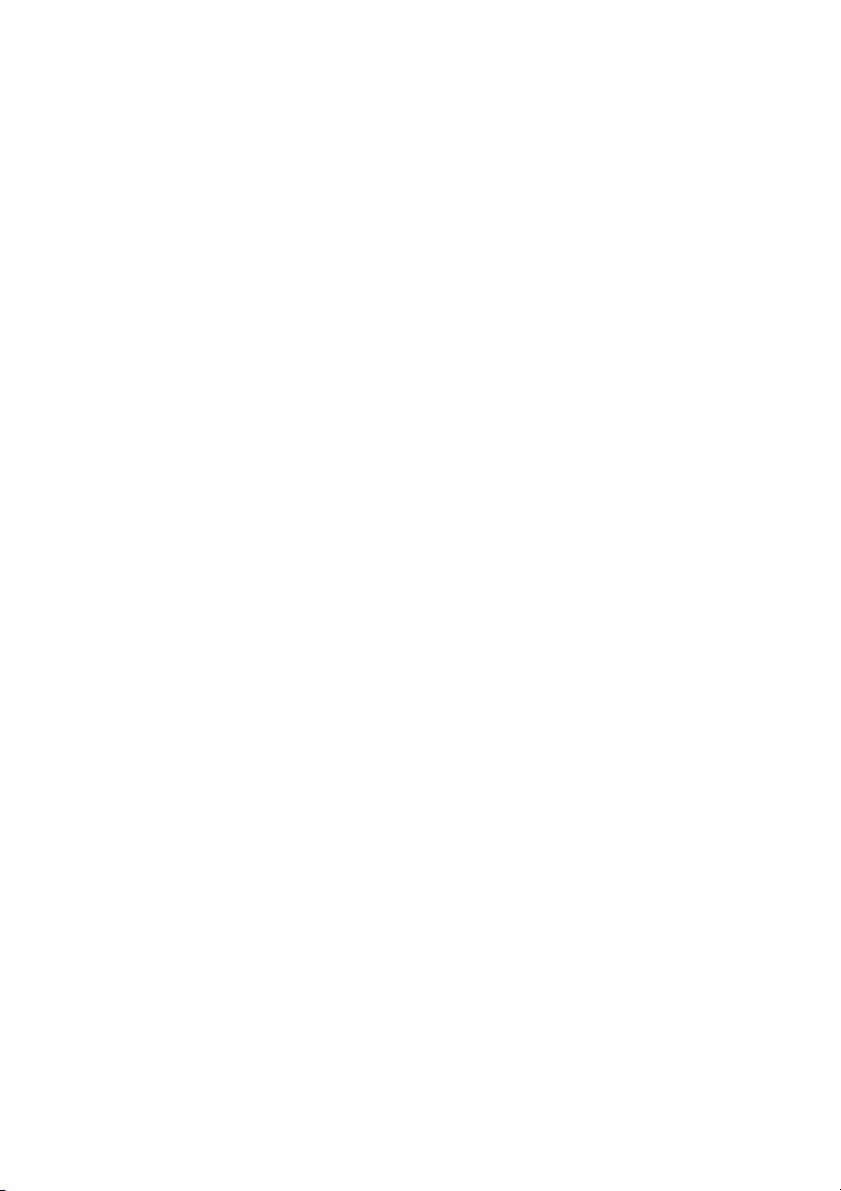
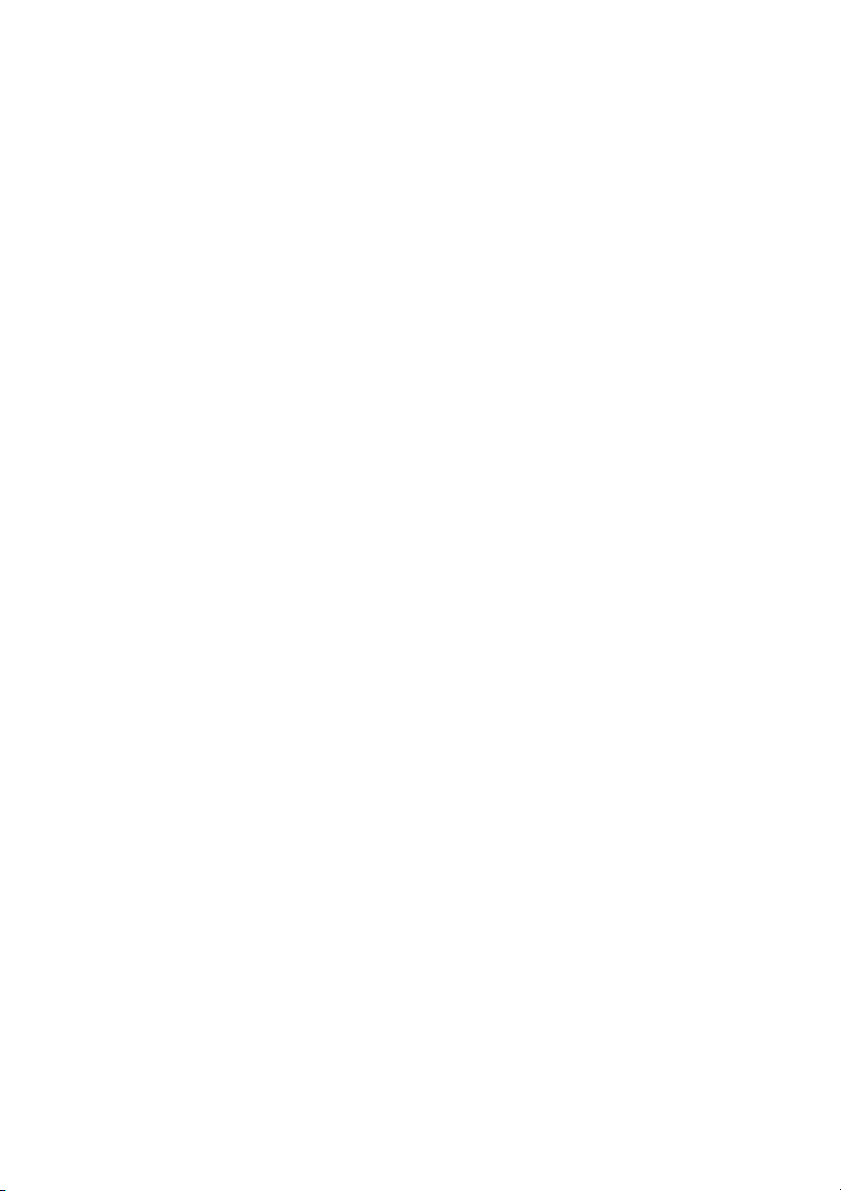
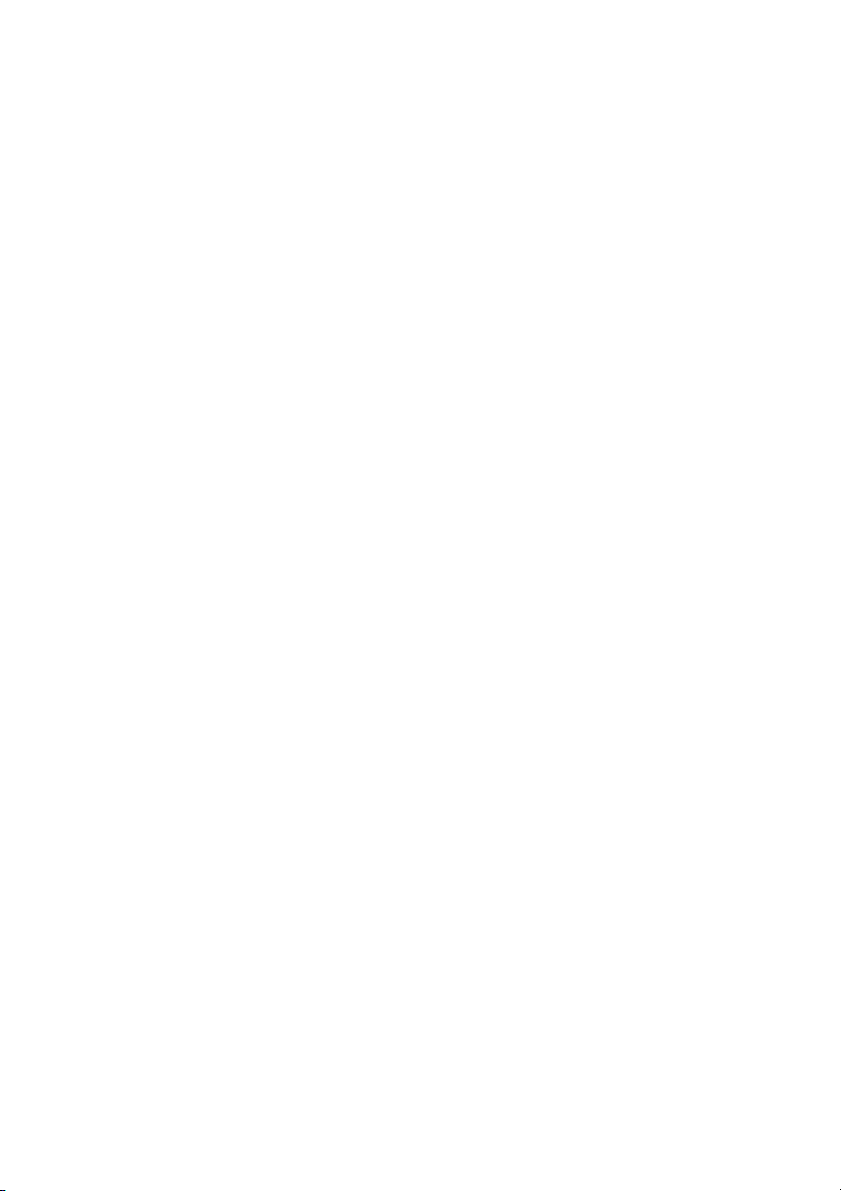
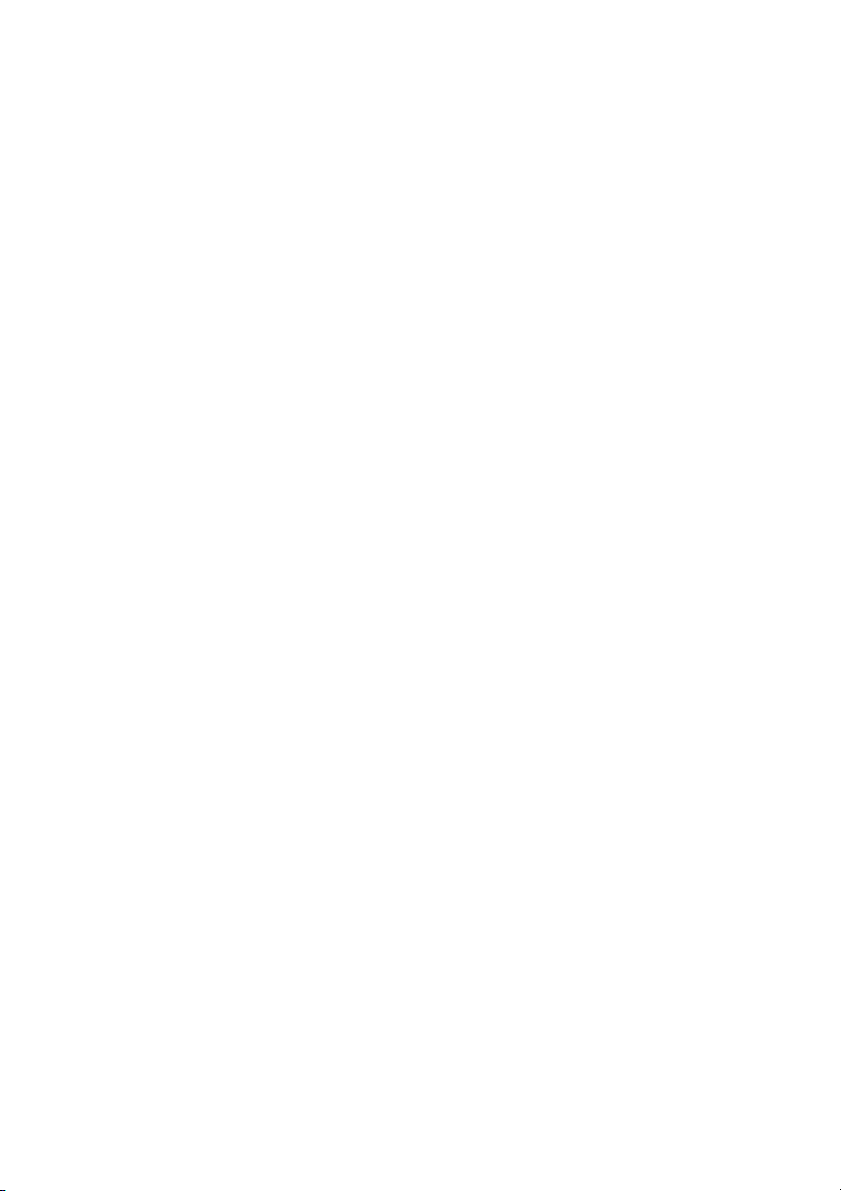
Preview text:
# Permutation import itertools E = {'a', 'b', 'c', 'd'} k = 3
# Print all the k-permutations of E n = len(E)
permute_k = list(itertools.permutations(E, k))
print("%i-permutations of %s: " %(k,E)) for i in permute_k: print(i)
print("The number of permutation = ", "%i!/(%i-%i)! = " %(n,n,k), len(permute_k)) # Combination import itertools E = {'a', 'b', 'c', 'd'} k = 3
# Print all the k-combinations of E
choose_k = list(itertools.combinations(E,k))
print("%i-combinations of %s: " %(k,E) ) for i in choose_k: print(i)
print("Number of combinations = %i!/(%i!(%i-%i)!) = %i" %(n,k,n,k,len(choose_k))) # Urn problems def cross(A, B):
# The set of ways of concatenating one item from collection A with one from B.
return {a + b for a in A for b in B}
urn = cross('W', '12345678') | cross('B', '123456') | cross('R', '123456789') print(urn) import itertools
U6 = list(itertools.combinations(urn, 6)) #Solution for (a): print(len(U6)) #Solution for (b): for s in U6: print(s) #Solution for (c): for s in U6:
if s[0][0]=='R' and s[-1][0]=='R': print(s) # exercise 1:
# A = {1, 2, 3, 4, 5}. Find all of 3-digit numbers can be formed from the
digits in set A without repetitions. Assign list of the numbers to
variable num_3_digit, and number of the numbers to variable num_length. import itertools A = {1, 2, 3, 4, 5} num_3_digit = 3 num_length = len(A)
permute_num_3_digit = list(itertools.permutations(A, num_3_digit))
print("%i-permutations of %s: "%(num_3_digit,A)) for i in permute_num_3_digit: print(i) # exercise 2: import itertools def cross(A, B):
return {a+b for a in A for b in B}
urn = cross('W', '12345678') | cross('B', '123456') | cross('R', '123456789') print(urn) # question a:
U3 = list(itertools.combinations(urn, 3)) len_U3 = len(U3) for s in U3: print(s) print('length U3 = ',len_U3) # question b: for s in U3:
if (s[0][0] != s[1][0] != s[2][0]): print(s) # question c: for s in U3:
if s[0][0] == 'W' and s[1][0] == 'R': print(s) # question 3: def cross (A, B):
return {a+b for a in A for b in B}
urn = cross ('M', '1234') |cross ('P', '123') | cross ('C', '12') |cross ('E', '1')
M = list(itertools.permutations(urn, 10)) k = 0 for i in M: count = 0 #for k in range(0, 10): if (i[0][0]!=i[1][0]): count= count + 1 if (i[1][0]!=i[2][0]): count= count + 1 if (i[2][0]!=i[3][0]): count= count + 1 if (i[3][0]!=i[4][0]): count= count + 1 if (i[4][0]!=i[5][0]): count= count + 1 if (i[5][0]!=i[6][0]): count= count + 1 if (i[6][0]!=i[7][0]): count= count + 1 if (i[7][0]!=i[8][0]): count= count + 1 if (i[8][0]!=i[9][0]): count= count + 1 #count = count + 1 #print(count) #print(count) if (count == 3): print(i) k = k + 1 print(k) # question 4: def cross(A, B):
return {a + b for a in A for b in B}
urn = cross('M', '123456') | cross('W', '123456789')
U5 = list(itertools.combinations(urn, 5)) k = 0 for s in U5: count = 0 if s[0][0] == 'W': count = count + 1 if s[1][0] == 'W': count = count + 1 if s[2][0] == 'W': count = count + 1 if s[3][0] == 'W': count = count + 1 if s[4][0] == 'W': count = count + 1 if(count == 2): print(s) k = k+1 print(k) # question 5: #import itertools #def cross (A, B):
# return {a + b for a in A for b in B}
#urn = cross('123456789JQK', '♠♣♦♥')
#U5 = list(itertools.combinations(urn, 5)) #for i in U5: # print(i) import itertools from itertools import product def cross(A, B):
return {a+b for a in A for b in B}
A = (1, 2, 3, 4 ,5 ,6 , 7, 8, 9, 10, 'J', 'Q', 'K')
B = ('♠', '♣', '♦' ,'♥') Card = list(product(A, B))
U5 = list(itertools.combinations(Card, 5)) #for i in U5: # print(i) len_poker_5 = len(U5) print(len_poker_5) len_three_of_a_kind = 0 for i in U5: count = 0 if i[0][0] == i[1][0]: count = count + 1 if i[1][0] == i[2][0]: count = count + 1 if i[2][0] == [3][0]: count = count + 1 if i[3][0] == i [4][0]: count = count + 1 if count == 3:
len_three_of_a_kind = len_three_of_a_kind + 1 print(len_three_of_a_kind)