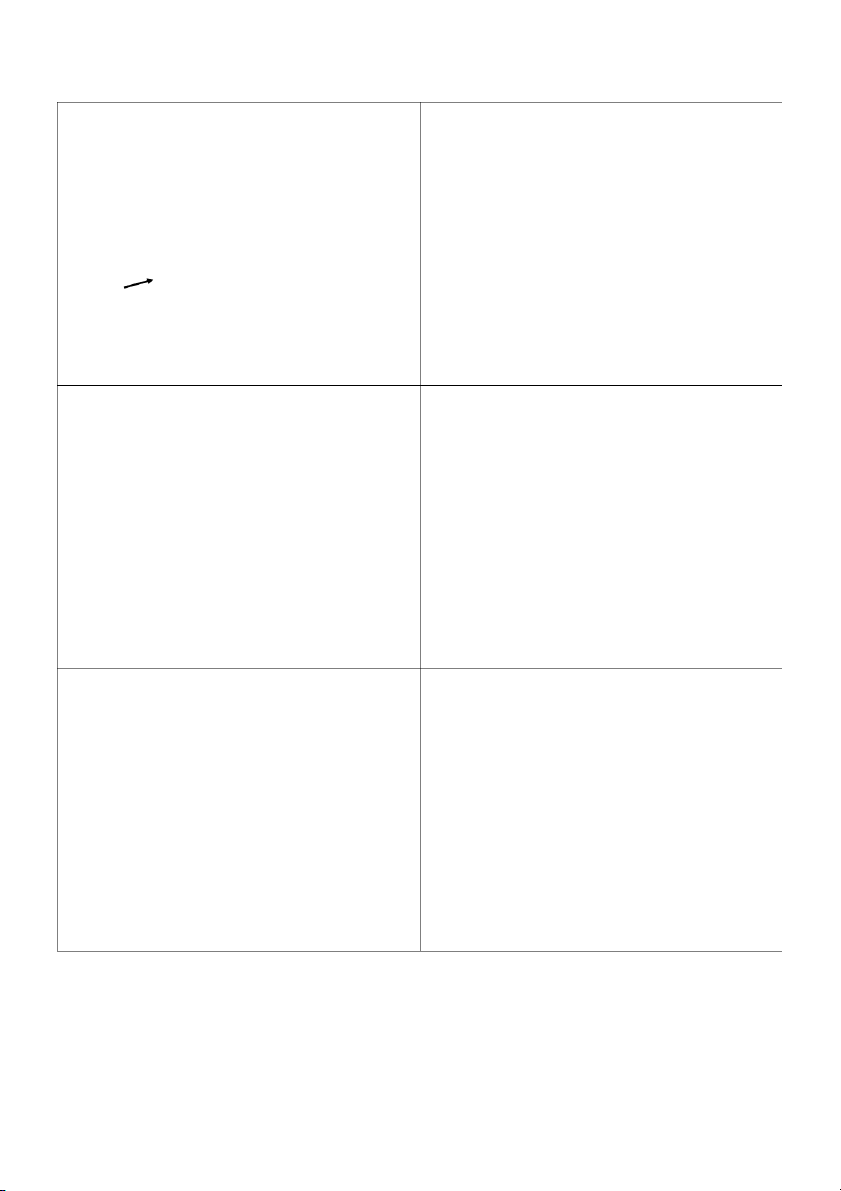
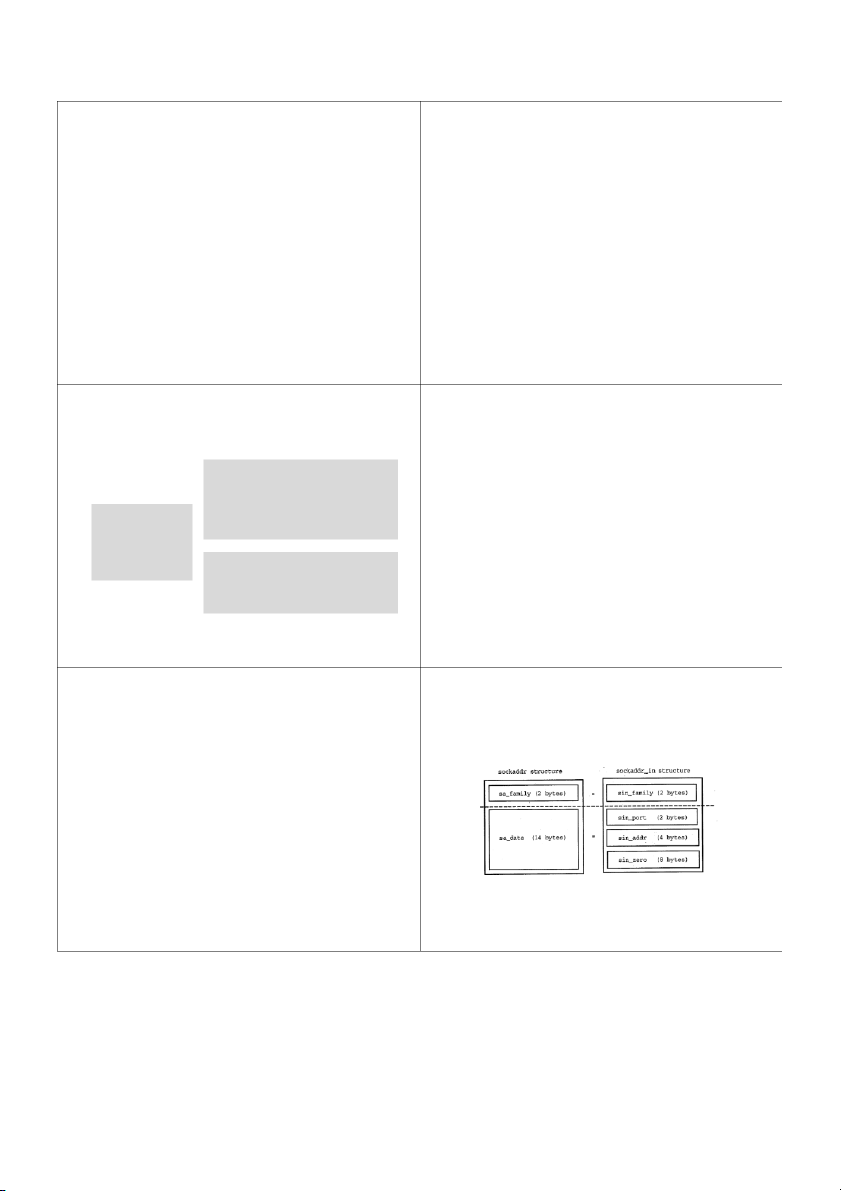
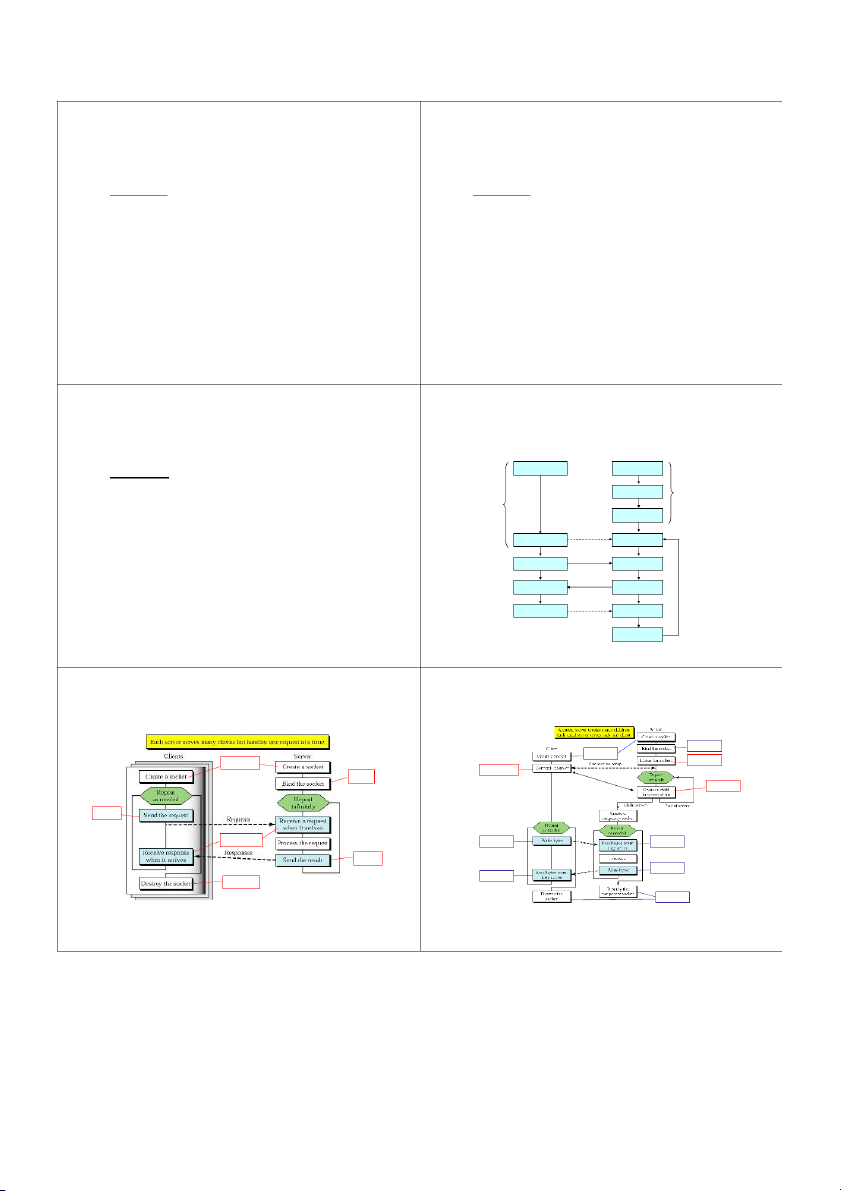
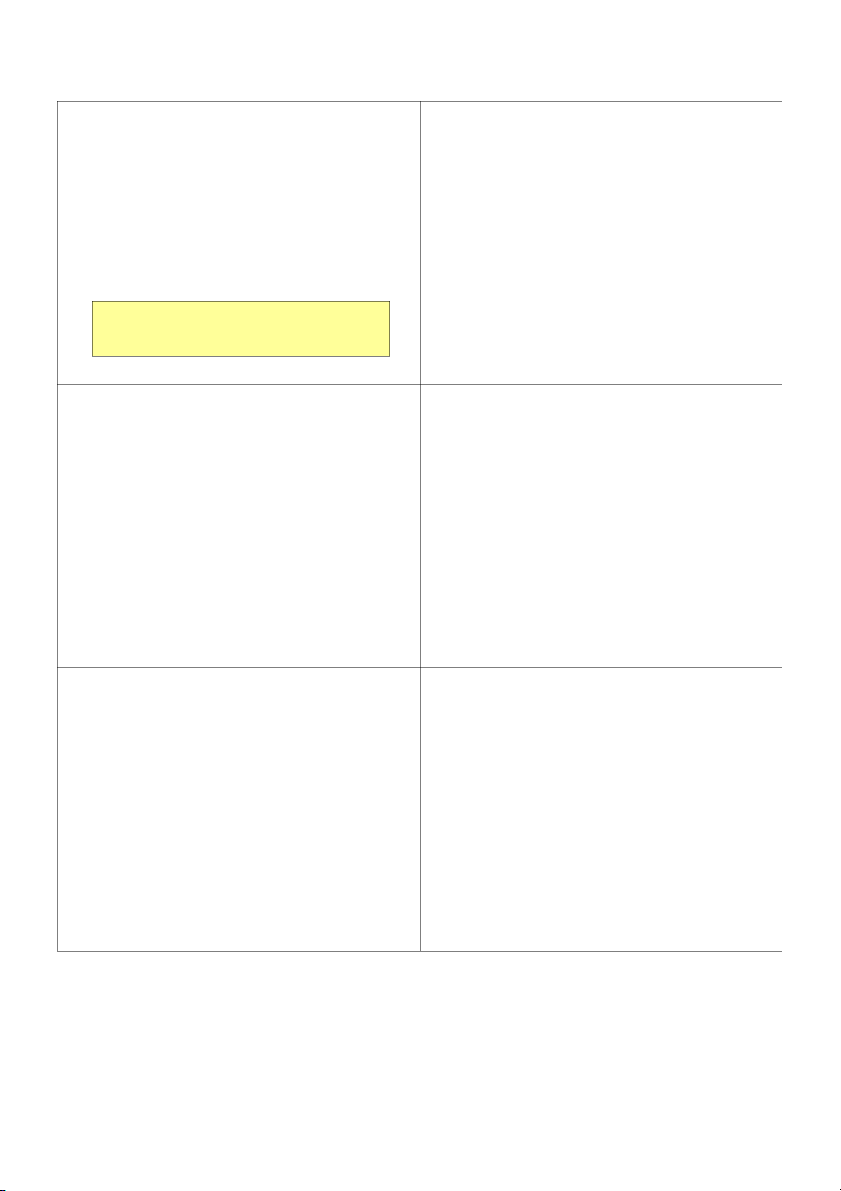
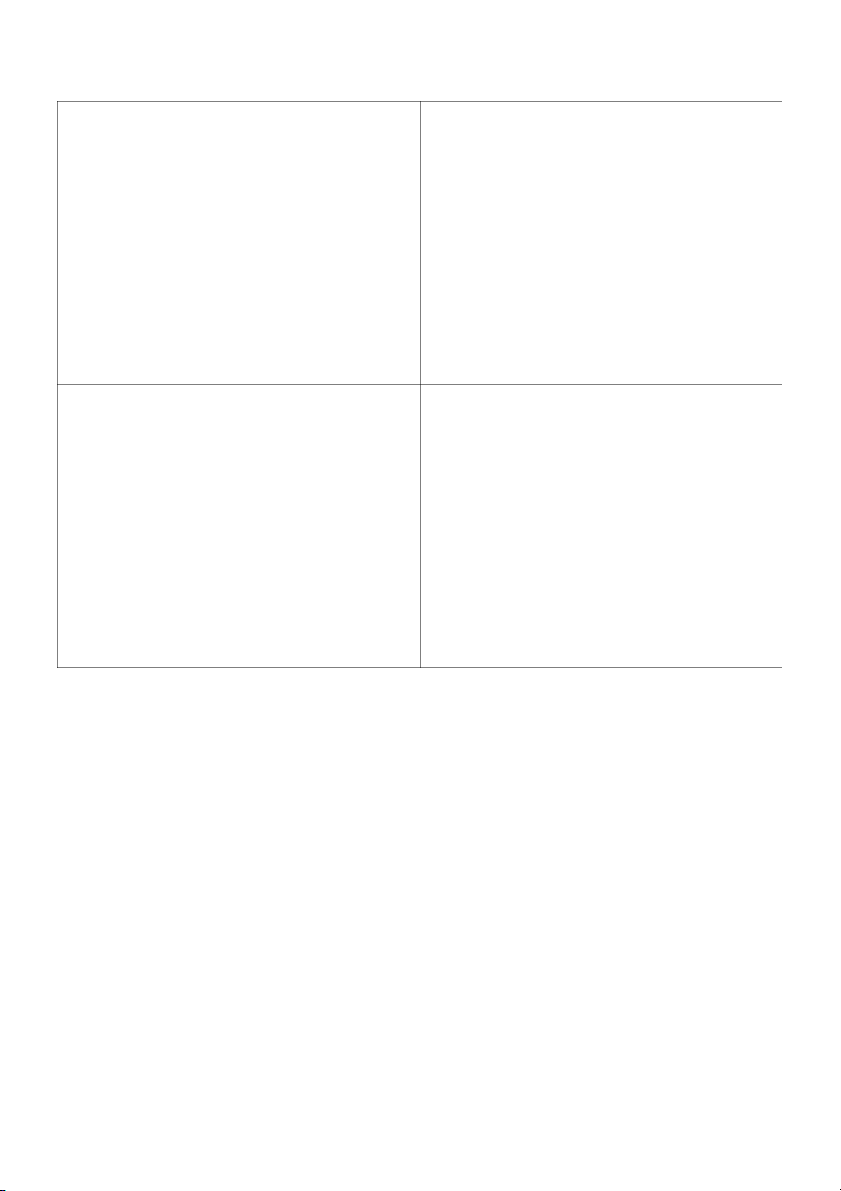
Preview text:
Topics • Client-server model • Sockets interface Computer Networking • Socket primitives
• Example code for echoclient and echoserver Network Programming • Debugging With GDB
• Programming Assignment 1 (MNS) Client/sever model
• Client asks (request) – server provides (response)
• Typically: single server - multiple clients
• The server does not need to know anything about the client – even that it exists
• The client should always know something about the application layer application layer server Client Process Internet Server Process
– at least where it is located Socket Socket
1. Client sends request
transport layer (TCP/UDP)
transport layer (TCP/UDP) OS network OS network Internet network layer (IP) network layer (IP) Client Server stack stack process process Resource
link layer (e.g. ethernet)
link layer (e.g. ethernet) 4. Client Internet
3. Server sends response 2. Server physical layer physical layer handles handles response request
The interface that the OS provides to its networking subsystem
Note: clients and servers are processes running on hosts
(can be the same or different hosts). Internet Connections (TCP/IP) Clients
• Examples of client programs
• Address the machine on the network
– Web browsers, ftp, telnet, ssh – By IP address • Address the process
• How does a client find the server? – By the “port”-number
– The IP address in the server socket address identifies the
• The pair of IP-address + port – makes up a “socket-address” host
Client socket address
Server socket address
– The (well-known) port in the server socket address identifies 128.2.194.242:3479 208.216.181.15:80
the service, and thus implicitly identifies the server process that performs that service. Server
– Examples of well known ports Client Connection socket pair (port 80) • Port 7: Echo server
(128.2.194.242:3479, 208.216.181.15:80) • Port 23: Telnet server Client host address Server host address • Port 25: Mail server 128.2.194.242 208.216.181.15 • Port 80: Web server Note: 3479 is an
Note: 80 is a well-known port
ephemeral port allocated
associated with Web servers by the kernel Using Ports to Identify Servers Services
• Servers are long-running processes (daemons).
Server host 128.2.194.242
– Created at boot-time (typically) by the init process Client host Service request for (process 1) Web server 128.2.194.242:80 (port 80)
– Run continuously until the machine is turned off. (i.e., the Web server) Client Kernel
• Each server waits for requests to arrive on a Echo server (port 7)
well-known port associated with a particular service. – Port 7: echo server
See /etc/services for a – Port 23: telnet server
comprehensive list of the Service request for Web server
services available on a 128.2.194.242:7 (port 80) – Port 25: mail server Linux machine. (i.e., the echo server) Client Kernel – Port 80: HTTP server Echo server
• Other applications should choose between 1024 and (port 7) 65535 1. Review of C Programming 1. Review of C Programming 1.1 Some data types 1.1 Some data types, cont.
– u_char unsigned 8-bit character
– structure: a group of different types of
– u_shortunsigned 16-bit integer data
– u_long unsigned 32-bit integer Example struct info { char name[20]; int id; }; …
struct info student[40]; student[0].name = “Tony”; 9 student[0].id = 1234; 10 1. Review of C Programming 1. Review of C Programming 1.2 Casting of Data Types 1.3 Pointers
– Casting: to change the data type of a
– Every memory location has an address
variable or expression to the designated
we can use this address to access the one.
content in this memory location. Example
– A pointer is a variable that stores the
If x is an int, the data type of the following
memory address (not the value) of a data expression is item. double (double)(x+1); 11 12 1. Review of C Programming 1. Review of C Programming 1.3 Pointers 1.4 Pointers and array
– Declare a pointer: data-type *pointer-variable;
– The name of an array is actually a pointer to the
– Dereference operator *: *p gives the content
first element in the array.
stored in the memory location with address p.
– If x is a one-dimensional array,
– Address operator &: The address storing the first
memory byte of the variable x in &x.
The address of the 1st array element is &x[0] or x; – Example:
The address of the 2nd array element is &x[1] or (x+1);
float x, y, *p; /* declare p as a pointer of float … p = &x;
/* assign the address of x to p y = *p;
/* assign the content of p to y 13 14 1. Review of C Programming 1. Review of C Programming
1.5 Byte-manipulation functions
1.5 Byte-manipulation functions
– We may not use string functions in network
• memcpy() copies the value of one field to another programming. Why? Example
Copy the value of y field to the x field:
• Three byte-manipulation functions
memcpy(&x, &y, sizeof(x));
– void *memset(void *dest, int chr, int len);
• memcmp() compares two fields
– void *memcpy(void *dest, const void *src, int len); Example
– int memcmp(const void *first, const void *secnd, int
Compares the first 10 bytes of fields x and y: len);
if (memcpy(&x, &y,10) == 0)
• memset() specified number of bytes to a value.
printf(“x and y are the same”); Example
Stores zeros in a field called x: memset(&x, 0, sizeof(x)); 15 16 Network Application Sockets Programming Interface (API) • What is a socket?
– To the kernel, a socket is an endpoint of communication.
• Services that provide the interface between
– To an application, a socket is a file descriptor that lets the
application read/write from/to the network.
application and protocol software (often by the
• Remember: All Unix I/O devices, including networks, are operating system). modeled as files.
• Clients and servers communicate with each by reading
from and writing to socket descriptors. Application
• The main distinction between regular file I/O and
socket I/O is how the application “opens” the socket Network API descriptors. Protocol A Protocol B Protocol C Socket Programming 18 Network API wish list
• Generic Programming Interface
– Support multiple communication protocol suites (families).
– Address (endpoint) representation independence.
– Provide special services for Client and Server?
• Support for message oriented and connection oriented communication
• Work with existing I/O services (when this makes sense)
• Operating System independence Socket Programming 20 TCP/IP Functions needed:
• TCP/IP does not include an API
• Specify local and remote communication definition. endpoints • Initiate a connection
• There are a variety of APIs for use with TCP/IP:
• Wait for incoming connection – Sockets • Send and receive data – TLI, XTI – Winsock
• Terminate a connection gracefully – MacTCP • Error handling Socket Programming 21 Socket Programming 22 Berkeley Sockets Socket • Generic:
• A socket is an abstract representation
– support for multiple protocol families. of a communication endpoint.
– address representation independence
• Sockets work with Unix I/O services
just like files, pipes & FIFOs.
• Uses existing I/O programming interface as much as possible.
• Sockets (obviously) have special needs: – establishing a connection
– specifying communication endpoint addresses Socket Programming 23 Socket Programming 24 Socket Descriptor Data Unix Descriptor Table Structure Descriptor Table Descriptor Table
Data structure for file 0 0 0 Family: PF_INET 1 1 Service: SOCK_STREAM Local IP: 111.22.3.4 2
Data structure for file 1 2 Remote IP: 123.45.6.78 Local Port: 2249 3 3 Remote Port: 3726 4 4
Data structure for file 2 Socket Programming 25 Socket Programming 26 Creating a Socket socket()
int socket(int family,int type,int proto);
• The socket()system call returns a
socket descriptor (small integer) or -1
• family specifies the protocol family (PF_INETfor TCP/IP). on error.
• type specifies the type of service (SOCK_STREAM, SOCK_DGRAM).
• socket()allocates resources needed
• protocol specifies the specific protocol for a communication endpoint
(usually 0, which means the default).
– but it does not deal with endpoint addressing. Socket Programming 27 Socket Programming 28 Specifying an Endpoint Address Generic socket addresses • Sockets API is generic. struct sockaddr {
• There must be a generic way to specify uint8_t sa_len; endpoint addresses. sa_family_t sa_family;
• TCP/IP requires an IP address and a char sa_data[14];
port number for each endpoint address. };
• Other protocol suites (families) may use other schemes.
• sa_family specifies the address type.
• sa_data specifies the address value. Socket Programming 29 Socket Programming 30 sockaddr AF_FAMILY
• An address that will allow me to use sockets
• Initializing a sockaddr structure to to communicate with my family. point to Daughter: • address type AF_FAMILY struct sockaddr mary; • address values: Daughter 1 Wife 2 mary.sa_family = AF_FAMILY; Mom 3 mary.sa_data[0] = 1; Dad 4 Sister 5 Brother 6 Socket Programming 31 Socket Programming 32 AF_INET struct sockaddr_in (IPv4) struct sockaddr_in { Length of
• For AF_FAMILY we only needed 1 byte structure (16) uint8_t sin_len; to specify the address. AF_INET sa_family_t sin_family; in_port_t sin_port; 16 bit Port number struct in_addr sin_addr; • For AF_INET we need: char sin_zero[8]; – 16 bit port number }; Make structure 16 bytes – 32 bit IP address struct in_addr { in_addr_t s_addr; 32 bit IPv4 address }; Socket Programming 33 Socket Programming 34 struct sockaddr_in (IPv4) struct sockaddr_in (IPv6) struct sockaddr_in6 { Length of structure (28) uint8_t sin6_len; sa_family_t sin6_family; AF_INET6 in_port_t sin6_port; Port number uint32_t sin6_flowinfo; Flow label struct in6_addr sin6_addr; uint32_t sin6_scope_id; Scope of address }; struct in6_addr { 128 bit uint8_t s6_addr[16]; IPv6 address }; Socket Programming 36 Network Byte Order
• All values stored in a sockaddr_in must be in network byte order.
– sin_port a TCP/IP port number. – sin_addr an IP address. !!! Common Mistake !!! Socket Programming 37 Socket Programming 38 Network Byte Order Functions Network Byte Order Functions • Network Byte Ordering
– Network is big-endian, host may be big- or little-endian
‘h’ : host byte order ‘n’ : network byte order
– Functions work on 16-bit (short) and 32-bit (long) values
‘s’ : short (16bit) ‘l’ : long (32bit)
– htons() / htonl() : convert host byte order to network byte order
– ntohs() / ntohl(): convert network byte order to host byte order
– Use these to convert network addresses, ports, … uint16_t htons(uint16_t); uint16_t ntohs(uint_16_t);
struct sockaddr_in serveraddr;
/* fill in serveraddr with an address */ …
/* Connect takes (struct sockaddr *) as its second argument */ uint32_t htonl(uint32_t);
connect(clientfd, (struct sockaddr *) &serveraddr, sizeof(serveraddr)); uint32_t ntohl(uint32_t); … • Structure Casts Socket Programming 39
– You will see a lot of ‘structure casts’ sockaddr_in6 sockaddr sockaddr_in TCP/IP Addresses length sa_len length
• We don’t need to deal with sockaddr AF_INET6 sa_family AF_INET
structures since we will only deal with a port port real protocol family. Flow-label addr
• We can use sockaddr_in structures.
• BUT: The C functions that make up the sa_data
sockets API expect structures of type addr zero sockaddr. Scope ID variable 16 bytes 28 bytes Socket Programming 41 Socket Programming 42
Assigning an address to a socket bind()
• calling bind() assigns the address specified sockaddr
• The bind()system call is used to assign an by the structure to the socket address to an existing socket. descriptor. bind() sockaddr_in int bind( int sockfd, • You can give a structure:
const struct sockaddr *myaddr, const! int addrlen); bind( mysock,
(struct sockaddr*) &myaddr, sizeof(myaddr) );
• bindreturns 0 if successful or -1 on error. Socket Programming 43 Socket Programming 44 bind()Example Uses for bind() int mysock,err;
• There are a number of uses for struct sockaddr_in myaddr; bind():
mysock = socket(PF_INET,SOCK_STREAM,0);
– Server would like to bind to a well known myaddr.sin_family = AF_INET; address (port number).
myaddr.sin_port = htons( portnum );
myaddr.sin_addr = htonl( ipaddress);
– Client can bind to a specific port.
err=bind(mysock, (sockaddr *) &myaddr, sizeof(myaddr));
– Client can ask the O.S. to assign any available port number. Socket Programming 45 Socket Programming 46 Port schmort - who cares ? What is my IP address ?
• Clients typically don’t care what port
• How can you find out what your IP address is so you can tell bind()? they are assigned.
• There is no realistic way for you to know the
• When you call bind you can tell it to
right IP address to give bind() assign you any available port:
– what if the computer has multiple network interfaces? myaddr.port = htons(0);
• specify the IP address as: INADDR_ANY,
this tells the OS to take care of things. Socket Programming 47 Socket Programming 48 IPv4 Address Conversion
IPv4 & IPv6 Address Conversion
int inet_aton( char *, struct in_addr *);
int inet_pton( int, const char *, void *);
– Convert ASCII dotted-decimal IP address
– (family, string_ptr, address_ptr)
to network byte ordered 32 bit value.
– Convert IP address string to network byte ordered
– Returns 1 on success, 0 on failure. 32 or 128 bit value.
– Returns 1 on success, -1 on failure, 0 on invalid input
char *inet_ntoa(struct in_addr);
char *inet_ntop(int,const void*,char*,size_t);
– Convert network byte ordered value to
– (family, address_ptr, string_ptr, length)
ASCII dotted-decimal (a string).
– Convert network byte ordered value to IP address string
• x:x:x:x:x:x:x:x or x:x:x:x:x:x:a.b.c.d Socket Programming 49 Socket Programming 50 Other socket system calls 3.Endpoint Address • Connection-oriented (TCP)
• To deal with struct sockaddr, a compatible – connect()
structure for TCP/IP endpoint address was created as follows. • General Use – listen() struct sockaddr_in { – accept() – read() u_short sin_family; /* address family */ – write() u_short sin_port; /* port number */ • Connectionless (UDP)
struct in_addr sin_addr; /* IP address */ – close() – send() char sin_zero[8]; /* unused (set to zero) */ } – recv() 52 Socket Programming 51 3.Endpoint Address 3.Endpoint Address
• The structure of in_addr is as follows.
• sockaddr and sockaddr_in are struct in_addr { c
u_long s_addr; /* that's a 32-bit long, or 4 bytes */ }
If you only use TCP/IP, you can use
sockaddr_in without using sockaddr. 53 54 3.Endpoint Address 3.Endpoint Address • Example 1 • Example 1, cont.
– The IP address of a server is 192.168.20.56. Its
– Uses inet_addr() converts a string in dotted
decimal value is 3232240696. We can specify the
decimal (e.g., 192.168.20.56) to an integer value
endpoint address for this server as follows.
suitable for use as an Internet address. struct sockaddr_in serverAddr; struct sockaddr_in serverAddr; …
char * serverIP = "192.168.20.56";
serverAddr.sin_family = AF_INET; …
serverAddr.sin_port = htons(2000);
serverAddr.sin_family = AF_INET;
serverAddr.sin_addr.s_addr = htonl(3232240696);
serverAddr.sin_port = htons(2000);
serverAddr.sin_addr.s_addr = inet_addr(serverIP); 55 56 3.Endpoint Address Overview of Sockets Interface Client Server • Example 2 socket socket
– We specify the endpoint address for a server as bind open_listenfd follows: open_clientfd struct sockaddr_in serverAddr; listen … Connection
serverAddr.sin_family = AF_INET; request connect accept
serverAddr.sin_port = htons(2000);
serverAddr.sin_addr.s_addr = htonl(INADDR_ANY); write read Await connection
• Where the symbolic constant INADDR_ANY represents a request from
wildcard address that matches any of the computer's IP address. read write next client EOF close read 57 close Client+server: connectionless
Client+server: connection-oriented BIND SOCKET CREATE LISTEN CONNECT BIND ACCEPT TCP three-way handshake SEND RECEIVE SEND RECEIVE SEND SEND RECEIVE CLOSE CLOSE Concurrent server Socket primitives
• LISTEN: int listen(int sockfd, int backlog);
– backlog: how many connections we want to queue
• SOCKET: int socket(int domain, int type, int
• ACCEPT: int accept(int sockfd, void *addr, int *addrlen);
protocol);
– addr: here the socket-address of the caller will be written
– domain := AF_INET (IPv4 protocol)
– returned: a new socket descriptor (for the temporal socket)
– type := (SOCK_DGRAM or SOCK_STREAM )
• CONNECT: int connect(int sockfd, struct sockaddr
*serv_addr, int addrlen); //used by TCP client
– protocol := 0 (IPPROTO_UDP or IPPROTO_TCP)
– parameters are same as for bind()
– returned: socket descriptor (sockfd), -1 is an error
• SEND: int send(int sockfd, const void *msg, int len, int flags);
– msg: message you want to send
• BIND: int bind(int sockfd, struct sockaddr
– len: length of the message
*my_addr, int addrlen); – flags := 0
– sockfd - socket descriptor (returned from socket())
– returned: the number of bytes actually sent –
• RECEIVE: int recv(int sockfd, void *buf, int len, unsigned int
my_addr: socket address, struct sockaddr_in is used flags);
– addrlen := sizeof(struct sockaddr)
– buf: buffer to receive the message struct sockaddr_in {
– len: length of the buffer (“don’t give me more!”)
unsigned short sin_family; /* address family (always AF_INET) */ – flags := 0
unsigned short sin_port; /* port num in network byte order */
– returned: the number of bytes received
struct in_addr sin_addr; /* IP addr in network byte order */
unsigned char sin_zero[8]; /* pad to sizeof(struct sockaddr) */ };
• SEND (DGRAM-style): int sendto(int sockfd, const void *msg, EchoClient.c – #include’s
int len, int flags, const struct sockaddr *to, int tolen);
– msg: message you want to send
– len: length of the message – flags := 0
#include /* for printf() and fprintf() */
– to: socket address of the remote process
#include /* for socket(), connect(),
– tolen: = sizeof(struct sockaddr) sendto(), and recvfrom() */
– returned: the number of bytes actually sent
#include /* for sockaddr_in and
• RECEIVE (DGRAM-style): int recvfrom(int sockfd, void *buf, inet_addr() */
int len, unsigned int flags, struct sockaddr *from, int
*fromlen);
#include /* for atoi() and exit() */
– buf: buffer to receive the message #include /* for memset() */
– len: length of the buffer (“don’t give me more!”) #include /* for close() */
– from: socket address of the process that sent the data
– fromlen:= sizeof(struct sockaddr) – flags := 0
#define ECHOMAX 255 /* Longest string to echo */
– returned: the number of bytes received
• CLOSE: close (socketfd);
EchoClient.c - creating the socket and
EchoClient.c -variable declarations sending
int main(int argc, char *argv[])
/* Create a datagram/UDP socket */ {
sock = socket(AF_INET, SOCK_DGRAM, 0);
int sock; /* Socket descriptor */
struct sockaddr_in echoServAddr; /* Echo server address */
/* Construct the server address structure */
struct sockaddr_in fromAddr; /* Source address of echo */
memset(&echoServAddr, 0, sizeof(echoServAddr)); /* Zero out
unsigned short echoServPort =7; /* Echo server port */ structure */
unsigned int fromSize; /* address size for recvfrom() */
echoServAddr.sin_family = AF_INET; /* Internet addr family */
char *servIP=“172.24.23.4”; /* IP address of server
echoServAddr.sin_addr.s_addr = htonl(servIP); /* Server IP */ address */
char *echoString=“I hope this works”; /* String to send
echoServAddr.sin_port = htons(echoServPort); /* Server port */ to echo server */
char echoBuffer[ECHOMAX+1]; /* Buffer for receiving
/* Send the string to the server */ echoed string */
sendto(sock, echoString, echoStringLen, 0, (struct sockaddr *)
int echoStringLen; /* Length of string to echo */
&echoServAddr, sizeof(echoServAddr);
int respStringLen; /* Length of received response */ /* Recv a response */ EchoServer.c
EchoClient.c – receiving and printing
int main(int argc, char *argv[]) { int sock; /* Socket */
struct sockaddr_in echoServAddr; /* Local address */ fromSize = sizeof(fromAddr);
struct sockaddr_in echoClntAddr; /* Client address */
recvfrom(sock, echoBuffer, ECHOMAX, 0, (struct sockaddr *)
unsigned int cliAddrLen; /* Length of incoming message */ &fromAddr, &fromSize);
char echoBuffer[ECHOMAX]; /* Buffer for echo string */
/* Error checks like packet is received from the same server*/
unsigned short echoServPort =7; /* Server port */
int recvMsgSize; /* Size of received message */
/* null-terminate the received data */
/* Create socket for sending/receiving datagrams */
echoBuffer[echoStringLen] = '\0';
sock = socket(AF_INET, SOCK_DGRAM, 0);
printf("Received: %s\n", echoBuffer); /* Print the echoed arg */
/* Construct local address structure */ close(sock);
memset(&echoServAddr, 0, sizeof(echoServAddr)); /* Zero out structure */ exit(0);
echoServAddr.sin_family = AF_INET; /* Internet address family */
echoServAddr.sin_addr.s_addr = htonl(“172.24.23.4”); } /* end of main () */
echoServAddr.sin_port = htons(echoServPort); /* Local port */
/* Bind to the local address */
bind(sock, (struct sockaddr *) &echoServAddr, sizeof(echoServAddr); for (;;) /* Run forever */ Socket Programming Help {
cliAddrLen = sizeof(echoClntAddr); • man is your friend
/* Block until receive message from a client */ – man accept
recvMsgSize = recvfrom(sock, echoBuffer, ECHOMAX, 0,
(struct sockaddr *) &echoClntAddr, &cliAddrLen); – man sendto
printf("Handling client %s\n", inet_ntoa(echoClntAddr.sin_addr)); – Etc.
• The manual page will tell you:
/* Send received datagram back to the client */
sendto(sock, echoBuffer, recvMsgSize, 0,
– What #include<> directives you need at the
(struct sockaddr *) &echoClntAddr, sizeof(echoClntAddr); } top of your source code – The type of each argument } /* end of main () */ – The possible return values Error handling is must
– The possible errors (in errno)