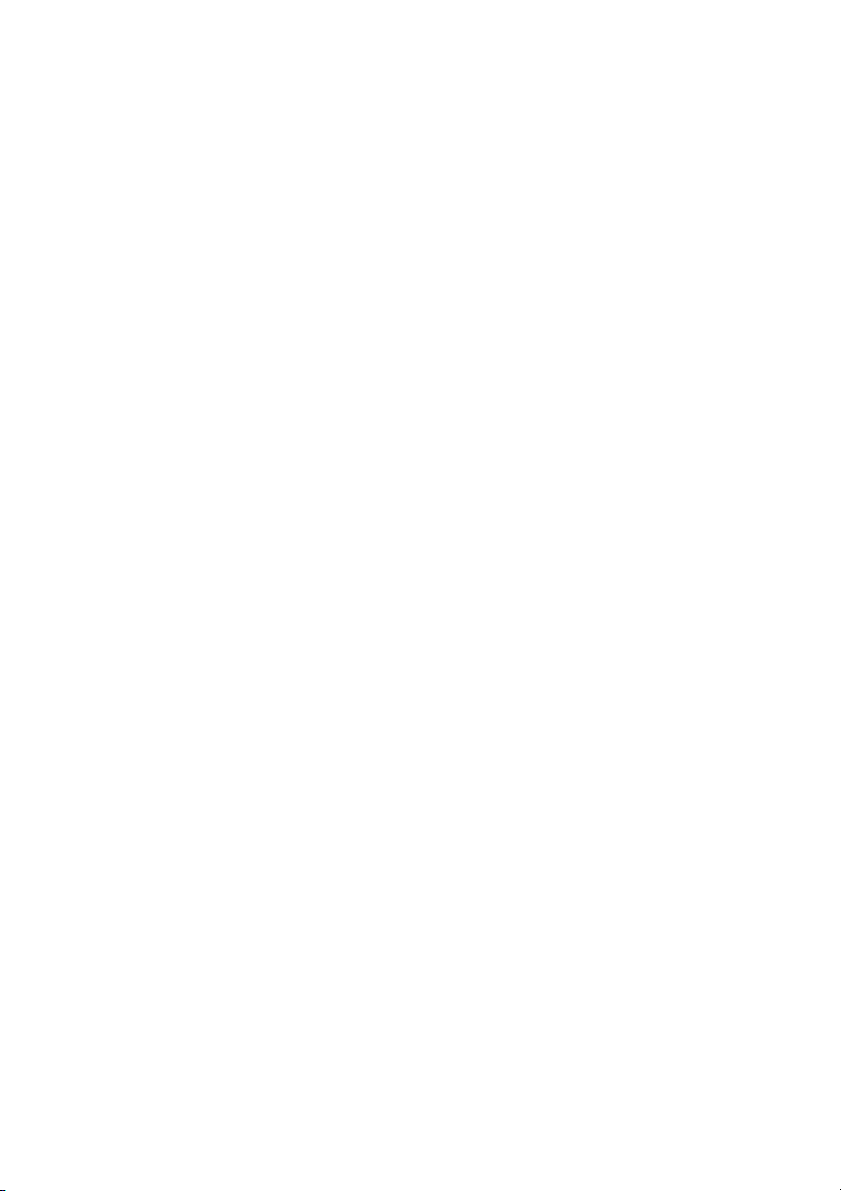
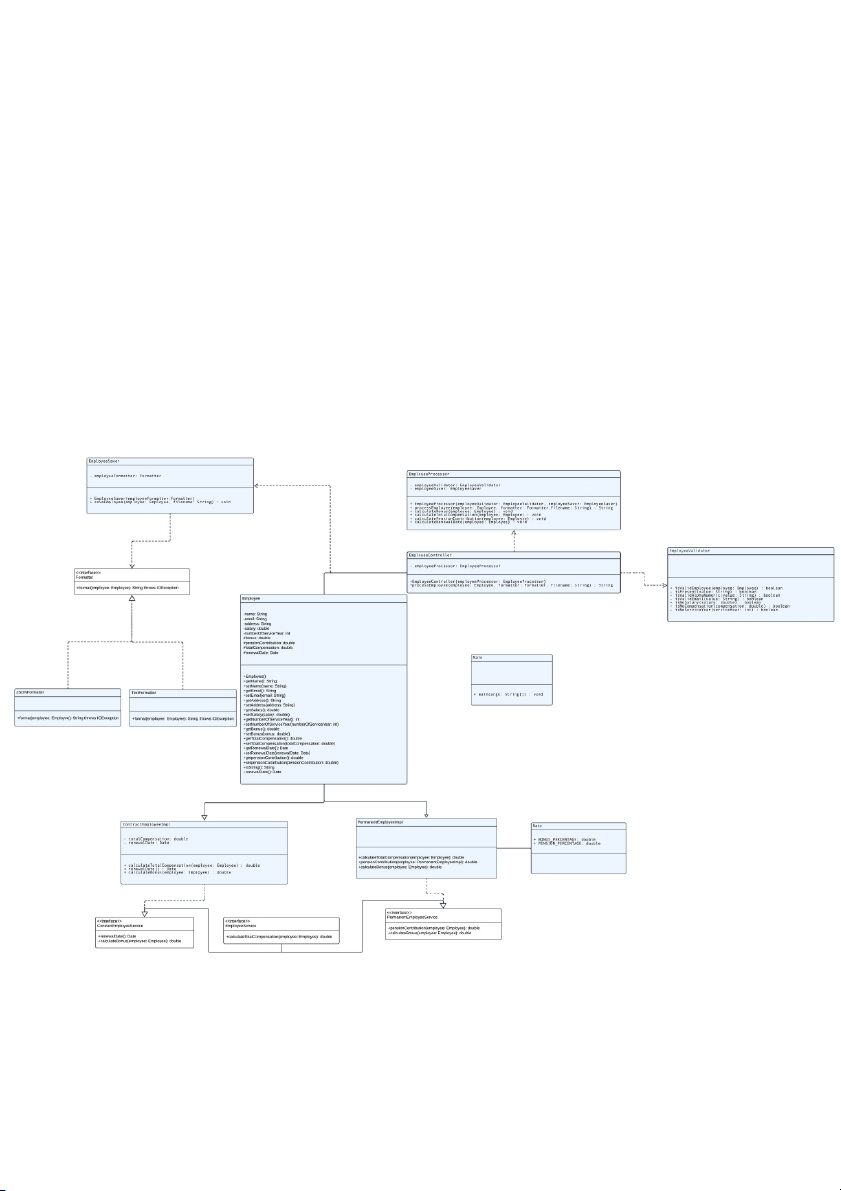
Preview text:
1. In the context of our EmployeeController class, it was initially handling multiple responsibilities such as
managing employee data and validating employee information. This was a violation of the SRP.
To rectify this, I introduced two new classes:
EmployeeSaver: This class is responsible for managing the employee data. It handles the storage,
retrieval, and manipulation of Employee objects. By separating these responsibilities into a dedicated
class, we ensure that changes to how employee data is managed will only affect this class.
EmployeeValidation: This class handles all the validation logic for Employee objects. It checks the
integrity and correctness of the employee data before it’s stored by the EmployeeStore class. By isolating
the validation logic in its own class, we can change or extend our validation rules without affecting other parts of our program.
By creating these two classes, we’ve ensured that each class has a single responsibility, thus adhering to
the SRP. This makes our code more maintainable, understandable, and flexible for future changes.
2. I also implemented the Interface Segregation Principle (ISP) to the EmployeeService interface to make
our code more flexible and adaptable to changes.
In the context of our EmployeeService interface, it initially had methods that were applicable to both
ContractEmployee and PermanentEmployee. However, not all methods were relevant to both types of
employees. This was a violation of the ISP.
To rectify this, I introduced three interfaces:
EmployeeService: This is a general interface that contains methods applicable to all employees, regardless of their type.
ContractEmployeeService: This interface extends EmployeeService and adds additional methods that
are specific to contract employees. Any class implementing this interface is a contract employee and
should implement these methods.
PermanentEmployeeService: This interface also extends EmployeeService and adds methods that are
specific to permanent employees. Any class implementing this interface is a permanent employee and
should implement these methods.
By creating these three interfaces, we ensure that a class implementing ContractEmployeeService
doesn’t need to know about the methods specific to PermanentEmployeeService, and vice versa. This
makes our code adhere to the ISP, making it more maintainable and flexible for future changes.
3. I created the ContractEmployeeImpl and PermanentEmployeeImpl classes to adhere to the Liskov
Substitution Principle (LSP) and the Open-Closed Principle (OCP), which are part of the SOLID principles
in object-oriented programming.The ContractEmployeeImpl and PermanentEmployeeImpl classes both
extend the Employee class, meaning they inherit all the properties and methods of the Employee class.
The ContractEmployeeImpl class implements the EmployeeService and ContractEmployeeService
interfaces, while the PermanentEmployeeImpl class implements the EmployeeService and
PermanentEmployeeService interfaces. This means that both classes have to provide implementations
for the methods declared in these interfaces.
By doing this, we ensure that each type of employee has its own class with methods that are specific to
that type of employee. This makes our code more organized and easier to understand. It also makes it
easier to add new types of employees in the future, as we can simply create a new class for each new
type of employee and implement the appropriate interfaces.
4. Lastly, I implemented the Formatter interface in the TextFormatter and JSONFormatter classes. This
required me to define the format method in TextFormatter and JSONFormatter, which takes an Employee
object as input and returns a String.
In the format method, I accessed the name and email properties of the Employee object and
concatenated them into a String with the format “name=xxxx, email=xyz@abc.com”.
By doing this, I was able to successfully save the Employee object as key-value pairs. This approach
adheres to the principles of clean and organized code, making it easier for me to understand and maintain.